Author |
Message |
Tony
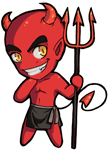
|
Posted: Mon Mar 10, 2003 1:27 am Post subject: [tutorial] If - elsif - else statments |
|
|
Some basics you cant program without - If statments
An if statment is a command that compares 2 (or more) values and outputs based on the result.
The only program you can make without an if stament is "Hello World" one. In the rest, you have some values to compare.
code: |
if tony = "cool" then
put "tony is cool"
elsif tony = "not cool" then
put "error, something wrong with your program"
else
put "something something, blah blah blah"
end if
|
this statment compares the value of variable tony to different values and if one of them matches, it outputs a result. If none are matched, it outputs the commands under else
You can have as many elsifs as you'd like. Just keep on adding them.
Also you dont have to have elsif or else. You can have both, or just 1 or none. You always need to start with an if though.
Someone once asked the difference between case and if and as far as I can see... If statments offer you much more control. You're not limited to just = comparisment. You can have much, much more
<
<=
=
>=
>
not=
and
or
you can use a combination of those to check for very spesific conditions.
code: |
if ((tony = "cool") or (dan = "cool")) and (numposts >= 1000) then
put "compsci has over 1000 posts and a cool admin"
end if
|
As you can see if first checks if ether tony or dan is cool. And if one of those is true, the overall statment is true. Then it checks if number of posts is more or equals to a 1000 and returns true/false for that. Now if both left and right side of "and" are true, then the overall statment is true.
confusing, isn't it? Thats why I used brakets to show each individual comparisment. You dont have to use that, but it just shows you how your if statment is structured and keeps trouble away when you have a bunch of "or" and "and" statments.
Hope this clears things up. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Namis
|
Posted: Thu Sep 11, 2003 2:55 pm Post subject: Im using Turing 4.0.4c Problem |
|
|
this is my code, im new so bare with me :\
var one:string
var two:string
put " Yes or No? "
if one = "Yes" then
put " Good Choice! "
elsif No = "No" then
put " Bad Choice! "
Im getting an error in
elsif No = "No" Then
Whats wrong? Thanks |
|
|
|
|
 |
krishon
|
Posted: Thu Sep 11, 2003 4:32 pm Post subject: (No subject) |
|
|
maybe u shuld add an input line........ |
|
|
|
|
 |
Tony
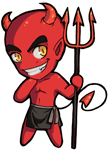
|
Posted: Thu Sep 11, 2003 4:39 pm Post subject: (No subject) |
|
|
well first of all, you didnt declear "No"... I think you wanted to use variable named "one" instead since you used it in
if one = "Yes" then...
Now there's soo many things wrong with this program... I donno where to start.
Lets start with bigest and most obvious mistakes:
The variable you're using in your ifstatment has no value. You should promp to enter their choice and assign input to the variable
code: |
var choice:string
put "enter choice"
get choice
put choice
|
You should read up a tutorial on I/O (input/output for more details).
also variable named "one" doesnt make much sence. one is a number, so a line "if one = "Yes" then" doesnt make much sence. I know it would work ether way, but if you would name your variables appropriatly, it would be just easier for yourself to understand how your own code works.
Also you forgot to end your if statment.
Here's how your program should have looked like:
code: |
var choice:string
put "enter choice, yes/no"
get choice
if choice="yes" then
put "you have picked yes"
else
put "you did not pick yes"
end if
|
I hope that helped - and dont worry... You'll get better with practice and understanding of how code works - just read some tutorials we got here and look around the site 8) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
krishon
|
Posted: Thu Sep 11, 2003 4:41 pm Post subject: (No subject) |
|
|
lol, ye tony put everything in a nutshell  |
|
|
|
|
 |
theguru
|
Posted: Fri Oct 07, 2005 10:03 pm Post subject: (No subject) |
|
|
this tutorial has everything you need to know about the if statement. i was wondering why turing has elsif instead of elseif. isn't that wierd.  |
|
|
|
|
 |
[Gandalf]

|
Posted: Fri Oct 07, 2005 10:27 pm Post subject: (No subject) |
|
|
Quite a few programming languages have it like that actually.
I think they reason behind it is to make a better differentiation between 'else' and 'if' so that you know it does something else, and so that newbs don't mistake it for and 'else' preceding an 'if'. |
|
|
|
|
 |
wtd
|
Posted: Fri Oct 07, 2005 11:03 pm Post subject: (No subject) |
|
|
"else if" is a way of having multiple clauses without actually having anything more complex than "if" and "else". |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
[Gandalf]

|
Posted: Sat Oct 08, 2005 4:40 pm Post subject: (No subject) |
|
|
Yeah, I forgot about that.
It wouldn't work quite well in Turing though:
code: | var word : string
get word
if word (1) = 'a' then
put "yes"
else
if word (1) = 'b' then
put "no"
else
if word (1) = 'c' then
put "this is even deeper.."
end if
end if
end if |
 |
|
|
|
|
 |
MysticVegeta

|
Posted: Tue Nov 08, 2005 8:19 pm Post subject: Re: [tutorial] If - elsif - else statments |
|
|
Tony wrote:
code: |
elsif tony = "not cool" then
put "error, something wrong with your program"
|
oh my god LOL really humorous! |
|
|
|
|
 |
xXInsanityXx

|
Posted: Mon Nov 28, 2005 8:39 pm Post subject: (No subject) |
|
|
This is an excellent Tut, for the super newbs, and gets them into basic programming logic. helped me a lot as a beginer thanks a lot |
|
|
|
|
 |
Nose_Pilot44
|
Posted: Mon Nov 28, 2005 10:19 pm Post subject: (No subject) |
|
|
keep getting an error. says "Syntax error at 'var' expected ':'" and it says "syntax error at then"
code: | var num1
var num2
get num1
get num2
if num1 > num2 then
put "Num1 is greater"
elsif num2 > num1 then
put "Num2 is greater"
elsif num1 = num2 then
put "They're equal"
elsif then
put "error! omgwtfbbq!"
end if |
|
|
|
|
|
 |
Mr. T

|
Posted: Mon Nov 28, 2005 11:34 pm Post subject: Alex's Opinion |
|
|
code: | var num1 : int
var num2 : int
get num1
get num2
if num1 > num2 then
put "Num1 is greater"
elsif num2 > num1 then
put "Num2 is greater"
elsif num1 = num2 then
put "They're equal"
else
put "error! omgwtfbbq!"
end if |
1) You were declaring the variables incorrectly
2) else covers every other option that doesn't fall under the if or elsif conditions |
|
|
|
|
 |
DIIST

|
Posted: Mon Dec 19, 2005 7:43 am Post subject: (No subject) |
|
|
'If's are nice but case statments become lot easier and faster when you are dealing for known values like yes and no or 1 and 0!
code: |
var answer : string
loop
put "Is compsci.ca cool?"
get answer
case answer of
label "Yes", "Y", "yes", "y" :
put "You are so right"
exit
label "No", "no", "n", "N" :
put "Are you crazy?"
exit
label :
put "You did not answer correctly!"
end case
end loop
|
|
|
|
|
|
 |
Cervantes

|
Posted: Mon Dec 19, 2005 5:35 pm Post subject: (No subject) |
|
|
thuvs wrote: yes and no or 1 and 0!
yes = 1
no = 0?
How about:
yes = true
no = false
Cases are nice, but less flexible than if statements. |
|
|
|
|
 |
|