Author |
Message |
cool dude

|
Posted: Sun Apr 30, 2006 1:46 pm Post subject: input |
|
|
i'm just starting to learn Java alone. i checked out wtd tutorial which was very helpful i just don't know how to get user input so can somebody show me a very simple example |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
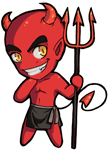
|
|
|
|
 |
cool dude

|
Posted: Sun Apr 30, 2006 3:36 pm Post subject: (No subject) |
|
|
i don't think u understood my question correctly. i want to know how to get input from the user. NOT from a file. just like in turing u say get so how do u do that in java. i'm just starting out i have no clue |
|
|
|
|
 |
Tony
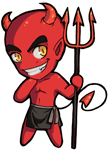
|
Posted: Sun Apr 30, 2006 4:26 pm Post subject: (No subject) |
|
|
I'm pretty sure that the code provided in the tutorial is for reading the keyboard input..  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
cool dude

|
Posted: Sun Apr 30, 2006 5:48 pm Post subject: (No subject) |
|
|
k u might be right because i'm just starting to learn java but i really can't understand it. what is the import java.io mean? and is it something i just need to memorize? also how come they are talking about files if u keep looking at the rest of the posts below if u said thats input from user. wat i mean is like what is your name? and then the user can answer and then it can say hello user. for some reason even using that code i can't do such a simple program. if i copy and paste that code it says identifier expected, and i have no clue wat that means since i just started learning java on my own. |
|
|
|
|
 |
Tony
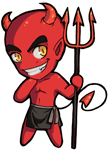
|
Posted: Sun Apr 30, 2006 6:21 pm Post subject: (No subject) |
|
|
java.io is the I/O class - Input/Output, you import it into the program to be able to use its functionality
the basic program would look something like
I think... I hope someone can correct me on this if needed. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
wtd
|
Posted: Sun Apr 30, 2006 6:46 pm Post subject: (No subject) |
|
|
java.io is a package which includes classes used in I/O operations. |
|
|
|
|
 |
cool dude

|
Posted: Sun Apr 30, 2006 7:27 pm Post subject: (No subject) |
|
|
wow thanks that helped!
the only things i don't get is firstly what is try and catch for and what does this line mean?
code: |
in = new BufferedReader(new InputStreamReader(System.in));
out = System.out;
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Krabjuice
|
Posted: Sun Apr 30, 2006 7:49 pm Post subject: (No subject) |
|
|
Are you using Java 1.5?
How about using the Scanner construct? Its pretty quick and easy. |
|
|
|
|
 |
Tony
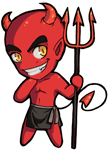
|
Posted: Sun Apr 30, 2006 8:31 pm Post subject: (No subject) |
|
|
wtd wrote: java.io is a package
you're absolutely right, thx
cool dude wrote: what is try and catch for
if there's any error inside the try block, catch will be executed instead. Since reading input is prone to screwups (wrong input? eof? etc) it is usually a good idea to place it within try/catch
the code declears the buffer you're reading from and outputting to.
Actually out is just an alias for System.out
so out.println is same as System.out.println |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
[Gandalf]

|
Posted: Sun Apr 30, 2006 9:01 pm Post subject: (No subject) |
|
|
Much simpler using the Scanner class:
Java: | import java.util.*;
public class UserInput {
public static void main (String[] args ) {
Scanner in = new Scanner (System. in);
String userName;
System. out. println("Hello, enter your name: ");
userName = in. nextLine();
System. out. println("Your name is " + userName + "!");
}
} |
Hope I didn't make any stupid/obvious mistakes, don't have Java SDK installed here. |
|
|
|
|
 |
Tony
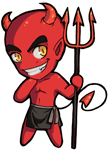
|
Posted: Sun Apr 30, 2006 9:05 pm Post subject: (No subject) |
|
|
the only difference is the declaration of the input buffer to read from really..
I think it should still be inside try/catch setup for the sake of good programming practice.
the bottom line is that when attempting to execute you're going to recieve an error message from the catch block and not have an exception escalate and crash the entire application. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
wtd
|
Posted: Sun Apr 30, 2006 9:51 pm Post subject: (No subject) |
|
|
No... good programming practice is not to dismiss errors unless you have something meaningful to do. |
|
|
|
|
 |
MysticVegeta

|
Posted: Mon May 01, 2006 1:45 pm Post subject: (No subject) |
|
|
Tony wrote: java.io is the I/O class - Input/Output, you import it into the program to be able to use its functionality
the basic program would look something like
I think... I hope someone can correct me on this if needed.
I think you can just do this to reduce the try and catch statements:
Notice the "throws IOException" reduces your lines, but one disadvantage is that you cant output any message "you" would like when the try fails |
|
|
|
|
 |
cool dude

|
Posted: Mon May 01, 2006 4:34 pm Post subject: (No subject) |
|
|
k i got this working with any input that is a string but how can i get it to work with integers? |
|
|
|
|
 |
|