Flying Through Space?
Author |
Message |
Slaivis
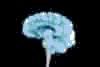
|
Posted: Mon Jul 03, 2006 11:15 pm Post subject: Flying Through Space? |
|
|
Alright, so I'm making a game, and here is the background for it.
The intended effect is that you're flying through space at >= light speeds.
Here is the code:
code: | setscreen ("graphics:800;600,offscreenonly,nobuttonbar")
var lc : int %Create Light?
var lmax : int := 100 %Light Maximum - maximum number of 'particles'
var le : array 1 .. lmax of boolean %The status of each 'slot' or potential particle.
var lang, ldc, ldci : array 1 .. lmax of int %particle angle, particle distance from centre, particle initial distance from centre
for i : 1 .. lmax %Initializing values
le (i) := false
ldci (i) := 0
ldc (i) := 0
end for
%Pre-calculate reccuring values so that the animation is faster
var sina, cosa : array 1 .. 360, 1 .. 100 of int
for i : 1 .. 360
for j : 1 .. 100
sina (i, j) := round (sind (i) * j)
cosa (i, j) := round (cosd (i) * j)
end for
end for
%Pre-calculate reccuring values so that the animation is faster
var lx, ly : array 1 .. 360, 1 .. (maxx * 2) of int
for i : 1 .. 360
for j : 1 .. (maxx * 2)
lx (i, j) := round (sind (i) * j)
ly (i, j) := round (cosd (i) * j)
end for
end for
%Pre-declare procedures so that their order of declaration does not matter.
forward proc createlight (n : int)
forward proc createlightQ
forward proc light (n : int)
%Procedure to re-set the values of a new particle
body proc createlight (n : int)
le (n) := true
randint (lang (n), 1, 360)
ldc (n) := 1
ldci (n) := 0
lx (lang (n), 1) := 0
ly (lang (n), 1) := 0
end createlight
%Procedure to decide whether a new particle is created or not
body proc createlightQ
randint (lc, 1, 100)
if lc > 75 then
for i : 1 .. lmax
if le (i) = false then
createlight (i)
exit
end if
end for
end if
end createlightQ
%Draw a given particle
body proc light (n : int)
if ldci (n) <= 98 then
drawline ((lx (lang (n), 1) + (maxx div 2)), (ly (lang (n), 1) + (maxy div 2)), (lx (lang (n), 1) + sina (lang (n), ldci (n)) + (maxx div 2)),
(ly (lang (n), 1) + cosa (lang (n), ldci (n)) + (maxy div 2)), 0)
else
drawline ((lx (lang (n), ldc (n)) + (maxx div 2)), (ly (lang (n), ldc (n)) + (maxy div 2)), (lx (lang (n), ldc (n)) + sina (lang (n), ldci (n)) + (maxx div 2)),
(ly (lang (n), ldc (n)) + cosa (lang (n), ldci (n)) + (maxy div 2)), 0)
end if
if ldc (n) >= round(maxx * 1.8) then
le (n) := false
end if
end light
%Main loop
drawfillbox (0, 0, maxx, maxy, 7)
loop
drawfillbox (0, 0, maxx, maxy, 7)
createlightQ
for i : 1 .. lmax
if ldci (i) < 97 then
ldci (i) += 4
elsif ldci (i) > 97 then
ldc (i) += 4
end if
if le (i) = true then
light (i)
end if
end for
View.Update
end loop
|
If anybody has some suggestions on how to either improve it, or even just general comments on if the effect is achieved or not, then I'll be happy to listen to them. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Cervantes

|
Posted: Tue Jul 04, 2006 7:44 am Post subject: (No subject) |
|
|
It takes a long time to initialize all those values.
I ran some benchmarks and discovered that your program takes 51996 ms to execute that main loop 10000 times, on my computer. If you use a version of your program that doesn't precalculate all those sin and cos values, it takes 60300 ms to execute that main loop 10000 times. Not too much of a difference, especially considering that you'd want some sort of frame rate control in there to slow it down even more.
But the slower version is faster, because it doesn't have to spend a long time at the startup (when the user doesn't know what's going on) calculating a table of values.
Also, realize that by calculating this table of values for only integers, you're losing accuracy. Now, it doesn't matter to us, the user, because we don't notice it. Just something to keep in mind. Rounding too early is never good (though often it's not that bad). |
|
|
|
|
 |
Slaivis
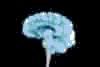
|
Posted: Tue Jul 04, 2006 12:10 pm Post subject: (No subject) |
|
|
Cervantes wrote: It takes a long time to initialize all those values.
I ran some benchmarks and discovered that your program takes 51996 ms to execute that main loop 10000 times, on my computer. If you use a version of your program that doesn't precalculate all those sin and cos values, it takes 60300 ms to execute that main loop 10000 times. Not too much of a difference, especially considering that you'd want some sort of frame rate control in there to slow it down even more.
But the slower version is faster, because it doesn't have to spend a long time at the startup (when the user doesn't know what's going on) calculating a table of values.
Also, realize that by calculating this table of values for only integers, you're losing accuracy. Now, it doesn't matter to us, the user, because we don't notice it. Just something to keep in mind. Rounding too early is never good (though often it's not that bad).
I don't know what you mean by all the stuff about the slower version is faster...
What I originally did with this program was inserted the sin/cos calculations right into the drawline command, and that worked waay too slowly, also, all those calculations are redundant simply because you're using the same values over and over again but re-calculating them each time.
As for the long initialization time, that doesn't really matter to me. It's only a few seconds, but keep in mind that this is not the final product, it is simply a portion of my program, and I plan to insert a 'loading' screen to inform the user of what is actually happening. Besides, it's not like they won't spend some time looking at the menu anyway, right?
As for calculating the table of values for only integers, well.... I sort of have to... You can't use the draw-commands with real values.
- Thanks for the advice |
|
|
|
|
 |
Cervantes

|
Posted: Tue Jul 04, 2006 4:23 pm Post subject: (No subject) |
|
|
Slaivis wrote: I don't know what you mean by all the stuff about the slower version is faster...
I mean that the slower version doesn't have to go through that 10 second initialization time. That is a good thing.
Slavis wrote:
As for calculating the table of values for only integers, well.... I sort of have to... You can't use the draw-commands with real values.
Yes, you have to use integers to draw your lines. However, you have to ask yourself when you want to round your real numbers to integers. It's generally best to round them right before you draw them.
code: |
Draw.Line (round (...), round (...), round (...), round (...), LineColour)
|
|
|
|
|
|
 |
Slaivis
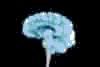
|
Posted: Tue Jul 04, 2006 4:45 pm Post subject: (No subject) |
|
|
Ah... I see what you mean. I'll have to go through my code again and see if it makes a difference, because there are many variables for even one parameter, but I think that most of them are integers anyway. My goal was to minimize the calculations that have to be doen right before drawing, but I'll see.
Although the slower version doesn't have to go through the initialization time, the slower version is also unbearably slow - it was the first way I did it and it wouldn't work.
I mean, that's the background alone, and re-calculating the value each time only slows things down. It is also redundant (as I've said before).
So, I'd rather have even a whole minute of initialization than to have a slow animation... A slow animation, in my opinion, would defeat the effect in this case.
However, I would still like to thank you for your advice. |
|
|
|
|
 |
Windsurfer

|
Posted: Tue Jul 04, 2006 4:59 pm Post subject: (No subject) |
|
|
Cool... nice line effect...
I agree with the comments above about pre-calculating. it doesn't seem worth it...
But my biggest issue is with the fact that the lines are always moving at the same speed on the screen. At the edge, where one would expect the lines to be closer and therefore faster, they move at the same speed.
But other than that, yeah, i can see how Quote: It's sort of the same effect... But it's very different, and it's done differently.  |
|
|
|
|
 |
Slaivis
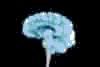
|
Posted: Tue Jul 04, 2006 9:26 pm Post subject: (No subject) |
|
|
Windsurfer wrote: Cool... nice line effect...
I agree with the comments above about pre-calculating. it doesn't seem worth it...
But my biggest issue is with the fact that the lines are always moving at the same speed on the screen. At the edge, where one would expect the lines to be closer and therefore faster, they move at the same speed.
But other than that, yeah, i can see how Quote: It's sort of the same effect... But it's very different, and it's done differently. 
Heh, thanks for the comment.
Perhaps to you the precalculating doesn't seem worth it, but to me, after I tried the 'caclulate as I go' method... Well... It was unacceptable... I had set the incremets of movement to 10, and the lines still moved at a snail's pace. It simply wasn't what I wanted.
Again, thank's for the advice.
I'll look into increasing the speed as the lines get closer to the edge of the screen... I really didn't think about that effect, but thanks for pointing it out. |
|
|
|
|
 |
xtremehacker
|
Posted: Thu Sep 07, 2006 6:11 pm Post subject: (No subject) |
|
|
Good work man it would be better if the lines went faster as they left the page but well done, good start. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Clayton

|
Posted: Fri Sep 08, 2006 2:40 pm Post subject: (No subject) |
|
|
well i can see your new to compsci so ill let you know that we dont support necromancers here (ie. reviving old or dead topics), generally a good rule of thumb, if its been more than three weeks since the last post in the topic, dont post, unless you have something really important to add, plz take a gander at [The Rules] to make sure you dont break any more. |
|
|
|
|
 |
|
|