Generating Multiple Random Numbers
Author |
Message |
Flashkicks

|
Posted: Thu Feb 10, 2005 1:35 pm Post subject: Generating Multiple Random Numbers |
|
|
This is an assignment I have, and the following is the coding. I can not get the "generate 5 random numbers between 1-10" part. [Just ignore the other stuff.. sqrRoot, absolute..etc..] Any help would be nice. I have my program to work, yes; but I think java should be far more capable of figuring out mulitple values in one line rather than having to write it out 5 times. I mean, even Turing just simply uses a for statement.. Can someone tell me how I would use a "for statement" for this?
code: | // The "PowerExample" class.
public class PowerExample
{
public static void main (String [] args)
{
// declare variables
int valueOne, valueTwo;
double answer, randomValue1, randomValue2, randomValue3, randomValue4, randomValue5;
//initialize
valueOne = 4;
valueTwo = 12;
//use Math class to find 4^12
answer = Math.pow (4, 7);
System.out.println (answer);
System.out.println (Math.sqrt (12)); // square root of 12
System.out.println (Math.abs (4 - 12)); // absolute of 4-12
/* Math.random () */
// this whole secion here.. This is Ugly!
randomValue1 = ((Math.random () * 10) + 1); // displays 5 random nums
randomValue2 = ((Math.random () * 10) + 1); // displays 5 random nums
randomValue3 = ((Math.random () * 10) + 1); // displays 5 random nums
randomValue4 = ((Math.random () * 10) + 1); // displays 5 random nums
randomValue5 = ((Math.random () * 10) + 1); // displays 5 random nums
System.out.println (randomValue1 + " " + randomValue2 + " " + randomValue3);
System.out.println (randomValue4 + " " + randomValue5);
} // main method
} // PowerExample class
//experiment with the various methods in the Math class.
//1. Find the square root of 12
//2. Find the absolute value of 4 - 12
//3. Declare a real (double) variable randomValue. Your program should display five random values between 0 and 10.
//4. Find 5 random values between 11 and 95
//5. Make up two questions and display the answer.
|
~Flashkicks |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
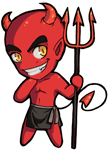
|
Posted: Thu Feb 10, 2005 1:40 pm Post subject: (No subject) |
|
|
Java: |
for(int i= 0;i< 5;i++ )
randomValue [i ] = ((Math. random () * 10) + 1);
|
btw, if you want all 5 digits in a single statement, you can pick a random numbet between (10000 and 99999) and split it up by digits  |
|
|
|
|
 |
Flashkicks

|
Posted: Thu Feb 10, 2005 1:49 pm Post subject: (No subject) |
|
|
Wow I think thats the fastest reply Iv ever seen .. Thanks once again Tony. Your the man ..lol. I shall go try it and I think it will work. I was just playing around with the for loops myself but I couldnt get it to work. So then I tried something called a "while loop" or something.. Still no luck.. But thanks man! I appreciate it!
~Flashkicks
:: UpDated ::
Okay- Tony. I am sorry but it is still not working.. Says randomValue is not an array type. I changed it to int and whatnot [also got rid of the randomValue1, and randomValue2 etc] and nothing will work.. Do you think you could try editing it into my program?. I am sorry to bug you. I shall keep trying..
::: UpDated AGAIN :::
Okay I am sorry to do this but I got it to work.. It was very simple. however, if you look below I am supposed to now do between 11-95 .. THAT--is where I am stuck. I pulled out the ol' reference book and everything. So please.. Someone help..lol This is why i hate Java. Iv always hated Java and I still say its EVIL!!! |
|
|
|
|
 |
Tony
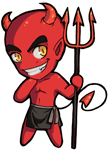
|
Posted: Thu Feb 10, 2005 3:21 pm Post subject: (No subject) |
|
|
Java: |
int randomValue [4];
for(int i= 0;i< 5;i++ )
randomValue [i ] = ((Math. random () * (95- 11)) + 11);
|
|
|
|
|
|
 |
wtd
|
Posted: Thu Feb 10, 2005 3:57 pm Post subject: (No subject) |
|
|
tony wrote: Java: |
int randomValue [4];
for(int i= 0;i< 5;i++ )
randomValue [i ] = ((Math. random () * (95- 11)) + 11);
|
That's just asking for problems. There's only room for 4 values in that array, but you run the loop 5 times.
Java: | // Java 1.5
import java.lang.*;
import java.util.*;
public class RandomNumbers
{
public static void Main ()
{
ArrayList<Integer> randomValue = new ArrayList<Integer> (5);
for (int i = 0; i < 5; i++ )
randomValue. add((Math. random() * (95 - 11)) + 11);
}
} |
|
|
|
|
|
 |
Tony
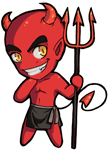
|
Posted: Thu Feb 10, 2005 4:28 pm Post subject: (No subject) |
|
|
aren't Java arrays 0-index? (0,1,2,3,4 ~ 5 elements).
anyways, wtd knows what he's doing. I'm just stabbing in the dark (haven't actually done any Java or even C++ for that matter in a while) |
|
|
|
|
 |
wtd
|
Posted: Thu Feb 10, 2005 4:42 pm Post subject: (No subject) |
|
|
tony wrote: aren't Java arrays 0-index? (0,1,2,3,4 ~ 5 elements).
Yes, but the number you give in the declaration/initialization is the capacity of the array, not the last index.
Of course, I'd still prefer Ruby.
code: | randomValue = Array.new(4) { rand * (95 - 11) + 11 } |
|
|
|
|
|
 |
Flashkicks

|
Posted: Fri Feb 11, 2005 10:14 am Post subject: (No subject) |
|
|
Kay I tried all of your guys' stuff and some of it wasa a bit complicating, while other stuff did kinda work.. So I took your coding and changed it a tad bit and now I got it working. Heres what I used:
code: | for (int i = 0 ; i < 5 ; i++)
System.out.println ((Math.random () * (84)) + 11); // Prints out 5 random numbers [11-95] |
And this did the job fiiine
Thanks for all your help~
~Flashkicks |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Fri Feb 11, 2005 2:59 pm Post subject: (No subject) |
|
|
Flashkicks wrote: Kay I tried all of your guys' stuff and some of it wasa a bit complicating, while other stuff did kinda work.. So I took your coding and changed it a tad bit and now I got it working. Heres what I used:
code: | for (int i = 0 ; i < 5 ; i++)
System.out.println ((Math.random () * (84)) + 11); // Prints out 5 random numbers [11-95] |
And this did the job fiiine
Thanks for all your help~
~Flashkicks
Note: while it may be good Turing style to put space between a function/procedure and the parens that follow it, the more generally accepted style for Java/C/C++/etc. is for there to be no space. Additionally, you can remove a number of parens due to the order of operations. Multiplication is performed first, then addition.
code: | for (int i = 0 ; i < 5 ; i++)
System.out.println(Math.random() * 84 + 11); |
|
|
|
|
|
 |
|
|