Text Based RPG(wacky kind)
Author |
Message |
Tat
|
Posted: Wed Feb 04, 2004 9:02 pm Post subject: Text Based RPG(wacky kind) |
|
|
code: | /*Tat Ho
Me=Newb in Java
this program wasn't fully finished but finished enough to play around with
this program was also done for my java class
save it as thRPGMain.java
u also need KeyboardReader
which i will post after this
*PRPG Project*/
class CharacterType
{
String name;
KeyboardReader reader=new KeyboardReader();
int money, hp, mp, strength, mstrength, choice;
boolean isTurn;
Special item;
int curAtt;
CharacterType()
{
name="Character";
money=100;
}
void displayInfo()
{
System.out.println("Name: " + name);
System.out.println("Money: " + money);
System.out.println("Health: " + hp);
System.out.println("Mana: " + mp);
System.out.println("Strength: " + strength);
System.out.println("MStrength: " + mstrength);
}
int attack()
{
curAtt=(int)Math.floor(Math.random()*strength);
return curAtt;
}
int useSpecial()
{
if(item instanceof Weapon){
curAtt=(int)Math.floor(Math.random()*(strength+item.strength));
return curAtt;
}else{
if(mp>=((Spell)item).manacost){
mp-=((Spell)item).manacost;
curAtt=(int)Math.floor(Math.random()*(mstrength+item.strength));
return curAtt;
}else{
System.out.print("Not enough mana for that spell, therefore you will only use your fist!!!");
curAtt=(int)Math.floor(Math.random()*(strength));
return curAtt;
}
}
}
boolean isDead()
{
if(hp<=0){
hp=0;
return true;
}else
return false;
}
void wins(int money)
{
this.money+=money;
System.out.println(name + " Wins!!!");
}
void loses()
{
System.out.println(name + " Loses!!!");
}
void flees()
{
System.out.println(name + " Flees!!!");
}
void changeTurn()
{
if(isTurn==false)
isTurn=true;
else
isTurn=false;
}
void menu()
{
choice=reader.readInt("1) Attack \n2) Use Special \n3) Flee");
}
}
class User extends CharacterType
{
KeyboardReader reader;
User()
{
name="User";
reader=new KeyboardReader();
}
User(String type)
{
if(type.equals("Barbarian")){
name="Barbarian";
hp=120;
mp=40;
strength=80;
mstrength=20;
}
else if(type.equals("Mage")){
name="Mage";
hp=80;
mp=100;
strength=30;
mstrength=90;
}
else if(type.equals("Elf")){
name="Elf";
hp=100;
mp=70;
strength=60;
mstrength=60;
}else{
name="Barbarian";
hp=120;
mp=40;
strength=80;
mstrength=20;
}
}
void inputName()
{
name=reader.readLine("Enter your name");
}
void inputChoice()
{
do{
menu();
if(choice<1 || choice>3)
System.out.println("Invalid choice please enter again");
}while(choice<1 || choice>3);
}
}
class Comp extends CharacterType
{
Comp()
{
name="Comp";
strength=100;
hp=200;
mp=0;
mstrength=0;
item=new Weapon("Axe");
}
void setChoice()
{
choice=(int)Math.floor(Math.random()*100);
if(choice==0)
flees();
else if(choice>0 || choice<20)
attack();
else
useSpecial();
}
}
abstract class Special
{
String name;
int strength;
int cost;
/*abstract void displayInfo()
{
}*/
int use()
{
int use=(int)Math.floor(Math.random()*strength);
return use;
}
}
class Weapon extends Special
{
Weapon()
{
name="Weapon";
}
Weapon(String weapon)
{
if(weapon.equals("Sword")){
name="Sword";
strength=40;
}else if(weapon.equals("Axe")){
name="Axe";
strength=60;
}else if(weapon.equals("Staff")){
name="Staff";
strength=50;
}else{
name="Sword";
strength=40;
}
}
void displayInfo()
{
System.out.println("Name: " + name);
System.out.println("Strength " + strength);
System.out.println("Cost: " + cost);
}
}
class Spell extends Special
{
int manacost;
Spell()
{
name="Spell";
}
Spell(String spell)
{
if(spell.equals("Fire1")){
name="Fire1";
strength=40;
manacost=20;
}else if(spell.equals("Fire2")){
name="Fire2";
strength=60;
manacost=40;
}else if(spell.equals("Fire3")){
name="Fire3";
strength=80;
manacost=60;
}else{
name="Fire1";
strength=40;
manacost=20;
}
}
void displayInfo()
{
System.out.println("Name: " + name);
System.out.println("Strength " + strength);
System.out.println("ManaCost: " +manacost);
System.out.println("Cost: " + cost);
}
}
class Fight
{
User player;
KeyboardReader reader;
Comp boss;
char again;
{
boss=new Comp();
reader=new KeyboardReader();
}
void chooseCharacter()
{
int choice;
do{
choice=reader.readInt("Choose your character\n1) Elf\n2) Mage\n3) Barbarian\n");
switch(choice)
{
case 1:player=new User("Elf");break;
case 2:player=new User("Mage");break;
case 3:player=new User("Barbarian");break;
default:System.out.println("No such choice!!!");
}
}while(choice<1 || choice>3);
}
void shop()
{
int choice;
do{
choice=reader.readInt("Special Shop\n1) Sword\n2) Axe\n3) Staff\n4) Fire1\n5) Fire2\n6) Fire3\n");
switch(choice)
{
case 1:player.item=new Weapon("Sword");break;
case 2:player.item=new Weapon("Axe");break;
case 3:player.item=new Weapon("Staff");break;
case 4:player.item=new Spell("Fire");break;
case 5:player.item=new Spell("Fire2");break;
case 6:player.item=new Spell("Fire3");break;
default:System.out.println("No such choice!!!");
}
}while(choice<1 || choice>6);
}
void fight()
{
do{
player.inputChoice();
switch(player.choice)
{
case 1: player.attack();
boss.hp-=player.curAtt;
System.out.println(player.name+" attacks with " + player.curAtt + " damage with a remaining of " + player.hp + "hp");
boss.setChoice();
player.hp-=boss.curAtt;
System.out.println(boss.name+" attacks with " + boss.curAtt + " damage with a remaining of " + boss.hp + "hp");
break;
case 2: player.useSpecial();
boss.hp-=player.curAtt;
System.out.println(player.name+" attacks with " + player.curAtt + " damage with a remaining of " + player.hp + "hp");
boss.setChoice();
player.hp-=boss.curAtt;
System.out.println(boss.name+" attacks with " + boss.curAtt + " damage with a remaining of " + boss.hp + "hp");
break;
case 3:player.flees();break;
default:System.out.println("No such choice!!!");
}
}while(player.isDead()==false && boss.isDead()==false);
if(player.hp>boss.hp){
System.out.println();
player.wins(900);
boss.loses();
player.displayInfo();
}else if(boss.hp>player.hp){
System.out.println();
boss.wins(0);
player.loses();
boss.displayInfo();
}
}
void run()
{
do{
boss.hp=200;
chooseCharacter();
shop();
fight();
again=reader.readChar("Want to play again, enter y or Y to do so");
}while(again=='y' || again=='Y');
}
}
class thRPGMain
{
static Fight fight=new Fight();
public static void main(String args[])
{
fight.run();
}
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tat
|
Posted: Wed Feb 04, 2004 9:03 pm Post subject: (No subject) |
|
|
code: | /*you need to save it as KeyboardReader.java in the same folder with the other file
*/
//Copyright Martin Osborne and Ken Lambert 1998-2001
//All rights reserved
import java.io.*;
/**
* The class KeyboardReader contains input methods for terminal I/O. Type-specific
* methods read characters, integers, doubles, and strings. Another
* method pauses output and waits for the user to press the enter key
* to continue. Examples:
*
*
* KeyboardReader reader = new KeyboardReader();
* char letter = reader.readChar ("Enter a letter: ");
* double d = reader.readDouble("Enter a real number: ");
* int i = reader.readInt ("Enter an integer: ");
* String name = reader.readLine ("Enter your full name: ");
* reader.pause();
*
*
*/
public class KeyboardReader{
private InputStreamReader reader;
private BufferedReader buffer;
public KeyboardReader(){
reader = new InputStreamReader (System.in);
buffer = new BufferedReader (reader);
}
/**
* Used with non-GUI applications to prevents a "fly-by"
* disappearance of the terminal window
* in some environments by pausing execution until the user
* presses the Enter key. Usage: reader.pause();
*/
public void pause(){
System.out.print ("\nPress Enter to continue . . . ");
try {
buffer.readLine();
}catch (Exception e){
System.exit(0);
}
}
/**
* Prompts the user and waits for integer input. Throws an exception
* if the input doesn't represent an integer. Returns the integer entered.
* @param prompt the prompt to the user.
*/
public int readInt(String prompt){
int value = 0;
String s = "";
System.out.print (prompt);
try {
s = (buffer.readLine()).trim();
value = (new Integer(s)).intValue();
}catch (Exception e){
System.out.println
("\n\nError: your input doesn't represent a valid integer value\n");
pause();
System.exit(0);
}
return value;
}
/**
* Waits for integer input without prompting the user. Throws an exception
* if the input doesn't represent an integer. Returns the integer entered.
*/
public int readInt(){
return readInt ("");
}
/**
* Prompts the user and waits for double input. Throws an exception
* if the input doesn't represent a double. Returns the double entered.
* @param prompt the prompt to the user.
*/
public double readDouble(String prompt){
double value = 0;
String s = "";
System.out.print (prompt);
try {
s = (buffer.readLine()).trim();
value = (new Double(s)).doubleValue();
}catch (Exception e){
System.out.println
("\n\nError: your input doesn't represent a valid double value\n");
pause();
System.exit(0);
}
return value;
}
/**
* Waits for double input without prompting the user. Throws an exception
* if the input doesn't represent a double. Returns the double entered.
*/
public double readDouble(){
return readDouble("");
}
/**
* Prompts the user and waits for character input. Returns the char entered.
* @param prompt the prompt to the user.
*/
public char readChar(String prompt){
int value = 0;
String s = "";
System.out.print (prompt);
try {
s = buffer.readLine();
s += "?";
value = s.charAt(0);
}catch (Exception e){
System.out.println
("\n\nError in method readChar:\n" + e.toString() + "\n");
pause();
System.exit(0);
}
return (char)value;
}
/**
* Waits for character input without prompting the user. Returns the char entered.
*/
public char readChar(){
return readChar("");
}
/**
* Prompts the user and waits for string input. Returns the entire line
* of text entered.
* @param prompt the prompt to the user.
*/
public String readLine(String prompt){
String value = "";
System.out.print (prompt);
try {
value = buffer.readLine();
}catch (Exception e){
System.out.println
("\n\nError in Console.readLine\n" + e.toString() + "\n");
pause();
System.exit(0);
}
return value;
}
/**
* Waits for string input without prompting the user. Returns the entire line
* of text entered.
*/
public String readLine(){
return readLine("");
}
} |
|
|
|
|
|
 |
shorthair

|
Posted: Wed Feb 04, 2004 9:06 pm Post subject: (No subject) |
|
|
How long have you been coding , this is quality stuff especially cause its writtin in such a unique language ,well done your doing awsome keep up the amazing code |
|
|
|
|
 |
Tat
|
Posted: Wed Feb 04, 2004 9:13 pm Post subject: (No subject) |
|
|
ty for ur complement
this is only um....text based stuff
i took java for half a year already 1 period a day
now 2 periods a day in ap java(starting this week) |
|
|
|
|
 |
Tony
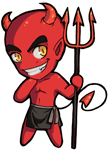
|
Posted: Wed Feb 04, 2004 11:12 pm Post subject: (No subject) |
|
|
I'm supposedly taking java in AP CS, but things aren't working out too great I dont know much Java... I have no clue how I'm gonna do CCC this year and I'm really hoping that AP exam is multiple choice  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|