recursive method help
Author |
Message |
Krocker
|
Posted: Fri Nov 29, 2013 10:38 am Post subject: recursive method help |
|
|
Hi, so i have to make a recursive method that calculates a to the power n. I have an idea on how to go about this, but its not working for me and i cant seem to get this working, this is the first time im wokring with recursive method, so im having a bit of difficulty. I either get java.lang.StackOverflow error or an Infinite answer.
code: | public static double exponential (int a, int n){
int exp = 0;
double result=0;
if (exp != n) {
result = a*Math.pow(a,exponential(a,n-1));
exp++;
}
return result;
} |
[/code] |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
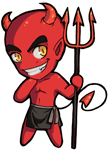
|
Posted: Fri Nov 29, 2013 3:28 pm Post subject: RE:recursive method help |
|
|
Since you are using Math.pow anyway, you can skip the recursion.
code: |
public static double exponential (int a, int n){
return Math.pow(a,n);
}
|
Of course this defeats the purpose of the exercise, so this should be a clue that the use of Math.pow is something that is obviously wrong.
Recursion is easy if you find the right answer to ask. E.g.
- assuming that you already (somehow) know what the value of 2^10 is, how would you calculate the value of 2^11 ? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Krocker
|
Posted: Fri Nov 29, 2013 4:05 pm Post subject: Re: recursive method help |
|
|
ok.... so i removed the Math.pow, so now its something like this:
code: |
public static int exponential (int a, int n){
int exp = 0;
int result=0;
if (exp < n) {
result = a * a;
exp++;
exponential (result, n);
}
return result;
}
|
but it doesnt work. IM so lost  |
|
|
|
|
 |
Insectoid

|
Posted: Fri Nov 29, 2013 4:24 pm Post subject: RE:recursive method help |
|
|
What you're doing is multiplying A by itself, then multiplying the new A by the new A, then that new A by that new A, etc.
ie, if a = 2, b = 4, then it looks like this
2*2
4*4
16*16
It should look like this:
2*2
2*4
2*8
So you really need to change the line 'result = a * a' to something else. |
|
|
|
|
 |
Krocker
|
Posted: Fri Nov 29, 2013 11:25 pm Post subject: Re: recursive method help |
|
|
ok, at the when the method repeats when it calls itself, will it also do the initialization of the 1st two variable? if so, then how would i initialize them without them resetting? Also, i get what i have to do in theory, its how i would do it in java using recursive method that i cant get my head around.
I fixed it to this, but still getting stackoverflow error:
code: |
public static int exponential (int a, int a2, int n){
int exp = 0;
int result=0;
if (exp < n) {
result = a * a2;
exp++;
exponential (a, result, n);
}
return result;
}
|
|
|
|
|
|
 |
Dreadnought
|
Posted: Fri Nov 29, 2013 11:46 pm Post subject: Re: recursive method help |
|
|
Krocker wrote: ok, at the when the method repeats when it calls itself, will it also do the initialization of the 1st two variable? if so, then how would i initialize them without them resetting? \
You can keep a value through the recursive call by changing the values you pass as arguments to the recursive call. (in this case a, a2 and n)
It looks like your are trying to have your exponential function call itself recursively until exp is as big as n. In this way exp tells you how far into the calculation you are but, like you said, exp is reset every time you call the function.
What you need to do is find a way of changing your arguments (a, a2 and n) when you make the recursive call. (hint: What could you do to n?)
Also, you probably want to do something with the value your function returns after you call it recursively. Right now you don't do anything with the result of your recursion. (hint: Does your function return something different on each recursive call?) |
|
|
|
|
 |
Tony
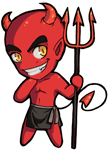
|
Posted: Sat Nov 30, 2013 12:22 am Post subject: RE:recursive method help |
|
|
As Dreadnought pointed out, this is still iterative way of thinking about the problem.
You can reshape your iterative solution to look like recursion (by doing tricks with the counters, passing intermediate values into next call, etc.), but that's no the recursive way of thinking about the problem.
Once again
Quote:
assuming that you already (somehow) know what the value of 2^10 is, how would you calculate the value of 2^11
The key ideas of recursion is that you only need to solve two small steps about any problem:
1. the trivial case (a^0 == 1)
2. how to sole the current problem, if a solution to a smaller problem is given to you (if you know the answer to a^n-1, how do you solve for a^n ?)
Once the two pieces are in place, you keep on recursively calling on step 2 until you get down to the base case. At this point you can fulfill the assumption of knowing a smaller answer (from that one simple answer that you know), and if everything is setup right, the solution falls into place. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Krocker
|
Posted: Sat Nov 30, 2013 10:07 am Post subject: RE:recursive method help |
|
|
I cant believe it was that simple! Thanks Tony and Dreadnought, so all i had to do was an if statment for if n<=0, return 1 else return a*exponetinal (a, n-1). |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|