Martin's Programming Guide
Author |
Message |
Martin

|
Posted: Tue Jan 25, 2005 10:07 pm Post subject: Martin's Programming Guide |
|
|
Little bits of information, I'll update this whenever I think of anything.
Turing
1. Use processes for everything. Need to make a timer? A process is the only way to do it.
code: | process timer
var time : int := 0
loop
time := time + 1
delay(1000)
end loop
end timer |
Other useful applications of processes include catching mouse input, keyboard input, and drawing various objects onto the screen (with things like a 'drawship' process).
2. Never use arrays for anything. Turing has to allocate extra memory to allow you to index the arrays properly, so all that using them will do is make your program run slowly.
3. Avoid using comments in your code, as this will only increase the size of your compiled program, as well as the amount of text that the compiler has to sift through.
4. Shortening your variable names to 1 or 2 characters will also add significantly to speed, as turing doesn't have to cover as much text while compiling your code. Keep in mind that turing is case sensitive, so a variable called 'i' is different than one called 'I'.
5. When inputting numbers, always use integers as the container. Converting numbers from strings to integers is a pain, and slows down everything considerably.
C++
1. Make all of your functions virtual and inline. Virtual makes it so that your function doesn't really exist (but the program thinks it does), and inline makes everything fast.
2. Use global variables whenever you can. By creating instance variables, you are only wasting memory, since every instance of the class will simply add another redundant value.
3. Don't make any member functions private. Writing accessor functions is just boring. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
rizzix
|
Posted: Tue Jan 25, 2005 10:14 pm Post subject: (No subject) |
|
|
ur being sarcastic right?  |
|
|
|
|
 |
Martin

|
Posted: Tue Jan 25, 2005 10:20 pm Post subject: Re: Martin's Programming Guide |
|
|
martin wrote: Little bits of information, I'll update this whenever I think of anything.
Turing
1. Use processes for everything. Need to make a timer? A process is the only way to do it.
code: | process timer
var time : int := 0
loop
time := time + 1
delay(1000)
end loop
end timer |
Other useful applications of processes include catching mouse input, keyboard input, and drawing various objects onto the screen (with things like a 'drawship' process).
2. Never use arrays for anything. Turing has to allocate extra memory to allow you to index the arrays properly, so all that using them will do is make your program run slowly.
3. Avoid using comments in your code, as this will only increase the size of your compiled program, as well as the amount of text that the compiler has to sift through.
4. Shortening your variable names to 1 or 2 characters will also add significantly to speed, as turing doesn't have to cover as much text while compiling your code. Keep in mind that turing is case sensitive, so a variable called 'i' is different than one called 'I'.
5. When inputting numbers, always use integers as the container. Converting numbers from strings to integers is a pain, and slows down everything considerably.
C++
1. Make all of your functions virtual and inline. Virtual makes it so that your function doesn't really exist (but the program thinks it does), and inline makes everything fast.
2. Use global variables whenever you can. By creating instance variables, you are only wasting memory, since every instance of the class will simply add another redundant value.
3. Don't make any member functions private. Writing accessor functions is just boring.
Allow me to quote myself for emphasis, rizzix. |
|
|
|
|
 |
wtd
|
Posted: Tue Jan 25, 2005 10:24 pm Post subject: (No subject) |
|
|
You forgot one that applies to all programming languages:
Never ever create any more functions or procedures than absolutely essential. If you want to reuse code, copy and paste. There's just so much overhead for the computer in calling that function. |
|
|
|
|
 |
Martin

|
Posted: Tue Jan 25, 2005 10:31 pm Post subject: (No subject) |
|
|
See, right now I'm working with the military simulation software ("Magellan") for DND, and, while sifting through the code, I actually came across one of these elusive virtual inline functions. |
|
|
|
|
 |
md

|
Posted: Wed Jan 26, 2005 12:32 pm Post subject: (No subject) |
|
|
Virtual function do infact exist, and they can't be inlined (in C++); so your example is pure bull
As for the rest of 'em, all I can say is 'I see you found a hookup'  |
|
|
|
|
 |
Mazer

|
Posted: Wed Jan 26, 2005 12:37 pm Post subject: (No subject) |
|
|
Martin... this wasn't in any way directed at me was it?  |
|
|
|
|
 |
Catalyst

|
Posted: Wed Jan 26, 2005 3:55 pm Post subject: (No subject) |
|
|
cornflake: wrong
inline virtual functions can exist in c++ |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Martin

|
Posted: Wed Jan 26, 2005 8:51 pm Post subject: (No subject) |
|
|
Yes, they definately exist.
I'd post the source, but...well...I'm not sure if this whole thing is classified or not, so I'll play it safe. |
|
|
|
|
 |
Tony
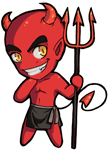
|
Posted: Thu Jan 27, 2005 9:23 am Post subject: (No subject) |
|
|
do what everybody else does and do a global search & replace on some variable identifiers and some function names  |
|
|
|
|
 |
md

|
Posted: Thu Jan 27, 2005 4:29 pm Post subject: (No subject) |
|
|
can they? I thought virtual functions were always called for the real type of a class, not what its currently cast as (i'llgive and example); inlining them would be almost imposible as you can't know what the class is until run-time.
Example
code: |
class a
{
...
virtual void foo(int bar) { ... }
...
}
class b as public a
{
...
void foo(int bar) { ... } // different from above
...
}
a *me;
int x = 10;
if( x == 10)
me = new b();
else
me = new a();
me->foo(x); // this should call b's version, not a's
|
how would the compiler know what function to use at compile time? In a more complex example x might be determined at run-time, and there man be even more classes involved.
[/code] |
|
|
|
|
 |
wtd
|
Posted: Thu Jan 27, 2005 6:20 pm Post subject: (No subject) |
|
|
For virtual functions, as I understand it, the compiler doesn't know at compile-time which function to call. Instead a vtable is created, which is basically a data structure chock full o' function pointers.
Bah... trying to be dynamic in languages not built for it sucks. |
|
|
|
|
 |
|
|