Text Based Ceelo
Author |
Message |
Tat
|
Posted: Wed Feb 04, 2004 8:43 pm Post subject: Text Based Ceelo |
|
|
code: | /*Tat Ho
Me=Newb in Java
CeeloGame written for my java class a while ago*/
class Die
{
int value;
void setRandomValue()
{
value=(int)Math.floor(Math.random()*6+1);
}
void showValue()
{
System.out.print(value);
}
void roll()
{
setRandomValue();
showValue();
}
}
class Dice
{
Die die1, die2, die3;
Dice()
{
die1=new Die();
die2=new Die();
die3=new Die();
}
void roll()
{
die1.roll();
die2.roll();
die3.roll();
}
}
class Player
{
String name;
int money,score;
final int bet=10;
boolean isTurn;
KeyboardReader reader;
int die1, die2, die3;
Player()
{
name="Player";
money=100;
reader=new KeyboardReader();
}
void showInfo()
{
System.out.println(name);
System.out.println("Money: " + money);
System.out.println("Bet: " + bet);
System.out.println();
}
void enterName()
{
name=reader.readLine("Enter your name: ");
}
void enterBet()
{
money-=bet;
System.out.print(name);
System.out.println(" bets " + bet);
}
void wins()
{
money+=20;
isTurn=true;
System.out.println(name + " wins!");
}
void loses()
{
isTurn=false;
System.out.println(name + " loses!");
}
boolean isBroke()
{
if(money>=0)
return true;
else
return false;
}
void tie()
{
System.out.println("Tie!");
money+=10;
}
}
class Ceelo
{
Player player1, player2;
KeyboardReader reader=new KeyboardReader();
Dice dice;
int value1;
int value2;
int value3;
int values;
int score1;
int score2;
boolean valid;
Ceelo()
{
player1=new Player();
player1.isTurn=true;
player2=new Player();
player2.isTurn=true;
dice=new Dice();
values=value1+value2+value3;
valid=false;
}
void title()
{
System.out.println("Ceelo");
reader.pause();
}
void enterNames()
{
player1.enterName();
player2.enterName();
}
void takeBets()
{
player1.enterBet();
player2.enterBet();
}
int calcRoll()
{
int score=-1;
if (value1 == value2 && value2 == value3) {
valid = true;
score = value1 + 6;
System.out.print(" score "+score);
}
else {
if (value1 == value3) {
valid = true;
score = value2;
System.out.print(" score "+score);
}
else if (value1 == value2) {
valid = true;
score = value3;
System.out.print(" score "+score);
}
else if (value2 == value3) {
valid = true;
score = value1;
System.out.print(" score "+score);
}
else if (values == 6) {
valid = true;
score = 0;
System.out.print(" score "+score);
}
else if (values == 15) {
valid = true;
score = 13;
System.out.print(" score "+score);
}
}
return score;
}
void rollBoth()
{
System.out.print(player1.name+" rolls ");
dice.roll();
value1=dice.die1.value;
value2=dice.die2.value;
value3=dice.die3.value;
score1=calcRoll();
System.out.println();
System.out.print(player2.name+" rolls ");
dice.roll();
value1=dice.die1.value;
value2=dice.die2.value;
value3=dice.die3.value;
score2=calcRoll();
System.out.println();
if (score1==-1 ||score2==-1){
System.out.println("No Score! Roll Again!");
rollBoth();
}
}
void findWinner()
{
if(score1>score2){
player1.wins();
player2.loses();
}else if(score2>score1){
player2.wins();
player1.loses();
}else if (score2==score1){
player1.tie();
player2.tie();
}
}
void play()
{
char again;
title();
enterNames();
do
{
takeBets();
rollBoth();
findWinner();
System.out.println(player1.name + " Money: " + player1.money);
System.out.println(player2.name + " Money: " + player2.money);
again=reader.readChar("Press 'Y' to play again or 'N' to exit");
}while(again=='Y' || again=='y');
if(again=='n' || again=='N' || isGameOver())
System.out.println("Exit");
}
boolean isGameOver()
{
if(player1.isBroke()==true || player2.isBroke()==true)
{
return true;
}
else
{
return false;
}
}
}
class CeeloMain
{
static Ceelo ceelo;
public static void main(String args[])
{
ceelo=new Ceelo();
ceelo.play();
}
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tat
|
Posted: Wed Feb 04, 2004 8:55 pm Post subject: (No subject) |
|
|
code: | /*opps almost forgot u need keyboard reader(which was given by the teacher)
Save it as KeyboardReader.java and put it in the same folder as CeeloMain.java
*/
//Copyright Martin Osborne and Ken Lambert 1998-2001
//All rights reserved
import java.io.*;
/**
* The class KeyboardReader contains input methods for terminal I/O. Type-specific
* methods read characters, integers, doubles, and strings. Another
* method pauses output and waits for the user to press the enter key
* to continue. Examples:
* <pre>
*
* KeyboardReader reader = new KeyboardReader();
* char letter = reader.readChar ("Enter a letter: ");
* double d = reader.readDouble("Enter a real number: ");
* int i = reader.readInt ("Enter an integer: ");
* String name = reader.readLine ("Enter your full name: ");
* reader.pause();
*
* </pre>
*/
public class KeyboardReader{
private InputStreamReader reader;
private BufferedReader buffer;
public KeyboardReader(){
reader = new InputStreamReader (System.in);
buffer = new BufferedReader (reader);
}
/**
* Used with non-GUI applications to prevents a "fly-by"
* disappearance of the terminal window
* in some environments by pausing execution until the user
* presses the Enter key. Usage: reader.pause();
*/
public void pause(){
System.out.print ("\nPress Enter to continue . . . ");
try {
buffer.readLine();
}catch (Exception e){
System.exit(0);
}
}
/**
* Prompts the user and waits for integer input. Throws an exception
* if the input doesn't represent an integer. Returns the integer entered.
* @param prompt the prompt to the user.
*/
public int readInt(String prompt){
int value = 0;
String s = "";
System.out.print (prompt);
try {
s = (buffer.readLine()).trim();
value = (new Integer(s)).intValue();
}catch (Exception e){
System.out.println
("\n\nError: your input doesn't represent a valid integer value\n");
pause();
System.exit(0);
}
return value;
}
/**
* Waits for integer input without prompting the user. Throws an exception
* if the input doesn't represent an integer. Returns the integer entered.
*/
public int readInt(){
return readInt ("");
}
/**
* Prompts the user and waits for double input. Throws an exception
* if the input doesn't represent a double. Returns the double entered.
* @param prompt the prompt to the user.
*/
public double readDouble(String prompt){
double value = 0;
String s = "";
System.out.print (prompt);
try {
s = (buffer.readLine()).trim();
value = (new Double(s)).doubleValue();
}catch (Exception e){
System.out.println
("\n\nError: your input doesn't represent a valid double value\n");
pause();
System.exit(0);
}
return value;
}
/**
* Waits for double input without prompting the user. Throws an exception
* if the input doesn't represent a double. Returns the double entered.
*/
public double readDouble(){
return readDouble("");
}
/**
* Prompts the user and waits for character input. Returns the char entered.
* @param prompt the prompt to the user.
*/
public char readChar(String prompt){
int value = 0;
String s = "";
System.out.print (prompt);
try {
s = buffer.readLine();
s += "?";
value = s.charAt(0);
}catch (Exception e){
System.out.println
("\n\nError in method readChar:\n" + e.toString() + "\n");
pause();
System.exit(0);
}
return (char)value;
}
/**
* Waits for character input without prompting the user. Returns the char entered.
*/
public char readChar(){
return readChar("");
}
/**
* Prompts the user and waits for string input. Returns the entire line
* of text entered.
* @param prompt the prompt to the user.
*/
public String readLine(String prompt){
String value = "";
System.out.print (prompt);
try {
value = buffer.readLine();
}catch (Exception e){
System.out.println
("\n\nError in Console.readLine\n" + e.toString() + "\n");
pause();
System.exit(0);
}
return value;
}
/**
* Waits for string input without prompting the user. Returns the entire line
* of text entered.
*/
public String readLine(){
return readLine("");
}
} |
|
|
|
|
|
 |
Paul
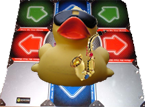
|
Posted: Wed Feb 04, 2004 9:08 pm Post subject: (No subject) |
|
|
Good job! Though its not all that exciting looking here, cause I run from MsDos prompt. Im really noob at this, so Im really impressed, your code will teach me alot. +10 bits  |
|
|
|
|
 |
|
|