String library last letter not = new letter ?
Author |
Message |
QuantumPhysics
|
Posted: Thu Aug 23, 2012 10:25 pm Post subject: String library last letter not = new letter ? |
|
|
I am practicing the string library here is my code what i am attempting:
Cplusplus: |
#include <iostream>
#include <string>
#define MAX 1000
using namespace std;
void getLastLetter () {
for (int i = 0; i <= MAX; ++i){
loop:
string theWord,newWord;
cout << "Please enter the word: ";
getline (cin,theWord);
cout << "Now you must enter a word starting with letter: " << theWord[4];
getline (cin,newWord);
if (theWord[4] != newWord[0]) goto loop;
}
}
int main (){
getLastLetter();
return 1;
}
|
Im trying to make it so the user inputs a word. Then they must input a new word with the last character of the previous word. Im not sure exactly how to do this, i tried to only work with 4 letter words and when i got the output for running that program the last character of the word was the square root sign and a N with a sqwiggly line on top? Easter egg? Anyways please help me out here. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
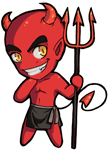
|
|
|
|
 |
QuantumPhysics
|
Posted: Thu Aug 30, 2012 12:19 pm Post subject: RE:String library last letter not = new letter ? |
|
|
Thanks tony, but what i came across doing was something like this:
lastchar = word[word.length()-1]
To get the last character of the word. Thanks a lot though. |
|
|
|
|
 |
Tony
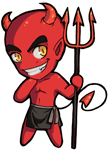
|
Posted: Thu Aug 30, 2012 6:46 pm Post subject: RE:String library last letter not = new letter ? |
|
|
yup, that's exactly how it should be. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
wtd
|
Posted: Tue Sep 04, 2012 1:58 am Post subject: RE:String library last letter not = new letter ? |
|
|
Make it a function.
code: | char lastLetterOfString(string inputString)
{
return inputString[inputString.length() - 1];
} |
|
|
|
|
|
 |
bl0ckeduser
|
Posted: Tue Sep 04, 2012 1:24 pm Post subject: Re: String library last letter not = new letter ? |
|
|
QuantumPhysics @ Thu Aug 23, 2012 10:25 pm wrote: Cplusplus: |
loop:
[...]
if (theWord[4] != newWord[0]) goto loop;
|
I think that is exactly the same as a do-while loop, which some
will find more readable.
wtd wrote: code: | char lastLetterOfString(string inputString)
{
return inputString[inputString.length() - 1];
} |
I would recommend checking that inputString.length() > 0,
just in case. String indexing crashes aren't fun.
Here's my quick and dirty C code for OP's problem, which is quite similar
to wtd's C++'s code.
code: |
int beginning_is_end(char* s)
{
return strlen(s) && *s == s[strlen(s) - 1];
}
|
I hope my comments are helpful.
Happy programming. |
|
|
|
|
 |
[Gandalf]

|
Posted: Tue Sep 04, 2012 9:55 pm Post subject: RE:String library last letter not = new letter ? |
|
|
Don't ever use goto in C++, especially not for loops. What you're looking for is a do-while loop, like bl0ckeduser said.
You probably want to return 0, which is a successful exit, and not 1.
What happens if theWord is shorter than 4 characters? |
|
|
|
|
 |
QuantumPhysics
|
Posted: Wed Sep 05, 2012 12:18 pm Post subject: RE:String library last letter not = new letter ? |
|
|
Actually, i always return true; from now on, and if you looked above you would see that I already have the answer. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
md

|
Posted: Wed Sep 05, 2012 12:30 pm Post subject: Re: RE:String library last letter not = new letter ? |
|
|
Gandalf @ 2012-09-04, 9:55 pm wrote: Don't ever use goto in C++, especially not for loops. What you're looking for is a do-while loop, like bl0ckeduser said.
You probably want to return 0, which is a successful exit, and not 1.
What happens if theWord is shorter than 4 characters?
Goto is great for error handling in some cases, expecially when interacting directly with the kernel or when using system calls which don't already have nice exception handling rules. |
|
|
|
|
 |
[Gandalf]

|
Posted: Wed Sep 05, 2012 5:24 pm Post subject: Re: RE:String library last letter not = new letter ? |
|
|
QuantumPhysics @ 2012-09-05, 12:18 pm wrote: Actually, i always return true; from now on, and if you looked above you would see that I already have the answer.
Where do you return true? In the code you posted you return 1 from int main(), which signifies a failure. Returning true from main() doesn't make sense... bool main() isn't a valid signature for main in standard C++, and returning true from int main() is very suspect since it relies on implicit type conversion and even then yields a return code of 1.
I commented on a few things in your code which should be clear for any C++ programmer from the start. C++ has a very steep learning curve from the very beginning.
md wrote:
Gandalf @ 2012-09-04, 9:55 pm wrote: Don't ever use goto in C++, especially not for loops. What you're looking for is a do-while loop, like bl0ckeduser said.
You probably want to return 0, which is a successful exit, and not 1.
What happens if theWord is shorter than 4 characters?
Goto is great for error handling in some cases, expecially when interacting directly with the kernel or when using system calls which don't already have nice exception handling rules.
This is why I said especially not for loops, but even then I would hardly call that great. It's a hack in lieu of better exception handling mechanisms when interfacing with C or other legacy code. |
|
|
|
|
 |
|
|