Please help with String
Author |
Message |
tinhnho
|
Posted: Tue Feb 24, 2004 11:25 pm Post subject: Please help with String |
|
|
Write the following member function:
// strcmp is negative if s < s1
// is 0 if s == s1
// and positive if s > s1
// where s is the emplicite argument
int my_string::strcmp(const my_string& s1);
// strrev reverses the my_string
void my_string::strrev();
//print oeverloaded to print the frist n characters
void my_string:: print(int n);
here is what i got so far:
code: |
#include <iostream>
#include <cstdlib>
#include <string>
using namespace std;
class my_string
{
public:
my_string();
my_string(const my_string& str);
my_string(const char* p);
~my_string();
int strcmp(const my_string& s1);
void strrev();
void print(int n);
int const getLen();
const char* getS();
private:
char* s;
int len;
};
my_string::my_string(): len(0),s (0){}
my_string::my_string(const char* p)
{
len = strlen (p);
s = new char[len +1];
assert(s !=0);
strcpy(s, p);
}
my_string::my_string(const my_string& str):len(str.len)
{
s = new char[len+1];
assert(s !=0);
strcpy(s, str.s);
}
// Destructor
my_string::~my_string()
{
delete [] s;
}
int my_string::strcmp(const my_string& s1)
{
int i;
for(i=0; s1.s[i] && s[i] && s1.s[i] == s[i]; ++i)
{
return s1.s[i]-s[i];
}
}
void my_string::strrev()
{
my_string strrev;
char* temp = new char[len + 1];
int i= 0;
int j= 0;
while (s[i])
{
temp[j++] = s[i--];
}
temp[j] ='\0';
strcpy(s,temp);
delete[] temp;
}
void my_string::print(int n)
{
return ;
}
int const my_string::getLen()
{
return len;
}
const char* my_string::getS()
{
return s;
}
int main ()
{
my_string myStr ("same");
if (myStr.strcmp ("same") < 0)
cout << myStr.getS() << " < " << "same" << endl;
else if (myStr.strcmp ("same") == 0)
cout << myStr.getS() << " = " << "same" << endl;
else
cout << myStr.getS() << " > " << "same" << endl;
cout << "Reverse string " << myStr.getS() << " to ";
myStr.strrev();
myStr.print();
system("pause");
} |
when i compile it ,the compiler telling me the error: " No matching function for call to my_string::print()" and "Candidates are : void my_string::print()".Please tell me where did i go wrong,thanks alot
Note:
code: |
Use the following string to test your function strcmp:
1st string 2nd string
gladiator gladiolus
xyz abcd
same same |
Can not use predefined strrev function. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
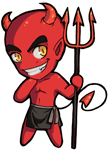
|
|
|
|
 |
wtd
|
Posted: Wed Feb 25, 2004 1:28 am Post subject: (No subject) |
|
|
It's C++, and the problem is that you've defined the print function to take one argument of type int, yet when you call it, you call it without arguments. |
|
|
|
|
 |
tinhnho
|
Posted: Wed Feb 25, 2004 9:33 am Post subject: (No subject) |
|
|
wtd wrote: It's C++, and the problem is that you've defined the print function to take one argument of type int, yet when you call it, you call it without arguments.
should i go for this:
code: |
void my_string::print(int n)
{
return my_string(s,n);
} |
|
|
|
|
|
 |
Tony
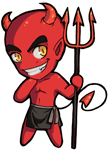
|
|
|
|
 |
tinhnho
|
Posted: Wed Feb 25, 2004 12:55 pm Post subject: (No subject) |
|
|
my bad,it should not have statemen in there |
|
|
|
|
 |
tinhnho
|
Posted: Wed Feb 25, 2004 6:45 pm Post subject: (No subject) |
|
|
here is what i have done.I compiled it and it said something about run time error , there are no message error.I'm using Dev C++.Thanks
code: |
#include <iostream>
#include <cstdlib>
#include <string>
using std::cout;
class my_string
{
public:
my_string();
my_string(const my_string& str);
my_string(const char* p);
~my_string();
int strcmp(const my_string& s1);
void strrev();
void print(int n=0);
int const getLen();
const char* getS();
private:
char* s;
int len;
};
my_string::my_string(): len(0),s (0){}
my_string::my_string(const char* p)
{
len = strlen (p);
s = new char[len +1];
assert(s !=0);
strcpy(s, p);
}
my_string::my_string(const my_string& str):len(str.len)
{
s = new char[len+1];
assert(s !=0);
strcpy(s, str.s);
}
// Destructor
my_string::~my_string()
{
delete [] s;
}
//strcmp
int my_string::strcmp(const my_string& s1)
{
int i;
while(s1.s[i] && s[i] && s1.s[i] == s[i])
{
i++;
}
return s1.s[i]-s[i];
}
//strrev
void my_string::strrev()
{
char* temp = new char[len + 1];
int i = len -1;
int j= 0;
while (s[i])
{
temp[j++] = s[i--];
}
temp[j] ='\0';
strcpy(s,temp);
delete[] temp;
}
void my_string::print(int n)
{
if (n > len || n == 0) n = len;
for (int i = 0; i < n; ++i)
cout << s[i];
cout << endl;
}
int const my_string::getLen()
{
return len;
}
const char* my_string::getS()
{
return s;
}
int main ()
{
my_string myStr("gladiator");
if (myStr.strcmp ("gladiolus") < 0)
cout << myStr.getS() << " < " << "gladiolus" << endl;
else if (myStr.strcmp ("gladiolus") == 0)
cout << myStr.getS() << " = " << "gladiolus" << endl;
else
cout << myStr.getS() << " > " << "gladiolus" << endl;
cout << "Reverse string " << myStr.getS() << " to ";
myStr.strrev();
myStr.print();
system("pause");
} |
|
|
|
|
|
 |
wtd
|
Posted: Wed Feb 25, 2004 7:07 pm Post subject: (No subject) |
|
|
Hopefully the following will prove educational. I cleaned it up quite a bit.
code: | #include <iostream>
#include <cstdlib>
#include <string>
class my_string
{
private:
char * s;
int len;
public:
my_string();
my_string(const my_string& str);
my_string(const char * p);
~my_string();
int compare(const my_string& s1) const;
void reverse();
int length() const;
const char * c_string() const;
friend std::ostream& operator<<(std::ostream& out, const my_string& str);
};
//*********************************************
int main ()
{
using namespace std;
my_string my_str("Same");
switch (my_str.compare("SAme"))
{
case -1:
cout << my_str << " < " << "SAme" << endl;
break;
case 0:
cout << my_str << " = " << "SAme" << endl;
break;
case 1:
cout << my_str << " > " << "SAme" << endl;
break;
default:
cout << "Uh... that shouldn't have happened..." << endl;
}
cout << "Reverse string " << my_str << " to ";
my_str.reverse();
cout << my_str << endl;
system("pause");
}
//*********************************************
my_string::my_string()
: len(0)
, s(0)
{ }
my_string::my_string(const char * p)
: len(strlen(p))
{
s = new char[len + 1];
assert(s != 0);
strncpy(s, p, strlen(p));
}
my_string::my_string(const my_string& str)
: len(str.length())
{
s = new char[len + 1];
assert(s != 0);
strncpy(s, str.c_string(), str.length());
}
// Destructor
my_string::~my_string()
{
delete [] s;
}
int my_string::compare(const my_string& s1) const
{
return strcmp(c_string(), s1.c_string());
}
void my_string::reverse()
{
my_string new_string;
char * temp = new char[length()];
for (int i = 0; i < length(); i++)
temp[length() - i - 1] = s[i];
strncpy(s, temp, length());
}
int my_string::length() const
{
return len;
}
const char * my_string::c_string() const
{
return s;
}
std::ostream& operator<<(std::ostream& out, const my_string& str)
{
return out << str.c_string();
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|