[D] Major Points Of Note For C++ Programmers
Author |
Message |
wtd
|
Posted: Sat Oct 30, 2004 3:59 am Post subject: [D] Major Points Of Note For C++ Programmers |
|
|
Forward declarations are unnecessary
The D compiler evaluates the entire source file, so forward declarations of functions are never needed.
C++
code: | #include <iostream>
void foo();
int main()
{
foo();
return 0;
}
void foo()
{
std::cout << "Bar" << std::endl;
} |
D
code: | import std.stream;
int main()
{
foo();
return 0;
}
void foo()
{
stdout.writeLine("Bar");
} |
More to come. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Andy
|
Posted: Sat Oct 30, 2004 9:49 am Post subject: (No subject) |
|
|
cool.. no need for prototypes... |
|
|
|
|
 |
Tony
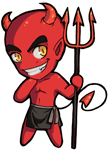
|
Posted: Sat Oct 30, 2004 2:53 pm Post subject: (No subject) |
|
|
ohh.. I'm liking D thus far.. what else does it have to offer? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
wtd
|
Posted: Sat Oct 30, 2004 4:38 pm Post subject: (No subject) |
|
|
Say goodbye to #include
D adopts the Java-ish "import" syntax, but puts an even better module system behind it.
Let's say I have two source files:
foo.d:
code: | private import std.stream;
void bar()
{
stdout.writeLine("bar");
} |
And my main file, test.d:
code: | import foo;
int main()
{
bar();
return 0;
} |
And I compile and run like so:
code: | saneones@lcars:~ $ dmd test foo
gcc test.o foo.o -o test -lphobos -lpthread -lm
saneones@lcars:~ $ ./test
bar
saneones@lcars:~ $ |
Now you're probably first wondering about that "private". It means that essentially, even though I've imported the std.stream module into the foo module, anything that imports foo shouldn't automatically import std.stream.
Now, did I do anything to indicate that foo.d was a module? Nope. Any file is automatically a module that can be reused. Just take off the file extension and you're set to go.
Update: the DigitalMars D compiler doesn't seem to enforce private imports yet. Oh well. Still an improvement. |
|
|
|
|
 |
wtd
|
Posted: Sat Oct 30, 2004 5:34 pm Post subject: (No subject) |
|
|
Function parameters
C++ supports passing variables to functions by reference, but it uses the & operator, which can get lost in a function declaration. D improves on this by introducing three new keywords: in, out, and inout.
Consider the following trivial example:
C++
code: | void setInt(int& dest, int newValue)
{
dest = newValue;
} |
D
code: | void setInt(out int dest, int newValue)
{
dest = newValue;
} |
The "in" version is used by default. "inout" is used when you want to both read the value in a variable and write to it.
C++
code: | int setInt(int& dest, int newValue)
{
return dest = newValue;
} |
D
code: | int setInt(inout int dest, int newValue)
{
return dest = newValue;
} |
Of course, whatyou should be picking up from these examples, is that D in many ways improves on C++, but it's also not that different, either. |
|
|
|
|
 |
wtd
|
Posted: Sat Oct 30, 2004 6:39 pm Post subject: (No subject) |
|
|
Design by Contract: Pre and Post Conditions, Classes, and Properties, oh my!
Let's say we have a "NameBase" class. Yes, this is one of my favorte trivial examples.
C++
code: | #include <string>
class Name
{
private:
std::string m_first, m_last;
public:
Name(std::string initFirst, std::string initLast)
: m_first(initFirst), m_last(initLast)
{ }
};
int main()
{
Name bob("Bob", "Smith");
return 0;
} |
D
code: | class Name
{
private char[] m_first, m_last;
public this(char[] initFirst, char[] initLast)
{
m_first = initFirst;
m_last = initLast;
}
}
int main()
{
Name bob = new Name("Bob", "Smith");
return 0;
} |
Now, we'll extend it so that we can get access to the parts of the person's name, and also change them.
C++
code: | #include <string>
#include <iostream>
class Name
{
private:
std::string first, last;
public:
Name(std::string initFirst, std::string initLast)
: first(initFirst), last(initLast)
{ }
std::string getFirst() { return first; }
std::string getLast() { return last; }
std::string setFirst(std::string newFirst) { return first = newFirst; }
std::string setLast(std::string newLast) { return last = newLast; }
std::string fullName() { return first + " " + last; }
};
int main()
{
Name bob("Bob", "Smith");
bob.setFirst("John");
bob.setLast("Doe");
std::cout << bob.fullName() << std::endl;
return 0;
} |
D
code: | import std.stream;
class Name
{
private char[] m_first, m_last;
public
{
this(char[] initFirst, char[] initLast)
{
m_first = initFirst;
m_last = initLast;
}
char[] first() { return m_first; }
char[] last() { return m_last; }
char[] first(char[] newFirst) { return m_first = newFirst; }
char[] last(char[] newLast) { return m_last = newLast; }
char[] fullName() { return m_first + " " + m_last; }
}
}
int main()
{
Name bob = new Name("Bob", "Smith");
bob.first = "John";
bob.last = "Doe";
stdout.writeLine(bob.fullName());
return 0;
} |
So, there's properties demonstrated, and some nifty bits about classes, including constructors. So, we're left with pre and post conditions.
Let's play with inheritance and create NotStupidName class which basically puts pre and post conditions on our ability to set the first and last names. People with the same firs and last names sound kinda stupid, so we'll prevent that.
code: | import std.stream;
class Name
{
private char[] m_first, m_last;
public
{
this(char[] initFirst, char[] initLast)
{
m_first = initFirst;
m_last = initLast;
}
char[] first() { return m_first; }
char[] last() { return m_last; }
char[] first(char[] newFirst) { return m_first = newFirst; }
char[] last(char[] newLast) { return m_last = newLast; }
char[] fullName() { return m_first ~ " " ~ m_last; }
}
}
class NotStupidName : Name
{
public
{
this(char[] initFirst, char[] initLast)
{
super(initFirst, initLast);
}
char[] first() { return super.first(); }
char[] last() { return super.last(); }
char[] first(char[] newFirst)
in { assert(newFirst != this.last); }
out { assert(this.first != this.last); }
body { return super.first = newFirst; }
char[] last(char[] newLast)
in { assert(newLast != this.first); }
out { assert(this.first != this.last); }
body { return super.last = newLast; }
}
}
int main()
{
NotStupidName bob = new NotStupidName("Bob", "Smith");
try
{
bob.first = "Smith";
stdout.writeLine(bob.fullName());
}
catch (Error)
{
stderr.writeLine("Stupid, stupid name...");
}
return 0;
} |
|
|
|
|
|
 |
wtd
|
Posted: Sat Oct 30, 2004 10:05 pm Post subject: (No subject) |
|
|
Back to little things
Time for a break after that last post.
The foreach loop
Much like the most recent version of Java and C#, D provides a convenient way to loop over aggregate data structures like arrays.
code: | import std.stream;
int main()
{
int[4] foo;
foo[0] = 2;
foo[1] = 3;
foo[2] = 9;
foo[3] = 7;
foreach (int i; foo)
{
stdout.writefln("%d", i);
}
} |
Of course, we can use the foreach loop to modify the contents of an array too.
code: | import std.stream;
int main()
{
int[4] foo;
foo[0] = 2;
foo[1] = 3;
foo[2] = 9;
foo[3] = 7;
foreach (inout int i; foo)
{
i++;
stdout.writefln("%d", i);
}
} |
Did I mention that strings are just arrays of characters? That means we can use strings in foreach loops too.
code: | import std.stream;
int main()
{
foreach (char ch; "hello")
{
stdout.writefln("%c", ch);
}
} |
|
|
|
|
|
 |
wtd
|
Posted: Sat Oct 30, 2004 10:57 pm Post subject: (No subject) |
|
|
Switch works on strings
code: | switch ("hello")
{
case "world":
stdout.writeLine("Yo");
break;
default:
stdout.writeLine("Oy...");
break;
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
rizzix
|
Posted: Sat Oct 30, 2004 11:13 pm Post subject: (No subject) |
|
|
i like the consistancy in D.. although i prefer strings as objects than characters... nywyz c++ should've really been like D.. its the c++ that is not backward compatible with a cleaned up syntax.. too bad it does not have much support yet.
apple should've gone for D than obj-c,, and it's easier to map D to Java.
(not implying that obj-c is any less good.. it has it's own strengths) |
|
|
|
|
 |
wtd
|
Posted: Sat Oct 30, 2004 11:32 pm Post subject: (No subject) |
|
|
Well, D didn't exist at the time (1997), and NeXT already had a very mature, extensive framework written in Objective-C. Besides, Objective-C is vastly more dynamic than C++, Java or D. Very few languages could do the same job.
As for strings... strings are objects in Java because... well anything that isn't a integral type or float is an object. In C++ it's to make up for the fact that arrays in C++ are just glorified pointers.
Arrays in D are more sophisticated, so it's quite acceptable that strings aren't objects. |
|
|
|
|
 |
rizzix
|
Posted: Sat Oct 30, 2004 11:35 pm Post subject: (No subject) |
|
|
do arrays in D hold length information?
edit: nvm they do... nice and there's support for slicing too.. sweet. but that is as far as it goes.. oh and they have the cleaner syntax for Dictionaries. |
|
|
|
|
 |
wtd
|
Posted: Sun Oct 31, 2004 12:45 am Post subject: (No subject) |
|
|
Debugging simplified
test.d
code: | import std.stream;
int main()
{
debug (Foo) stdout.writeLine("Entering main function...");
debug (Foo) stdout.writeLine("Exiting main function...");
return 0;
} |
Compiling it:
code: | $ dmd test -debug=Foo
gcc test.o -o test -lphobos -lpthread -lm
$ ./test
Entering main function...
Exiting main function...
$ dmd test
gcc test.o -o test -lphobos -lpthread -lm
$ ./test
$ |
|
|
|
|
|
 |
|
|