How to: Base 64 Encrypting/Decrypting
Author |
Message |
randint
|
Posted: Fri Feb 03, 2012 6:39 pm Post subject: How to: Base 64 Encrypting/Decrypting |
|
|
This is a very simple (but insecure) way to encrypt and/or decrypt a String using Base64
1. Make sure you have the package "org.apache.commons.codec.binary.*" installed in your system, if not, download it from http://commons.apache.org/codec/download_codec.cgi and unzip the folder, extract it anywhere you want, then somehow utilize them (usually adding them to the build path, in the case of Eclipse, go to the toolbar, click on Project->Properties->Java Build Path->Libraries->Add External JARs, then browse to the JARs you downloaded, and then import org.apache.commons.codec.binary.* to use this feature)
Java: |
import org.apache.commons.codec.binary.*;
import java.io.*;
public class BASE64ENCODEDECODE
{
public static void main (String args [])
{
try
{
BufferedReader br = new BufferedReader (new InputStreamReader (System. in));
//Encrypt a String
System. out. print ("Enter a String: ");
String original = br. readLine ();
String encrypt = new String (Base64. encodeBase64 (original. getBytes ("UTF-8")));
System. out. println ("The encrypted String is " + encrypt );
//Decrypt a String
System. out. print ("Enter an encrypted String: ");
String encrypted = br. readLine ();
String decrypt = new String (Base64. decodeBase64 (encrypted. getBytes ("UTF-8")));
System. out. println ("The decrypted String is " + decrypt );
}
catch (Throwable t )
{
System. out. println ("Exception or error " + t + " has occured!");
}
}
}
|
Hopefully this works for you! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
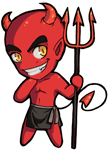
|
Posted: Fri Feb 03, 2012 6:57 pm Post subject: RE:How to: Base 64 Encrypting/Decrypting |
|
|
as the class name suggests, Base64 is an encoding method (not encyrption). Suggesting otherwise is misleading.
Also, you're catching Throwable, which will attempt to catch more than just Exceptions. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TokenHerbz

|
Posted: Sat Mar 17, 2012 9:22 pm Post subject: RE:How to: Base 64 Encrypting/Decrypting |
|
|
i thought to catch an exception you must have that at the class declaration...
EX:
code: |
public static void main (String args[]) throws IOException {
}
|
please let me know if im mistaken and why / how it all really works (the exceptions).
EDIT:
I also just wanted to comment on the naming conventions used about, i Dislike how the class was named, I think something more suitable would be, "Base_64_EncodeDecode"
I guess i want to share my opinion with other ones to while I'm on this case....
code: |
String sStringVar = "string;
int iNumber = 76;
boolean bAnswerIsTrue = false;
double dTotalAmount;"
int[][] aGrid = new int[5][5];
Scanner scanUserInput = new Scanner;
|
just for a few, do you guys think its necessary or not?.. if you can see how the variables are defined... |
|
|
|
|
 |
DemonWasp
|
Posted: Sat Mar 17, 2012 10:26 pm Post subject: RE:How to: Base 64 Encrypting/Decrypting |
|
|
Token:
1. You only declare 'throws' for exceptions you actually throw. His main() method doesn't throw anything of any kind, so it doesn't list a 'throws' clause. You'll notice he has catch ( Throwable t ), which catches the root exception-type class, Throwable. Exception extends Throwable. This will catch ANYTHING that gets thrown, including things you're not generally supposed to catch, like OutOfMemoryError or StackOverflowError. Since no exception can ever "escape" main(), main() doesn't throw anything.
2. Java has standards and naming conventions. If you aren't following them, you are probably wrong. Class names should be NamedLikeThis and should be a noun. This class could reasonably be called "Base64EncoderMain" or "Base64EncoderRuntime" or similar. You are correct that all-caps is a poor decision, though.
3. Hungarian naming conventions ('sStringVar', 'iNumber', see: http://en.wikipedia.org/wiki/Hungarian_notation ) went out of style around when we invented IDEs. I haven't seen anyone use them in code written since about 1990, so please don't do so yourself. Variable names should be nouns and should correspond to how they will be used locally. |
|
|
|
|
 |
|
|