Cycle() and print() function
Author |
Message |
tinhnho
|
Posted: Mon Mar 22, 2004 1:01 pm Post subject: Cycle() and print() function |
|
|
Hi everyone
please help me this proplem,i'm really appreciate.
Question:
Write a cycle, and print function follow difination
Quote:
template<class TYPE>
void cycle(TYPE& a,TYPE& b, TYPE& c)
{
//replace a's value by b's value and b's by c's and c's by a's
}[/code]
print() function which prints value a,b,c
EX: print(a,b,c);
Two generic function using example values of data typ char,int,double.
Here what i did:
[code]
#include <iostream>
#include <cstdlib>
int main()
{
int i,j,e;
cycle(i,j,e);
print(i,j,e);
system("PAUSE");
return 0;
}
template<class TYPE>
void cycle(TYPE& a, TYPE& b, TYPE& c)
{
TYPE temp;
//replace a's value by b's value
temp = a;
a = b;
b = temp;
//replace b's value by c's value
TYPE temp1;
temp1 = b;
b = c;
c = temp1;
//replace b's value by c's value
TYPE temp2;
temp2 =c;
c= a;
a =temp2;
}
template<class TYPE>
void print(TYPE& a, TYPE& b, TYPE& c)
{
cout<< a << b << c <<endl;
}
note: i sitll working on it,sorry if my code look messed up.Should i use this ?:
[code]
template <class TYPE>
class triple
{
public:
...........
private:
TYPE a,b,c;
}[/code] |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
xtxnx
|
Posted: Mon Mar 22, 2004 4:34 pm Post subject: (No subject) |
|
|
i think you should do :
template<class type>
class triple
{
}
int main()
{
.....
return 0.
}
void cycle(Type& a, Type& b, Type& c)
{
.....
}
void print(Type& a, Type& b, Type& c)
{
............
}
good luck |
|
|
|
|
 |
Tony
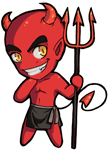
|
|
|
|
 |
wtd
|
Posted: Mon Mar 22, 2004 7:05 pm Post subject: (No subject) |
|
|
You could certainly wrap it up in a class. This has one small error, but shift_right works.
code: | #include <iostream>
template <typename _t, unsigned int _length>
class bundle
{
private:
_t storage[_length];
unsigned int number_stored;
public:
bundle();
void add(_t);
_t at(unsigned int) const;
bool full();
template <unsigned int _slots>
void shift_right();
template <typename __t, unsigned int __length>
friend std::ostream& operator<<(std::ostream&, const bundle<__t, __length>&);
};
template <typename _t>
class triple : public bundle<_t, 3>
{
public:
triple();
void cycle();
_t get_first() const;
_t get_second() const;
_t get_third() const;
};
int main()
{
triple<int> t;
t.add(4);
t.add(5);
t.add(2);
std::cout << t << std::endl;
t.shift_right<1>();
std::cout << t << std::endl;
return 0;
}
template <typename _t, unsigned int _length>
bundle<_t, _length>::bundle()
: number_stored(0)
{
for (int i = 0; i < _length; i++)
storage[i] = _t();
}
template <typename _t, unsigned int _length>
void bundle<_t, _length>::add(_t new_element)
{
if (!full())
storage[number_stored++] = new_element;
}
template <typename _t, unsigned int _length>
_t bundle<_t, _length>::at(unsigned int position) const
{
return storage[position];
}
template <typename _t, unsigned int _length>
bool bundle<_t, _length>::full()
{
return number_stored >= _length;
}
template <typename _t, unsigned int _length>
template <unsigned int _slots>
void bundle<_t, _length>::shift_right()
{
_t temp = storage[_length - 1];
for (int i = _length - 1; i >= 0; i--)
{
storage[i] = storage[i - 1];
}
storage[0] = temp;
}
template <typename _t, unsigned int _length>
std::ostream& operator<<(std::ostream& out, const bundle<_t, _length>& b)
{
for (int i = 0; i < _length; i++)
out << b.at(i);
return out;
}
template <typename _t>
triple<_t>::triple()
: bundle<_t, 3>()
{ }
template <typename _t>
void triple<_t>::cycle()
{
shift_right<1>();
}
template <typename _t>
_t triple<_t>::get_first() const
{
return at(0);
}
template <typename _t>
_t triple<_t>::get_second() const
{
return at(1);
}
template <typename _t>
_t triple<_t>::get_third() const
{
return at(2);
}
|
|
|
|
|
|
 |
tinhnho
|
Posted: Mon Mar 22, 2004 8:40 pm Post subject: (No subject) |
|
|
thanks WTD, i changed and i compiled your program it work,and the output :
452
245
But are there anyway shorter than this?like i thought from beginning |
|
|
|
|
 |
tinhnho
|
Posted: Tue Mar 23, 2004 4:07 pm Post subject: (No subject) |
|
|
Thanks wtd,unfortunatly my intructor doesn't accept that code.Here what i did,Please correct me if i was wrong,i'm appreacitate that.
code: |
#include <iostream>
template<class Type>
class triple
{
public :
triple(Type x):a(x),b(x+1),c(x+2){}
void print(){};
void cycle(){};
private:
Type a, b, c;
};
int main()
{
triple<char> p1('A');
triple<int> p2(1);
triple<double> p3(1.2);
//Print them before cycle
p1.print();
p2.print();
p3.print();
//Cycle them and print
p1.cycle();
p2.cycle();
p3.cycle();
p1.print();
p2.print();
p3.print();
system("PAUSE");
return 0;
}
template <class Type>
void cycle()
{
Type temp;
temp = a;
a = b;
b = c;
c = temp;
}
template <class Type>
void print()
{
cout<< a << b << c <<endl;
} |
note:when i compiling it,the screen is just empty,it doesn't tell me any error |
|
|
|
|
 |
tinhnho
|
Posted: Tue Mar 23, 2004 6:43 pm Post subject: (No subject) |
|
|
Here is the original question:
Create the print() function,that will print the values a,b,and c.Also create the cycle()function follow this difination
code: |
template<class TYPE>
void cycle(TYPE& a,TYPE& b, TYPE& c)
{
//replace a's value by b's value and b's by c's and c's by a's
} |
print() function which prints value a,b,c
EX: print(a,b,c);
Two generic function using example values of data typ char,int,double. |
|
|
|
|
 |
wtd
|
Posted: Tue Mar 23, 2004 7:09 pm Post subject: (No subject) |
|
|
This acts a bit peculiarly when dealing with doubles, but I simplified it a bit by removing the inheritance.
code: | #include <iostream>
template <typename _t>
class triple
{
private:
_t first, second, third;
public:
triple(_t);
// Overload the member operator <<
// instead of:
// void cycle();
triple<_t>& operator<<(unsigned int);
// Plus add an operator that cycles
// in the opposite direction, just
// for the heck of it:
triple<_t>& operator>>(unsigned int);
// Overload the << in place of this:
// void print() const;
template <typename __t>
friend std::ostream& operator<<(std::ostream&, const triple<__t>&);
};
int main()
{
triple<char> p1('A');
triple<int> p2(1);
triple<double> p3(1.2);
std::cout << p1 << std::endl;
std::cout << p2 << std::endl;
std::cout << p2 << std::endl;
// Shift two spots left,
// then twice right, then
// back one right. :-)
p1 << 2 >> 2 << 1;
p2 << 1;
p3 << 1;
std::cout << p1 << std::endl;
std::cout << p2 << std::endl;
std::cout << p2 << std::endl;
return 0;
}
template <typename _t>
triple<_t>::triple(_t init_first)
: first(init_first)
, second(init_first + static_cast<_t>(1))
, third(init_first + static_cast<_t>(2))
{ }
template <typename _t>
triple<_t>& triple<_t>::operator<<(unsigned int places)
{
for (int i = 0; i < places; i++)
{
_t temp = first;
first = second;
second = third;
third = temp;
}
return *this;
}
template <typename _t>
triple<_t>& triple<_t>::operator>>(unsigned int places)
{
for (int i = 0; i < places; i++)
{
_t temp = third;
third = second;
second = first;
first = temp;
}
return *this;
}
template <typename _t>
std::ostream& operator<<(std::ostream& out, const triple<_t>& t)
{
return out << t.first << " "
<< t.second << " "
<< t.third << std::endl;
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
tinhnho
|
Posted: Wed Mar 24, 2004 9:34 am Post subject: (No subject) |
|
|
thanks wtd,but the assigment is required write cycle() function and print() |
|
|
|
|
 |
tinhnho
|
Posted: Wed Mar 24, 2004 11:38 am Post subject: (No subject) |
|
|
i got it,thanks wtd alot  |
|
|
|
|
 |
wtd
|
Posted: Wed Mar 24, 2004 6:51 pm Post subject: (No subject) |
|
|
tinhnho wrote: thanks wtd,but the assigment is required write cycle() function and print()
That's only because your teachers are trying to teach you "C++ as a super-duper C", which is so blatantly false it makes me want to cry.
And you're welcome.  |
|
|
|
|
 |
tinhnho
|
Posted: Thu Mar 25, 2004 9:30 am Post subject: (No subject) |
|
|
i got it work today ,thanks wtd |
|
|
|
|
 |
|
|