Frame Animation Counter.
Author |
Message |
Unisyst
|
Posted: Thu Sep 14, 2006 10:20 pm Post subject: Frame Animation Counter. |
|
|
Animating sprites can be a pain, especially if you don't know where to start. Here's a little snippit of code to help you on your way.
This will create a counter variable called worldtick, which changes frames quite nicely. The image file is stored in an array called picID, each image is automatically added depending on how many frames there are.
All you have to do is enter how many frames you have (framecount) and have the files ready to be read.
code: |
var iWindow : int := Window.Open ("graphics:320;240, offscreenonly") %open up a new window (not necessary) you can open your window how you want, I just like doing this because you can close it using Window.Close (iWindow)
var worldtick, framecount, speed : int
framecount := 8
speed := 3 %lower the number, the faster it is
/*okay this might be a bit confusing here this will create an array
picID which holds each frame in onevariable, you must make it start on
0 because of the variable that displays the pictures starts at 0*/
var picID : array 0 .. framecount - 1 of int
for p : 0 .. framecount - 1
picID(p) := Pic.FileNew ("frame" + intstr (p) + ".bmp") %inserts the image into each picID() keeps code tidy
end for
worldtick := 1 %first tick starts now
loop
put "Current frame: ", worldtick div speed mod framecount %div = framerate, mod = number of frames
Pic.Draw (picID(worldtick div speed mod framecount), 2, 2, picCopy)
worldtick += 1 %For every loop, there is a tick
/*[1] overflow barrier the world tick constantly ticks
if this number gets to high, turing will throw up an error*/
if worldtick = maxint - 1 then
worldtick := 1
end if %%[/1]
/*[2] Usually in anything relating to graphics needs to be
updated properly on the screen */
View.Update
delay (10)
cls %%[/2]
end loop
|
Note to mod, move this into the Turing Source Code section of the forum if you so please.
Description: |
|
 Download |
Filename: |
frametut.rar |
Filesize: |
9.47 KB |
Downloaded: |
72 Time(s) |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
NikG
|
Posted: Sat Sep 16, 2006 12:39 am Post subject: (No subject) |
|
|
Not bad, but let's say you wanted to do this for multiple sprites each with its own delay. You COULD create separate counter variables for all of them, but that's not efficient and it's not flexible.
Instead, implementing your code within classes easily allows you to customize each "sprite" from the pic it's using to the delay before the next one.
|
|
|
|
|
 |
Tony
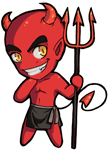
|
Posted: Sat Sep 16, 2006 2:28 pm Post subject: (No subject) |
|
|
delays in multiple objects is a bad idea.. it will just halt the entire program, unless it's in a process. Which is also a bad idea.
I suggest measuring time change between ticks, and simply do nothing until enough time has elapsed
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Unisyst
|
Posted: Sat Sep 16, 2006 4:16 pm Post subject: (No subject) |
|
|
Actually all you have to do is specify in, Pic.Draw (picID(worldtick div speed mod framecount), 2, 2, picCopy) instead of using speed and framecount you can specify your own.
|
|
|
|
|
 |
Unisyst
|
Posted: Sat Sep 16, 2006 4:17 pm Post subject: (No subject) |
|
|
Tony wrote: delays in multiple objects is a bad idea.. it will just halt the entire program, unless it's in a process. Which is also a bad idea.
I suggest measuring time change between ticks, and simply do nothing until enough time has elapsed
Aye that's exactly what my code does.
|
|
|
|
|
 |
NikG
|
Posted: Sun Sep 17, 2006 1:45 am Post subject: (No subject) |
|
|
Tony wrote: delays in multiple objects is a bad idea.. it will just halt the entire program, unless it's in a process. Which is also a bad idea.
I suggest measuring time change between ticks, and simply do nothing until enough time has elapsed Sorry for not being clear. I was not refering to Turing's delay command. I was just talkin about the general sprite animation delay/interval (i.e. one sprite updating every frame whereas another updating every two frames). This can be done easily in classes (as shown in my rts game where the radar and hq update at different intervals).
Unisyst wrote: Actually all you have to do is specify in, Pic.Draw (picID(worldtick div speed mod framecount), 2, 2, picCopy) instead of using speed and framecount you can specify your own.  Unisyst, what if you had 9 worldticks (worldtick1, worldtick2... worldtick9) and each one had a different delay? Again, you COULD create that statment 9 times (Pic.Draw(worldtick1 div speed1 mod framcount1...) or you could create a class with the name of the pic and the interval amount.
|
|
|
|
|
 |
|
|