[Tutorial] JOptionPane: easy input, output with dialog boxes
Author |
Message |
Paul
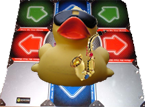
|
Posted: Wed Feb 04, 2004 9:35 pm Post subject: [Tutorial] JOptionPane: easy input, output with dialog boxes |
|
|
JOptionPane is a easy way to do dialog boxes, messages or inputs.
You have to import it at the beginning of the program:
Java: |
import javax.swing.JOptionPane;
|
The arguments for JOptionPane:
for a simple message box:
Java: |
JOptionPane. showMessageDialog (
null, "Put your message here" );
to show text in different rows:
JOptionPane. showMessageDialog (
null, "Put \nyour \nmessage \nhere" );
the \n switches to next line
|
Sample program:
Java: |
//A sample program. Sample.java
import javax.swing.JOptionPane; // imports JOptionPane class
public class Sample {
public static void main ( String args [] )
{
JOptionPane. showMessageDialog (
null, "This is a sample program" );
System. exit ( 0 ); //stops the program
}
}
|
To show different messages with different icons, you'll need 4 arguments instead of 2.
There are different kinds of icons for a dialog box, just replace the last argument:
Java: |
JOptionPane. PLAIN_MESSAGE // this is a plain message
JOptionPane. INFORMATION_MESSAGE // this is a info message
JOptionPane. ERROR_MESSAGE // this is a error message
JOptionPane. WARNING_MESSAGE // this is a warning message
|
You can also use JOptionPane for dialog boxes for input, and assign them to variables, just put:
Java: |
name= // The variable
JOptionPane. showInputDialog ( "put your message here" );
|
note: after using JOptionPane, dont forget to exit System.exit
Sample Program:
Java: |
// Addition.java
// An addition program
import javax.swing.JOptionPane; //import class JOptionPane
public class Addition {
public static void main ( String args [] )
{
String firstNumber, //first string entered by user
secondNumber; // second string entered by user
int number1, // first number to add
number2, //second number to add
sum; //sum of number1 and number2
//read in the first number from user as a string
firstNumber =
JOptionPane. showInputDialog ( "Enter first integer" );
//read in the second number from user as a string
secondNumber =
JOptionPane. showInputDialog ( "Enter second interger" );
//convert numbers from type String to type int
number1 = Integer. parseInt ( firstNumber );
number2 = Integer. parseInt ( secondNumber );
//add the numbers
sum = number1 + number2;
//display the results
JOptionPane. showMessageDialog (
null, "The sum is " + sum, "Results",
JOptionPane. PLAIN_MESSAGE);
System. exit ( 0 ); //ends the program
}
}
|
NOTE:
JOptionPane can ONLY be used to input string, so if you want to use numbers, you have to create separate variables to change it into integers, or real numbers.
PS: I'll add more if needed, if you don't know the parse.Int part, thats ok, as long as you get the main idea of JOptionPane. These may not work 100%, feed back pls.[/b] |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
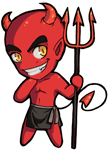
|
Posted: Wed Feb 04, 2004 11:03 pm Post subject: (No subject) |
|
|
nice And this is soo much simpler then setting up input buffers and exseption handlers for basic cin>> Might be using this on CCC if they'll have any questions with manual input
+50Bits |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
the_short1
|
Posted: Fri Apr 08, 2005 1:39 pm Post subject: (No subject) |
|
|
very nice.. i already learned this. .but its a very good tut. . heres 5bits :d |
|
|
|
|
 |
|
|