The Dir.Module
Author |
Message |
jamonathin

|
Posted: Fri Mar 24, 2006 1:43 pm Post subject: The Dir.Module |
|
|
I cant really explain the Dir. Module definition better than Holtsoft did, so here it is.
F10 wrote: This unit contains the predefined subprograms that deal with directories. You can use these subprograms to list the contents of directories, create directories, change directories and return the current directory.
Part 1: Dir.Module
Getting Started . . .
Each Dir. Module that I explain includes a sample program, hopefully at the end of this tutorial you will be able to understand how to create a program using several of these commands.
Dir.Current : string
Dir.Current tells your program which Directory you are in. This command is very useful when writing an extensive program where you are constantly changing directories.
Dir.Current returns a string value and can be assigned to a variable, or displayed on the screen.
Turing: |
var directory : string
directory := Dir.Current
put directory |
Dir.Create (x : string)
Dir.Create creates a folder in the current Directory. After creating this directory, the user can now access this through turing and is able to write files and save them to this folder, as well as create more folders.
Dir.Change (x : string)
Dir.Change allows the user to change the current directory that files and folders are created in. This is useful for creating text files, saving pictures, and creating new folders, because whichever Directory you changed to, those files are saved there.
Dir.Delete (x : string)
Dir.Delete deletes a folder that is it assigned to. However, if that directory is not empty, it wont be deleted. Later on in the Tutorial I will explain how to clear the folder then Delete it.
Dir.Exists (x : string) : boolean
Dir.Exists is a simple boolean function. All it does is tell the user if the directory that the user entered exists or not, and is returned by a true or false boolean statement.
Here is a sample program that outputs false.
Now theoretically this should return true, correct? Wrong, but why? If 'x' represents the current Directory and we just created a new folder there, why would it say it doesn't exist? Best way to check, look at what Dir.Exists is actually reading.
Add this line to the end.
Turing: |
put x + "New Folder"
|
You'll notice that your directory and your "New Folder" are mushed together, this is because you're not telling the program that "New Folder" is a folder, and not an extension to the string Dir.Current.
Simple, but commonly forgotten.
Later on in the tutorial there is a section on how to properly use variables to represent folders, and using the slashes ("/", "\").
-------------------------------------------------------------------
The next three Dir.Methods that I'll be explaining all work together, and can't be used by oneself, or else it would serve no real purpose, (my opinion). So, each method will be explained individually, but only one example will be shown.
Dir.Open (x : string) : int
Dir.Open uses an integer variable to represent the directory's stream number, where a string value is inserted into the function. It is used like such:
Remember, Dir.Current returns a string value, so it can be inserted into this just like any string variable.
Dir.Close (x : int)
Dir.Close is fairly simple, it closes the directory that was opened by Dir.Open. After the directory is closed, it cannot be accessed by other Dir.Modules unless re-opened by Dir.Open.
Here's what it looks like:
Dir.Get (x : int) : string
Dir.Get now works with Dir.Open. It uses the streamNumber that Dir.Open used, and returns a string value to a variable. It is used in this context:
Ok great, a dot, like I really have a file named ".". That dot represents the current directory. Now if you were to add this to the end of the file, guess what, 2 dots! Where the second dot represents the parent directory
Turing: |
file := Dir.Get (streamNumber )
put file
|
But that's all for now, I promise. After that the files names will start to appear (assuming your current directory isn't empty)
Here's the big question, how do I get ALL of the file names? . . . . Loop it! Here's how you do that.
** Note, this program uses flexible arrays if unsure on how to use, refer to the Turing Walkthrough after **
Turing: |
% Just incase we have a lot of files :)
View.Set ("text")
var streamNumber : int
% Open the Current Directory
streamNumber := Dir.Open (Dir.Current)
% We use a flexible array because we don't know exactly how
% many files and folders we are lookin at.
var files : flexible array 1 .. 0 of string
% Loop it!
loop
% New File
new files, upper (files ) + 1
% Get that file
files (upper (files )) := Dir.Get (streamNumber )
% Show the file name
put files (upper (files ))
%Exit when there's no more
exit when files (upper (files )) = ""
end loop
Dir.Close (streamNumber )
|
:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
Part 2: File.Module
In Part 1, I said I was going to explain how to Delete a Directory that contains files. This Part of the tutorial I will be explaining parts of the File Module that help use some of the Dir.Module tools.
** However I wont be explaining everything in the File.Module because that alone can be a tutorial. **
File.Exists (x : string) : boolean
Starting off easy, File.Exists does exactly what Dir.Exists does, only instead of a directory, it checks for a file.
Here is a sample program that asks the user for a filename on their current Directory, and checks if it exists or not.
Turing: |
var file : string
put "Current Directory: ", Dir.Current
put skip
put "Enter a file name to see if it exists: " ..
get file
put skip
put "Does the file exits: ", File.Exists (file )
|
File.Delete (x : string)
File.Delete just deletes a file that it is told to delete, that is of course if it exists. Here's a sample program using File.Exists.
Turing: |
var file : string
put "Current Directory: ", Dir.Current
put skip
put "Enter a file name to delete it Permanately: " ..
get file
put skip
if File.Exists (file ) then %If File exists then delete
File.Delete (file )
put "File Deleted!"
else %Else, don't even try.
put "File does not exist."
end if
|
File.Rename (xStart, xEnd : string)
You can use this tool to rename a file. You first enter the original file name, then enter the name you would like to change it to - seperated by a comma. The good thing about this command is that it wont crash your program if the file does not exist. You can see if the change was successful or not by adding in a "Error.LastMsg" command.
** Note, if your error message reads "Unknown error #0", the change was successful. **
File.Copy (xStart, xEnd : string)
This command is used just how File.Rename is used. Only difference is we are copying the file to somewhere, rather than renaming it. Here's an example on how it can be used.
That is, assuming there's a folder named "My Documents" in your current Directory.
** Note again, if your error message reads "Unknown error #0", the copy was successful. **
:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
Part 3: Misc.
Proper use of slashes ["\\" or "/"]
Throughout the tutorial, strings like such:
Quote: "My Documents\\changer2.t"
Have been used.
Now what if we were to assign a variable to "My Documents" and "changer2.t", would we be able to still use "\\" ? Let's check.
Now at first it looks like something is messed up, since everything past the last quote is in red text - no worries, just hit F2 or run the program and everything will work right.
Paths in Windows use a forward slash ("\") between folders. However, our paths in our Turing code look like "C:\\Windows" because, as you may know, the backslash is a special character -- the "escape character". This means that it escapes the character that follows it to new meaning. For example, "\n" is the newline character; "\d" represents a digit. "\s" represents whitespace. Now, "\" is a special character, because it is the escape character. So to escape it, we need to put another "\" in front of it, to get this: "\\".
Alternatively, we can use a forward slash ("/") just as we use "\\". Turing recognizes this as the same. Just don't go typing "C:/Windows" in Windows Explorer.
Deleting a Directory
This program here is simply going to create a folder, makes some files in it, then delete all of the files and the directory created.
Turing: |
%Holding the first directory
var origDir : string := Dir.Current
% stream variable that creates files
var stream : int
Dir.Create ("New Dir") %Creating first directory
put "'New Dir' created in: ", Dir.Current
Dir.Change ("New Dir") %Change to new Directory
%Creating files in 'First Dir'.
for i : 1 .. 10 %Making 10 files
open : stream, intstr (i ) + ".txt", put
put : stream, i %Just puting a number in there
close : stream
put intstr (i ) + ".txt created!"
end for
% Deleting Part %
%%%%%%%%%%%%%%%%%
put "Now you can check to see if this worked or not, or press any key to delete"
Input.Pause
cls
% Represents the files that we are retrieving
var files : string
put "Retrieving files from: ", Dir.Current %Displaying where we are
var currentDir : int %This represents which directory we are in
currentDir := Dir.Open (Dir.Current)
loop
% Saving that file name
files := Dir.Get (currentDir )
% Exit when there are no more files
exit when files = ""
% Deleting that file
put "Deleting File: ", files
File.Delete (files )
end loop
Dir.Close (currentDir ) %Close the directory
% Change Directory
Dir.Change (origDir )
put "\nChanging Dir to: ", Dir.Current
%Finally delete the folder
Dir.Delete ("New Dir")
put "New Dir deleted."
|
That's it for this tutorial, if there's anything here you need me to explain- or if you need help incorporating any of these into a program of your own, feel free to ask .
- Thx Cervantes  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
person
|
Posted: Fri Mar 24, 2006 5:27 pm Post subject: (No subject) |
|
|
Man, that's a long tutorial, nice job  |
|
|
|
|
 |
Cervantes

|
Posted: Fri Mar 24, 2006 8:21 pm Post subject: (No subject) |
|
|
Good stuff, jamonathin.
+200 bits! |
|
|
|
|
 |
Tony
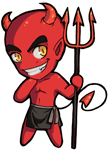
|
|
|
|
 |
Prince Pwn
|
Posted: Tue Dec 26, 2006 1:00 am Post subject: (No subject) |
|
|
How would you use the Dir module to read all of a directory, determine whether other directories exists within that directory and scan them, also checking the files, then exit when you hit as deep as it goes, then goes up a level. Basically like a virus/spyware scanner? I've tried for some time now and never got it down right. |
|
|
|
|
 |
Cervantes

|
Posted: Tue Dec 26, 2006 9:41 am Post subject: (No subject) |
|
|
You need to use recursion. Basically, create a subroutine that scans a directory and performs some action on all the entries. If the entry is a folder, recursively call that same subroutine on that folder. |
|
|
|
|
 |
|
|