Parallel port light chasers.
Author |
Message |
Lapsus Antepedis

|
Posted: Tue Aug 16, 2005 5:43 pm Post subject: Parallel port light chasers. |
|
|
In Computer Engineering last year, we had to make a cable that we could use to control parts of our circuits with the parallel port. To test it, I decided to plug 8 LEDs into my breadboard, one for each data pin...
Needless to say, I was very entertained by my brand new, homebrew 8 pixel screen :P. I lined all of the LEDs in a row and wrote the following program to do something with the lights.
I was then requested by one of my classmates to make the lights go by two, so I wrote this...
He then wanted me to put a space between the two lights as they went, so I wrote this program...
I was then requested to do a pair of lights going back and forth in opposite directions. I'd be willing to bet you are all sick of seeing all of this hardcoded stuff, but I didn't know of the easier way that was pointed out to me by someone who had actually pressed F9 and read the help. Please be patient.
After I read the helpfile, I wrote this much easier to use program.
Turing: |
var light : int := 0
var step : int := 1
loop
light + = step
parallelput (2 ** light )
if light < 1 or light > 6 then
step * = - 1
end if
delay (del )
end loop
|
These are all exceedingly useless programs, and they all require you to have 8 LEDs plugged into your parallel port, but they are pretty cool. Next year I am going to design an LED signboard that uses hardware to scroll the message across the display, and gets the input from turing.
If you have any comments or questions, feel free to ask me.
if there is enough demand, I might be able to find enough LEDs to remake the lighs setup and make a video of what each program does... |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
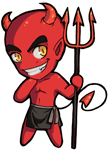
|
Posted: Tue Aug 16, 2005 9:32 pm Post subject: (No subject) |
|
|
well I get a pretty good idea of what they do. But make sure to post pictures for your next year's project  |
|
|
|
|
 |
beard0

|
Posted: Sun Sep 11, 2005 7:33 pm Post subject: (No subject) |
|
|
Sorry for opening an old topic, but I had to comment. Back when you posted this I had just started working with assembly in PIC programming. - It allows you to program a small IC with binary I/O. Anyway, the first kit I used had 5 LEDs that you could control. Naturally, the first porgram I wrote only used one of the LEDs - to flash "Hello World" in morse code  |
|
|
|
|
 |
ZeroPaladn
|
Posted: Tue Sep 13, 2005 1:34 pm Post subject: (No subject) |
|
|
lolz, neat. im doing a "Street Light" in comm tech gr 11, so ill keep this in mind. thanks for the help. |
|
|
|
|
 |
|
|