Author |
Message |
aldreneo
|
Posted: Fri May 05, 2006 7:50 pm Post subject: My C code is acting strange |
|
|
What I want the code to output(red is stuff I typed blue is stuff the computer outputted):
>test
test
>test with spaces
test with spaces
>
code: |
#include<stdio.h>
main( )
{
int loop;
while(loop>=0)
{
char i;
printf( ">" );
scanf( "%s", &i );
printf( "%s \n", &i );
}
}
|
But this is what I get as an output (Stuff in red is what I typed stuff in blue is what the computer outputted):
>test
test
>test with spaces
test
>with
>spaces
>
Gcc latest |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
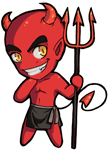
|
|
|
|
 |
aldreneo
|
Posted: Fri May 05, 2006 8:08 pm Post subject: (No subject) |
|
|
Not much....more used to c++ why? is it something stupid? |
|
|
|
|
 |
Tony
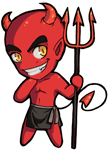
|
Posted: Fri May 05, 2006 8:24 pm Post subject: (No subject) |
|
|
I don't think you know C++ well ether..
#include<stdio.h>
main( )
{
int loop; <- uninitialized variable, no assigned value
while(loop>=0) <- comparing random memory location against 0.
// how about while(true) for an infinite loop?
{
char i; <- declaring a character
printf( ">" );
scanf( "%s", &i ); <- reading a character string into character
printf( "%s \n", &i );
}
}
%s ends by whitespace (space, newline, etc)
you're probably looking for gets() |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
aldreneo
|
Posted: Fri May 05, 2006 8:34 pm Post subject: (No subject) |
|
|
That was stupid of me...
Updated source works...
code: |
#include<stdio.h>
main( )
{
int loop;
while(loop>=0)
{
char i;
printf( ">" );
gets( &i );
printf( "%s \n",&i );
}
}
| [/code] |
|
|
|
|
 |
rizzix
|
Posted: Fri May 05, 2006 8:49 pm Post subject: (No subject) |
|
|
That code is not safe. |
|
|
|
|
 |
aldreneo
|
Posted: Fri May 05, 2006 8:53 pm Post subject: (No subject) |
|
|
How? |
|
|
|
|
 |
Tony
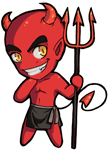
|
Posted: Fri May 05, 2006 9:02 pm Post subject: (No subject) |
|
|
let me try to explain it to you again
Tony wrote:
char i; <- declaring a character
scanf( "%s", &i ); <- reading a character string into character
Now you're reading an entire line.
Guess what - a line is much bigger than a character, so
- you locate 2 empty bytes of space in your memory
- you fill those 2 bytes, and up to 254 bytes after (is there a limit on gets?). All but the first 2 bytes are not neccessarely free, so you end up writing over abstract memory location.
Oh, and it's an infinite loop, so you're doing this over and over and over again. Do it enough, and watch your system start acting up funny and die. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
md

|
Posted: Fri May 05, 2006 9:35 pm Post subject: (No subject) |
|
|
You are still using an unitialized int for running through your loop (int loop); nad your reading a string in and and trying to store it in a character. I would sugest reading some more about C (and C++ for that matter), as these are serious errors. |
|
|
|
|
 |
Martin

|
Posted: Fri May 05, 2006 11:53 pm Post subject: (No subject) |
|
|
Guys, can we be a little less hostile and a little more friendly please? |
|
|
|
|
 |
md

|
Posted: Sat May 06, 2006 1:05 pm Post subject: (No subject) |
|
|
Ok, here is your Code
C: |
#include<stdio.h>
main( )
{
int loop;
while(loop>=0)
{
char i;
printf( ">" );
gets( &i );
printf( "%s \n",&i );
}
}
|
Here is one possible way of writing it would be (that is proper)
C: |
#include<stdio.h>
main( )
{
int loop = 0;
while(loop>=0)
{
char buffer[256];
printf( ">" );
gets( &buffer );
printf( "%s \n",&buffer );
}
}
|
Notice how the variable loop is initialized to be 0 before it's used? If you don't initialize a varaible then it's value is undefined (it could be anything). Also note that buffer is an array of characters. In C char is a single character, whereas strings are stored as a null-terminated array of characters. |
|
|
|
|
 |
rizzix
|
Posted: Sat May 06, 2006 3:14 pm Post subject: (No subject) |
|
|
That too, is not safe. What happens if the user enters 256 or more characters?
Simply put: gets is unsafe by design. Don't use it in this manner (i.e. to get strings). |
|
|
|
|
 |
|