Author |
Message |
wtd
|
Posted: Mon Oct 04, 2004 5:54 pm Post subject: [Tutorial] Putting data into a string |
|
|
Yesterday I wrote a tutorial on extracting data from a string using the istringstream class. Today I'll cover putting data into a string using the ostringstream class.
code: | #include <string>
#include <sstream>
#include <iostream>
int main()
{
std::ostringstream output;
int i = 37;
double d = 27.42;
output << i << d;
std::string final_string = output.str();
std::cout << final_string << std::endl;
return 0;
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
rizzix
|
Posted: Mon Oct 04, 2004 9:36 pm Post subject: (No subject) |
|
|
thats rather cool. so ehm.. would u suggest using this over sprintf/sscanf ? |
|
|
|
|
 |
wtd
|
Posted: Mon Oct 04, 2004 10:11 pm Post subject: (No subject) |
|
|
If you're writing C code, write C (sprintf, sscanf, etc). If you're writing C++, then write C++.  |
|
|
|
|
 |
rizzix
|
Posted: Mon Oct 04, 2004 10:20 pm Post subject: (No subject) |
|
|
ha well .. but i find it easier to do error checking using stdio than using iostreams.
for ex:
code: |
int num;
char* str = "1000";
if (!sscanf(str, "%d", &i))
printf("error\n");
|
and the syntax is a lot cleaner.. no operator overloading .. although that shouldn't bug a c++ programmer a lot.. i rather like a consistent behaviour. and the c libraries tend to provide that.
but things would be different if iostream supported exceptions. |
|
|
|
|
 |
wtd
|
Posted: Tue Oct 05, 2004 12:23 am Post subject: (No subject) |
|
|
The problem with stdio.h is the danger of buffer overflows. |
|
|
|
|
 |
Tony
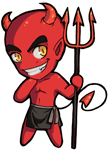
|
Posted: Wed Oct 06, 2004 4:15 pm Post subject: (No subject) |
|
|
i seem to be having trouble figuring out how to flush ostringstream  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|