Something related to loops i think
Author |
Message |
JR
|
Posted: Mon Sep 27, 2004 5:18 pm Post subject: Something related to loops i think |
|
|
Well i got this assigment which i have a starting rate of $2500 and i need to program to tell me after how many years the rate will be $5000 if theres a 7.5% compuned annualy.
Heres what i got for now but it doesnt seem to work...
code: |
#include <iostream.h>
void main ()
{
float starting_rate;
float percent;
float per;
float years=0;
float final;
starting_rate=2500;
percent=0.075;
while (starting_rate < 5001)
{
per=starting_rate*percent;
final=starting_rate+per;
final=starting_rate;
years=years+1;
if (final=5000)
{
break;
}
}
cout<<"it will take:"<<years<<"year";
cin.get();
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
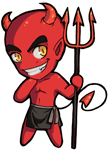
|
Posted: Mon Sep 27, 2004 8:11 pm Post subject: (No subject) |
|
|
eh? isn't it something like
code: |
5000 = 2500(1.07)^x
|
solving for x you end up with about 10.25 years |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
wtd
|
Posted: Mon Sep 27, 2004 8:35 pm Post subject: (No subject) |
|
|
Several notes:
Header files
code: | #include <iostream.h> |
The header "iostream.h" is obsolete.
code: | #include <iostream> |
Is the standard way of doing things.
The type of "main"
Indicates that "main" is a procedure with no return value.
Is the proper way of writing this. If "main" returns an int, then the program can return a value indicating whether or not the program executed successfully, or if it failed, how.
Declare and initialize your variables together
code: | float starting_rate;
...
starting_rate = 2500; |
This just makes your code longer than it has to be.
code: | float starting_rate = 2500; |
This is much more concise, and ultimately more readable.
Using assignment operators
This is overly lengthy.
This is more concise, and shorter code often leaves less opportunity for mistakes.
Use whitespace well
Your code is easier to understand if you use space judiciously. For instance, put spaces between most operators and values and variables. Secondly, use tabs to indent your code. This will make it easier to follow the flow of the program.
Properly use the std namespace
You haven't included "using namespace std;" anywhere in your program, so you'd have to use "std::cout" and "std::cin" instead of "cut" and "cin".
Finally, the program cleaned up
code: | #include <iostream>
int main()
{
float starting_amount = 2500;
float running_amount = starting_amount;
float percent = 0.075;
int years = 0;
do
{
years += 1;
running_amount += running_amount * percent;
} while ((running_amount * (1 + percent)) <= 5000);
std::cout << "The years it takes: " << years << std::endl;
std::cout << "And the final amount: " << running_amount << std::endl;
}
|
|
|
|
|
|
 |
|
|