A Detailed Tutorial of Raybiss by Jack140
Author |
Message |
Jack140
|
Posted: Fri Nov 14, 2008 1:51 pm Post subject: A Detailed Tutorial of Raybiss by Jack140 |
|
|
Hello All,
Here is a simple step by step tutorial on how to make a similar game that I made (Raybiss).
To begin, you must chose an adventure or have a game plan. You must make a map of your game (Include rooms, inventory items ect. Anything that is relevant.)
Once you've established a setting or situation, let's get on with the tutorial.
1. In order to be successful, the program must be neat, organize and carefully planned. Such as a program header...
Example: (You'll obviously have to change a few things, this is my example from my game that I made)
Turing: | % Author: Jack140
% Date: Monday, September 29, 2008
% Filename: Raybiss.t
% Description: RAYBISS - A game of strategy and adventure.
% You've the only survivor, who had survived from a horrible hurricane,
% you must find a way to be rescued. Remember even thou you are alone,
% there are full of surprise ahead to watch out for...
|
2. The next simple step is to set your screen...
Turing: | setscreen ("graphics") % This enables you to add in graphics
setscreen ("graphics:1000,650") % This adjusts your screen size, you can adjust it to whatever you like
|
3. Next is to declare you variables, all of the variables for this game will be located at the top or below the setscreen commands, or else your program wouldn't function properly.
Turing: | % Declare Raybiss Variables
% Game Options Variables
var name : string % You can ask the user to input their name
var age : real % You can ask the user to input their age
var continue : string % I will show you this variable later
var ready : string
var input : string
% Inventory Variables % This is where you add all of the items found in this game
var key: int:=0 % Example is the key, key is = 0 because the user sis not find the key, if the user found the key then key = 1, therefore you can use it
var keyanswer:string % This is where the user inputs "grab key" or "use key"
var radio: int :=0 % Another item
var radioanswer : string
% Area Variables % This is where you organize your rooms or places
var answer : string % The answer would be to go north, south, east or west
var area : int % Say if you started out in your basement then basement would be equal to area 1, and so on
area := 0 |
4. Once you've finished organizing your variables you will have to introduce your game to the user (or whoever is playing it)...
Turing: | % Introduction to Raybiss and Melanie and Ratings %Melanie is just someone that will explain it to the user
put " RAYBISS "
put skip %Skip command is to skip a few lines
color (2)
put "'Hello, My name Is Melanie, your guide to Raybiss.' "
put "'What is your name?' " ..
get name :*
put " "
put "'Before we start, I need to know your age?'" ..
get age
loop
if age <= 12. 99 then
put "'Your too young to play,", name, " wait until your 13. "
put " This game may contain scences of violence viewer discretion is advised!'"
put "'Just kidding you can play, there are no graphical images in this game'"
put "'Here are the following rules:'"
end if
exit when age <= 12. 99
if age >= 50 then
put "'Sorry, you are too old to play'... ('Just Kidding! ", name, "')"
put "'Here are the following rules:'"
end if
exit when age >= 50
if age >= 13 then
put "'You are allowed to play this game!'", name
put "'Here are the following rules:'"
end if
exit when age >= 13
end loop
put skip
color (3)
% Declare Rules of Game
put " How to play Raybiss Rules: "
put " "
put " 1. You must indicate the direction you are willing to travel "
put " (North, South, East or West) "
put " "
put " 2. You can pick up items and use them for your advantages "
put " "
put " 3. You must clearly use two words... a Verb and a Noun (Ex. use/pickup key), "
put " to activate the action, so that you are able to, "
put " use or pickup any objects, that you've found. "
put " "
put " 4. You must be rescued to be able to win the game "
put " "
loop
put " type 'continue', to proceed "
get input :*
exit when input = "continue"
end loop
put " "
% Explaining Situations
color (12)
put " 'Hi ", name ..
put " this is Melanie, your guide'"
put " "
put " 'Firstly, I will like to introduce you to my game called 'Raybiss' "
put " So...lets begin!, this is your situation..."
put " You are currently in need of help, "
put " meaning that you've just survived from a devastating hurricane. "
put " In fact you are the only survivor, nobody else had survived this deadly strike, "
put " Here are some objective you must accomplish...'"
put " "
loop
put " type 'continue', to proceed "
get input : *
exit when input = "continue"
end loop
put " "
% Explaining Objectives and Circumstances
color (9)
put " Objectives: "
put " 1. Find basic survival supplies (Food, Shelter,) "
put " 2. Find Telecommunication Equipment "
put " 3. Obviously, you must be rescued "
put " 4. Have Fun!!! "
put " "
put " 'Where are you?, you are in your basement. To the east, you see an exit, "
put " Which leads upstairs, I reccommend you go there, and get out of the flooded "
put " basement, but before I go, ", name ..
put ", "
put " I must warn you of the possible upcoming surprises, Good Luck' "
put " "
loop
put " type 'ready', to begin your game... "
get input : *
exit when input = "ready"
end loop |
5. Once your finished with the introduction, its time to make the game!!!
This part gets tricky... Just try to follow along. I will explain how it works...
Turing: | %//////////////////////Gaming Begins////////////////////////////%
proc basement % You have to make individual procedures for each room or place
put "You are currently in the basement. Exit is to the east"
area := 1 % Assign areas to your rooms or places (staring with the first one which is equal to 1 and so on)
end basement
proc room
put "You are in a room. Exits to the north, south and west"
area := 2
end room
proc kitchen
put "You are in the kitchen. Exits to north, west and south"
area := 3
end kitchen
proc livingroom
put "You are currently in your living room. Exit to the east "
if radio = 0 then % If you want the user to notice the radio, you must make the item to be equal to 0. Then when user picks up the item, then the item will be equal to 1
put "you see a radio"
put "It might be useful"
get radioanswer:*
if radioanswer = "get radio" or radioanswer = "grab radio" or radioanswer = "take radio" or radioanswer = "pickup radio" then
put "you've got the radio! Go to your backyard and test the radio out"
radio := 1
else
put "I don't know what that means. Try different words." % If the user doesn't type the following words or misspelled a word this will pop up
end if
end if
area := 4
end livingroom
proc frontdoor
put "You are at the front door, Apparently it has been damaged and blocked,"
put " Please find another exit. Exit is to the north (kitchen)"
area := 5
end frontdoor
proc backyard
put "You are in your backyard. Exits is to the east, west and south"
if radio = 1 then
put "Alright hopefully the radio will work out here"
get radioanswer :*
if radioanswer = "try radio" or radioanswer = "use radio" or radioanswer = "test radio" then
put "Sorry, your radio connection has failed and its been damaged from the flood"
end if
end if
area := 6
end backyard
proc shed
put "You are in the shed. Exit is to the east"
if key = 0 then
put "you see a key"
put "what do you want to do?"
get keyanswer:*
if keyanswer = "get key" or keyanswer = "grab key" or keyanswer = "pickup key" or keyanswer = "take key" then
put "you got the key!"
key:= 1
else
put "I don't know what that means."
end if
end if
area := 7
end shed
proc gate
put "You are at the gates. Exit is to the west unless you have a key"
put "You can then access to many more exits. "
if key = 1 then %If the user has the key with him, then he could use it to unlock the gate and continue, but if he doesn't have the key from the shed, then he won't be able % to access the gate
put "Hey, it looks like your key might be able fit into the lock"
get keyanswer:*
if keyanswer="try key" or keyanswer = "use key" or keyanswer = "open lock with key" or keyanswer = "open with key" then
put "Your key has successfully unlocked the gate!. It releases a monster"
put " Game Over! You are instantly Dead! Haha"
put " Just kidding, My game isn't finished "
put " Congrats you've made it through section 1 of 4."
end if
end if
area := 8
end gate |
6. The next part is pretty significant... This part of the program allows you to move back and forth between rooms or places.
The code is simple, when you think of it
My game has a primary or seconday paths, you can just have a primary path in it, its entirly up to you
Primary path = You must take this path to win the game
Secondar Path = Its not mandatory, but you can finds some stuff to help you for along the way
Turing: | loop
% Basement to Room, Room to Basement (Primary Path)
if area = 1 and (answer = "go east" or answer = "east" or answer = "e") then
room
elsif area = 2 and (answer = "go west" or answer = "west" or answer = "w") then
basement
% Room to Kitchen, Kitchen to Room (Secondary Path)
elsif area = 2 and (answer = "go south" or answer = "south" or answer = "s") then
kitchen
elsif area = 3 and (answer = "go north" or answer = "north" or answer = "n") then
room
% Room to Backyard, Backyard to Room (Primary Path)
elsif area = 6 and (answer = "go south" or answer = "south" or answer = "s") then
room
elsif area = 2 and (answer = "go north" or answer = "north" or answer = "n") then
backyard
% Kitchen to Front Door, Front Door to Kitchen (Secondary Path)
elsif area = 3 and (answer = "go south" or answer = "south" or answer = "s") then
frontdoor
elsif area = 5 and (answer = "go north" or answer = "north" or answer = "n") then
kitchen
% Kitchen to Living Room, Living Room to Kitchen (Secondary Path)
elsif area = 3 and (answer = "go west" or answer = "west" or answer = "w") then
livingroom
elsif area = 4 and (answer = "go east" or answer = "east" or answer = "e") then
kitchen
% Backyard to Shed, Shed to Backyard (Primary Path)
elsif area = 6 and (answer = "go west" or answer = "west" or answer = "w") then
shed
elsif area = 7 and (answer = "go east" or answer = "east" or answer = "e") then
backyard
% Backyard to Gate, Gate to Backyard (Primary Path)
elsif area = 6 and (answer = "go east" or answer = "east" or answer = "e") then
gate
elsif area = 8 and (answer = "go west" or answer = "west" or answer = "w") then
backyard
% If they do not type the required word, this will pop up %
put "I don't Know the word...'", answer, "' "
put "Please type a different word(s) "
end if
put " "
get answer :*
end loop |
I hope you now have a better understanding of how this game works
Good Luck, and Have Fun
If you have any questions, in regards to this code, please reply and I will hopefully replay back with an answer to your questions.
Enjoy! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
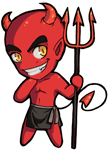
|
Posted: Fri Nov 14, 2008 3:04 pm Post subject: RE:A Detailed Tutorial of Raybiss by Jack140 |
|
|
things like
code: |
answer = "go east" or answer = "east" or answer = "e"
|
should probably be in a function.
Also, you have a redundancy in establishing the paths. Once in the procedure for a given room
code: |
put "You are in a room. Exits to the north, south and west"
|
and in 3 more places, one for each choice.
That's an absolute mess to maintain. Just think of how much work it will be to add or remote an area from the game. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Jack140
|
Posted: Fri Nov 14, 2008 4:14 pm Post subject: Re: A Detailed Tutorial of Raybiss by Jack140 |
|
|
I totally agree with you Tony,
I will have to educate myself, because I have no idea what functions are...
then I can convert that mess that your talking about into something more established.
Thanks Tony for you help  |
|
|
|
|
 |
Tony
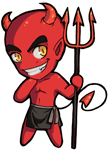
|
Posted: Fri Nov 14, 2008 6:20 pm Post subject: RE:A Detailed Tutorial of Raybiss by Jack140 |
|
|
procedures are functions that return void (no value). I've noticed that you are already using procedures, so it shouldn't be that difficult to get a hold of the functions.
There's a link to a couple of tutorials on the subject, available through the Turing Walkthrough -- check it out. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|