JPanel hurts my brain
Author |
Message |
Tallguy

|
Posted: Thu Oct 04, 2012 5:02 pm Post subject: JPanel hurts my brain |
|
|
Greetings All!!!
I am once again back into java Here is my dilemma i am using the GUI's in JPanel (ONLY) to create a GUI for a calculator. I just can not for the life of me get it to appear on my screen, the array holds the String values to be used in GridLayout. i am only able to BorderLayout || FlowLayout || GridLayout. I can do this in about 10 min using JFrame, but we are not allowed to touch it.
Here is the code that matters
Any help would be awesome
code: |
import java.awt.*;
import javax.swing.*;
public class CalculatorView extends JPanel {
private static final long serialVersionUID = 1L;
public CalculatorView() {
}
public void addButton() {
JPanel content = new JPanel(new BorderLayout());
int width = 256;
int height = 256;
Dimension screen = Toolkit.getDefaultToolkit().getScreenSize();
int x = (screen.width-width)/2;
int y = (screen.height-height)/2;
setBounds(x,y,width,height);
JPanel buttons = new JPanel();
buttons.setLayout(new GridLayout(4,5,5,10));
content.setBorder(BorderFactory.createEmptyBorder (0,2,5,2));
String [] values = { "7", "8", "9","/", "P","4","5","6","*","\u221A","1","2","3","-","C","0",".","+/-","+","="};
for (int i=0; i<values.length ; i++){
buttons.add(new Button(values[i]));
System.out.print (values[i]);
}
content.add(buttons,BorderLayout.SOUTH);
content.setVisible(true);
}
}
|
MY MAIN
code: |
import java.awt.EventQueue;
public class Calculator {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run (){
System.out.print ("3\n");
CalculatorView calcview = new CalculatorView ();
calcview.addButton();
calcview.setVisible(true);
}
});
}
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Thu Oct 04, 2012 6:09 pm Post subject: RE:JPanel hurts my brain |
|
|
I've never been able to get any of Java's gui elements to do what I want. If you were me you'd give up now, but you're Tallguy, not me, so don't give up.
Then again, I am six & a half feet tall, so maybe you are me. |
|
|
|
|
 |
Tony
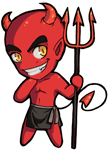
|
Posted: Thu Oct 04, 2012 7:49 pm Post subject: Re: JPanel hurts my brain |
|
|
Doesn't answer your question, but... are you using Java 5+ ? for-each loops are your friend
Tallguy @ Thu Oct 04, 2012 5:02 pm wrote:
String [] values = { "7", "8", "9","/", "P","4","5","6","*","\u221A","1","2","3","-","C","0",".","+/-","+","="};
for (int i=0; i<values.length ; i++){
buttons.add(new Button(values[i]));
System.out.print (values[i]);
}
vs.
code: |
String [] values = {...} // same
for (String value : values ) {
buttons.add(new Button(value));
}
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DemonWasp
|
Posted: Thu Oct 04, 2012 8:08 pm Post subject: RE:JPanel hurts my brain |
|
|
JPanels represent "panel" components, not "frame" components: that is, they don't represent windows on their own. You need to add them to a JFrame (or other container) which is being drawn to have the panel be drawn. See: http://docs.oracle.com/javase/tutorial/uiswing/components/toplevel.html
I don't understand how you're supposed to complete this assignment "without touching JFrame".
Edit: You could use another top-level container, such as JDialog or JApplet. |
|
|
|
|
 |
TokenHerbz

|
Posted: Thu Oct 04, 2012 9:46 pm Post subject: RE:JPanel hurts my brain |
|
|
yeah JApplet would, but i find with that the complier (i suppose depending which you use) wont detect bugs that are found compiling to JFrame.. so i would use JFrame and then change it to JApplet. |
|
|
|
|
 |
DemonWasp
|
Posted: Thu Oct 04, 2012 10:16 pm Post subject: RE:JPanel hurts my brain |
|
|
I'm not sure what you mean by that sentence. If your compiler isn't finding compile-time problems, then it's not compliant with the Java Language Specification, which means it is not a Java compiler. Which compiler were you having trouble with (and give an example of the code in question)? |
|
|
|
|
 |
Tallguy

|
Posted: Thu Oct 11, 2012 9:29 am Post subject: Re: JPanel hurts my brain |
|
|
Thank you all - I have got it to work. Note that this assignment is just the GUI aspect, once i get the application working I'll post in submissions. Here is the fix, my main was able to use JFrame, just none of the contents
Main
Java: |
/*
* File name: Calculator.java
* Author: Tallguy
* Professor:-----
* Purpose: The driver class for my Swing GUI, responsible for running everythings. Calls the splash screen
* and creates an intial frame(JFrame) in which to hold the contents (buttons, text field etc)
* of all aspects of the calculator to be created.
* Class list: []
*/
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Toolkit;
import javax.swing.JFrame;
/************************************************
@author Tallguy
@version 1
@see [javax.swing.*, java.awt*]
@since java 1.6
************************************************/
public class Calculator {
public static void main (String[] args ) {
/************************************************
{@value} WIDTH - constant var for the screen width
{@value} HEIGHT - constant var for the screen height
************************************************/
final int WIDTH = 256;
final int HEIGHT = 256;
EventQueue. invokeLater(new Runnable() {
/************************************************
Creates the initial JFrame settings its values, such as height/width and resizable
factors. It thens adds contents to the frame
@param []
@return [ ]
************************************************/
public void run () {
CalculatorView calcview = new CalculatorView ();
JFrame frame = new JFrame("Calculator"); /*creates a new JFrame object*/
frame. setResizable(false);
frame. setDefaultCloseOperation(JFrame. EXIT_ON_CLOSE); /*overrides default close oepration (close window) to kill process*/
int width = WIDTH;
int height = HEIGHT;
Dimension screen = Toolkit. getDefaultToolkit(). getScreenSize();
int x = (screen. width - width ) / 2;
int y = (screen. height - height ) / 2;
frame. setBounds(x, y, width, height ); /*sets screen size*/
frame. add(calcview ); /*adds contents to the JFrame*/
frame. setVisible(true); /*makes the screen visible*/
}
});
}
}
|
CalculatorView
Java: |
/*
* File name: CalculatorView.java
* Author: Tallguy
*/
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
import javax.swing.border.Border;
public class CalculatorView extends JPanel {
private static final long serialVersionUID = 1L;
private JTextField display;
private JLabel error;
private JButton dotButton, backspace, btn;
private JPanel viewPanel;
private JRadioButton radioInt, radioFl;
private ButtonGroup Rbgroup /* = new ButtonGroup()*/;
public CalculatorView () {
this. setLayout(new BorderLayout());
this. setBorder(BorderFactory. createEmptyBorder(0, 2, 5, 2));
Controller handler = new Controller ();
/* sets look and feel to work on both Win and MacOS */
try {
UIManager. setLookAndFeel(UIManager
. getCrossPlatformLookAndFeelClassName());
} catch (Exception e ) {
e. printStackTrace();
}
/* sets look and feel to work on both Win and MacOS - END */
/* radio buttons */
JPanel Rbutton = new JPanel(new FlowLayout());
Border outer = BorderFactory. createEmptyBorder(0, 15, 3, 15);
Border inner = BorderFactory. createLineBorder(Color. BLUE, 4);
Rbutton. setBorder(BorderFactory. createCompoundBorder(outer, inner ));
Rbgroup = new ButtonGroup();;
Rbutton. setFocusable(false);
/* radio buttons - END */
/* north layer parts */
/* backspace button */
backspace = new JButton("<<");
backspace. setPreferredSize(new Dimension(20, 20));
backspace. setBorder(BorderFactory. createEmptyBorder());
backspace. setOpaque(false);
backspace. setToolTipText("Backspace (Alt-B)");
backspace. setContentAreaFilled(false);
backspace. setBorderPainted(false);
backspace. setMnemonic('B');
backspace. setFocusPainted(false);
backspace. addActionListener(handler );
/* backspace button - END */
/* text field */
display = new JTextField("0.0", 15);
display. setHorizontalAlignment(JTextField. RIGHT);
display. setBackground(Color. WHITE);
display. setEditable(false);
/* text field - END */
/* error field */
error = new JLabel(" ");
error. setPreferredSize(new Dimension(20, 20));
error. setBorder(BorderFactory. createEmptyBorder());
error. setOpaque(true);
error. setBackground(Color. GREEN);
error. setToolTipText("Error");
/* error field - END */
/* north layer parts - END */
/* holds all north end parts */
viewPanel = new JPanel(new FlowLayout());
viewPanel. add(error, BorderLayout. WEST);
viewPanel. add(display, BorderLayout. NORTH);
viewPanel. add(backspace, BorderLayout. EAST);
/* holds all north end parts - END */
/* calc buttons */
JPanel buttons = new JPanel(new GridLayout(4, 5, 5, 5));
String[] values = { "7", "8", "9", "/", "P", "4", "5", "6", "*",
"\u221A", "1", "2", "3", "-", "C", "0", ".", "+/-", "+", "=" };
for (String value : values ) {
addButton (buttons, value, handler );
}
String[] radio = { "Integer", "Float" };
for (String value : radio ) {
addButton (Rbutton, value, handler );
}
/* calc buttons - END */
/* adds all to master panel */
this. add(viewPanel, BorderLayout. NORTH);
this. add(Rbutton, BorderLayout. CENTER);
this. add(buttons, BorderLayout. SOUTH);
/* adds all to master panel - END */
}
private void addButton (Container c, String s, ActionListener handler ) {
if (s == "Integer") {
radioInt = new JRadioButton("Integer", false);
radioInt. setFont(new Font("Serif", Font. BOLD, 12));
radioInt. setBackground(Color. yellow);
radioInt. addActionListener(handler );
radioInt. setFocusPainted(false);
Rbgroup. add(radioInt );
c. add(radioInt );
} else if (s == "Float") {
radioFl = new JRadioButton("Float", true);
radioFl. setFont(new Font("Serif", Font. BOLD, 12));
radioFl. setBackground(Color. PINK);
radioFl. addActionListener(handler );
radioFl. setFocusPainted(false);
Rbgroup. add(radioFl );
c. add(radioFl );
} else {
btn = new JButton(s );
btn. setPreferredSize(new Dimension(30, 33));
btn. setForeground(Color. BLUE);
btn. setFont(new Font("Serif", Font. BOLD, 12));
if (s == "+/-") {
btn. setFont(new Font("Serif", Font. BOLD, 10));
}
if (s == ".") {
dotButton = btn;
}
if (s == "C") {
btn. setBackground(Color. RED);
btn. setForeground(Color. black);
}
if (s == "=") {
btn. setBackground(Color. yellow);
btn. setForeground(Color. black);
}
btn. setFocusPainted(false);
btn. addActionListener(handler );
c. add(btn );
}
}
private class Controller implements ActionListener {
public void actionPerformed (ActionEvent e ) {
String digit = e. getActionCommand();
display. setText(digit );
//display.setText(display.getText() + digit);
}
}
}
|
@Tony - You are awesome good sir, I always forget about the for-each loops
Tony wrote:
Doesn't answer your question, but... are you using Java 5+ ? for-each loops are your friend
Insectoid wrote:
I've never been able to get any of Java's gui elements to do what I want. If you were me you'd give up now, but you're Tallguy, not me, so don't give up.
Then again, I am six & a half feet tall, so maybe you are me.
-only 6'4"
EDIT-Added syntax |
|
|
|
|
 |
QuantumPhysics
|
Posted: Mon Oct 15, 2012 9:55 pm Post subject: RE:JPanel hurts my brain |
|
|
Its not JPanel, its just java overall. Why not use a Applet or Jython wx package. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tallguy

|
Posted: Wed Oct 17, 2012 10:32 am Post subject: RE:JPanel hurts my brain |
|
|
assignment specifications |
|
|
|
|
 |
QuantumPhysics
|
Posted: Fri Oct 19, 2012 12:11 am Post subject: RE:JPanel hurts my brain |
|
|
Oh. Fair Enough. |
|
|
|
|
 |
|
|