Author |
Message |
resq
|
Posted: Sun Feb 06, 2005 10:51 am Post subject: Question about input |
|
|
I'm just learning C++ so there are somethings that are confusing me. i am using g++ on linux to complie my programs.
I have this database of employee records. I am trying to create the user interface for this database. Now how do I make it so I can input commands plus their parameters in one line?
ie) some commands are:
newrec number- makes a new record with 'number' as employee number
printdb - prints the employee records
update number firstname lastname - updates a record with emplloyee number and adds a first name and last name
ok. the problem for me is being able to handle many or no parameters.
if I did this:
string command;
int parameter1;
cin << command << number;
You see that can handle the first command of creating a newrec. But what if I wanted to printdb instead? When I compile and run it, i type printdb, but it is still waiting for another input for parameter1.
I tried doing it another way,
char command[100];
cin.getline(command, 100);
so it takes in the whole line. I use strstr(command, "newrec") to see if it is the newrec command. But for this, I do not know how I would go about extracting the integers for the employee number.
So how can I fix either one of my implementations. Or is there another way I am not seeing? thanks. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
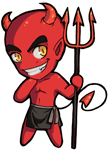
|
Posted: Sun Feb 06, 2005 12:01 pm Post subject: (No subject) |
|
|
you're in the right direction with the second approach - you got to get the entire line.
afterwards you split the line up by spaces and put individual words/numbers into arrays.
your array[0] is your command. your array[n !=0] is your argument if not NULL |
|
|
|
|
 |
resq
|
Posted: Sun Feb 06, 2005 2:11 pm Post subject: (No subject) |
|
|
thanks.. i got my program working now... I found something called istringstream and used that to break up the entire line...ok a follow up... it now works for keyboard input... now how would i get my program to get commands and parameters by getting them line by line from an external file? |
|
|
|
|
 |
bugzpodder

|
Posted: Sun Feb 06, 2005 2:25 pm Post subject: (No subject) |
|
|
add this to the top:
#include<fstream>
add this to the main :
ifstream cin("stupidassignment.txt"); |
|
|
|
|
 |
resq
|
Posted: Sun Feb 06, 2005 2:47 pm Post subject: (No subject) |
|
|
thanks that works.. heh i forgot to check for eof and i ended up rebooting my comp lol... but i think i was dumb to forget to include something in my question... i should have been more specific.. I am particularly interested in getting the commands and parameters from the txt file when i input it in the command line... how do i do that? and what should i include in main() to make it work? reason for this is because i might not know what the file name of the file with the commands might be.
much thanks again. |
|
|
|
|
 |
resq
|
Posted: Sun Feb 06, 2005 3:53 pm Post subject: (No subject) |
|
|
nm i figured it out... ./program < input.dat was what i wanted i spent an hour looking it up
*me tired* |
|
|
|
|
 |
wtd
|
Posted: Sun Feb 06, 2005 4:07 pm Post subject: (No subject) |
|
|
bugzpodder wrote: add this to the top:
#include<fstream>
add this to the main :
ifstream cin("stupidassignment.txt");
Do not redefine cin. It's already defined as something else in the std namespace, and it's not a very descriptive name. |
|
|
|
|
 |
bugzpodder

|
Posted: Sun Feb 06, 2005 4:51 pm Post subject: (No subject) |
|
|
if you have the complete program written using cin's then it is convienient to just redefine cin... search and replace is more work. after all, we programmers are lazy. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Sun Feb 06, 2005 7:41 pm Post subject: (No subject) |
|
|
bugzpodder wrote: if you have the complete program written using cin's then it is convienient to just redefine cin... search and replace is more work. after all, we programmers are lazy.
Lazy programmers use good, meaningful variable names because it makes maintenance so much easier. |
|
|
|
|
 |
|