Author |
Message |
Krocker
|
Posted: Sun Oct 20, 2013 5:13 pm Post subject: odd indices of an array |
|
|
hi, so i need to output the odd number indices of an array without using any if statments. i know that odd = 2n+1 where n is the an integer starting at 0. my trouble is getting the for loop im using to stop when all the odd indices are outputed. So far, all i get it to is before the last index or beyond the last index, giving me an out of bound error. This is my for loop so far:
code: |
System.out.println ("\n\nValues at odd-numbered indices:");
int odd = 2;
int shiftedOddArray = 0;
for (int i = 0; i < (odd-1); i++){
odd = (2*i)+1;
shiftedOddArray = shiftedArray[odd];
System.out.print ( shiftedOddArray + "\t");
}
|
shiftedArray is the original array that i have to output every odd index. The number of indices and the numbers in the indices are all determined by the user at the begining of the program. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Raknarg

|
Posted: Sun Oct 20, 2013 7:47 pm Post subject: RE:odd indices of an array |
|
|
You don't have to use i++. THat's just a shortcut for saying add one to i. You can do whatever you want in there (I'm pretty sure) |
|
|
|
|
 |
Tony
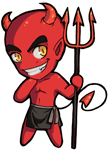
|
Posted: Sun Oct 20, 2013 8:48 pm Post subject: RE:odd indices of an array |
|
|
anything you want, or even nothing.
is a valid loop. At least for C, probably Java. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Krocker
|
Posted: Sun Oct 20, 2013 9:42 pm Post subject: RE:odd indices of an array |
|
|
but how would that stop when the program has gone through the number of odd indices that are possiible according tho the user specified number of indices? |
|
|
|
|
 |
Insectoid

|
Posted: Sun Oct 20, 2013 10:13 pm Post subject: RE:odd indices of an array |
|
|
How would you do it if you wanted to run through all of the indices of the array up to the user specified limit? |
|
|
|
|
 |
Raknarg

|
Posted: Mon Oct 21, 2013 8:05 am Post subject: RE:odd indices of an array |
|
|
@Tony is that a loop that does nothing or loops infinitely? |
|
|
|
|
 |
Insectoid

|
Posted: Mon Oct 21, 2013 8:14 am Post subject: RE:odd indices of an array |
|
|
The 2nd argument to a for loop is the exit condition. If there is no exit condition, what do you think will happen? |
|
|
|
|
 |
Krocker
|
Posted: Mon Oct 21, 2013 8:36 am Post subject: RE:odd indices of an array |
|
|
if there isnt an exit condition, there will be an infinite loop, thats not what im looking for. Anyways, i figured it out, i simply used a while loop and said that while (odd< numberOfArray) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
C14
|
Posted: Tue Oct 22, 2013 1:04 pm Post subject: Re: RE:odd indices of an array |
|
|
Krocker @ Mon Oct 21, 2013 8:36 am wrote: Anyways, i figured it out, i simply used a while loop and said that while (odd< numberOfArray)
Can we see your code? |
|
|
|
|
 |
Krocker
|
Posted: Fri Oct 25, 2013 8:28 am Post subject: Re: odd indices of an array |
|
|
Heres the code that i used:
code: |
System.out.println ("\n\nValues at odd-numbered indices:");
int odd = 1; //declare and initializes variables to be used
int shiftedOddArray = 0;
int n = 0;
//does through each odd indices and outputs the value in order of the shifted array by calling shiftedArray [odd] where odd is 2n+1
while (odd <= numberOfArray-1){
shiftedOddArray = shiftedArray[odd];
System.out.print ( shiftedOddArray + "\t");
n++;
odd = (2*n)+1;
}
|
|
|
|
|
|
 |
|