Author |
Message |
nelsonkkyy
|
Posted: Thu Oct 20, 2011 2:44 pm Post subject: for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
Quote:
/**
Adds up the values from 1 to 10000, but skipping certain ones
*/
public class Adder2
{
public static void main(String[] args)
{
//----------------------Start here. Do not remove this comment.
// todo: define a local integer constant called LIMIT equal to 10000
// approximate lines of code required: 1
int total = 0;
//----------------------End here. Do not remove this comment.
int sum = 0 ;
int sumNo5s = 0 ;
int sumOf5s = 0 ;
//----------------------Start here. Do not remove this comment.
// todo: 1. write a for-loop that adds values from 1 to LIMIT, storing the total in sum; 2. at the same time, do the summation but skipping values which have a 5 anywhere in the number, such as 5 15, 251, 5552, storing this result in sumNo5s; 3. and at the same time add up the numbers you are skipping in to the variable sumOf5s.
// approximate lines of code required: 8
for (total = 0; total <= 10000; total++)
{
sum = total + sum;
}
//----------------------End here. Do not remove this comment.
System.out.println("Sum = " + sum ) ;
System.out.println("SumNo5s = " + sumNo5s ) ;
System.out.println("SumOf5s = " + sumOf5s ) ;
System.out.println("Expected: ") ;
System.out.println("Sum = " + 50005000) ;
System.out.println("SumNo5s = 32406760" ) ;
System.out.println("SumOf5s = 17598240" ) ;
}
}
What kind of methods can i use to get rid of the 5 in all the values? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
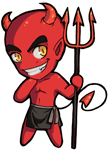
|
Posted: Thu Oct 20, 2011 3:00 pm Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
follow an example
does this value have a digit 5 in it? How did you know? Do the same thing for every number. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
nelsonkkyy
|
Posted: Thu Oct 20, 2011 6:29 pm Post subject: Re: for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
it has a 5 in it but i dun know how to detect the 5, like by using what method? |
|
|
|
|
 |
crossley7
|
Posted: Thu Oct 20, 2011 6:33 pm Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
modular functions and division.
In turing notation with the most basic example. I don't know the specific java equivalent but my guess is that % is mod and / is div provided the 123 is stored in an integer.
123 div 100 = 1
123 div 10 mod 10 = 2
123 mod 10 = 3 |
|
|
|
|
 |
Tony
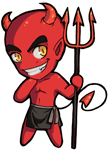
|
Posted: Thu Oct 20, 2011 6:41 pm Post subject: Re: for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
nelsonkkyy @ Thu Oct 20, 2011 6:29 pm wrote: it has a 5 in it but i dun know how to detect the 5
but
Tony @ Thu Oct 20, 2011 3:00 pm wrote: How did you know?
You looked at each digit, one at a time, to see if any of them were 5. Do that. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
nelsonkkyy
|
Posted: Thu Oct 20, 2011 6:46 pm Post subject: Re: for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
obviously,, but i couldnt find any command to do that. |
|
|
|
|
 |
Insectoid

|
Posted: Thu Oct 20, 2011 7:54 pm Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
There is no command to do that. There's a lot of things you might have to do for which there is no command. That's likely the objective of the assignment- find a way to identify each digit in a number. Big hint: You can do this on paper, with math. |
|
|
|
|
 |
Tony
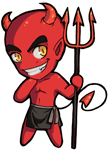
|
Posted: Thu Oct 20, 2011 8:40 pm Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
Hey guys, I want to build a search engine to find web pages, like Google does. What's the command in Java to do that?  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Nick

|
Posted: Fri Oct 21, 2011 7:15 am Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
in this case you're looking at the number like a sentence, checking for a specific letter. The program is looking at it like a number. Make the program look at it like a sentence. There was this one variable type that did that... what was it? |
|
|
|
|
 |
md

|
Posted: Fri Oct 21, 2011 9:58 am Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
Actually, looking at it like a number turns out to be much faster and easier - plus you can use some simple logic to skip ahead to the next possible non-5 number to check.
The trick to solving the problem is to figure out how to do it by hand. Once you can describe the solution you can trivially write the Java code (in this case, not all cases are trivial). |
|
|
|
|
 |
mirhagk
|
Posted: Fri Oct 21, 2011 4:38 pm Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
depends on the library but converting the value to a string and calling an index of method on it is trivial, and the speed of the program is not important in this case. |
|
|
|
|
 |
Tony
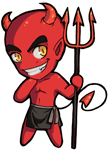
|
Posted: Fri Oct 21, 2011 5:52 pm Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
trivial solutions are rarely the point of assignments. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Velocity

|
Posted: Fri Feb 10, 2012 10:58 am Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
if numvalue mod 5 = 1 or 2 or 3 * .75 / 500 + 2.25 * 10 ^ -4 it will have a number with 5 in it |
|
|
|
|
 |
RandomLetters
|
Posted: Fri Feb 10, 2012 8:41 pm Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
There is a command for that, the good old
(""+n).indexOf('5') >= 0 |
|
|
|
|
 |
Velocity

|
Posted: Mon Feb 13, 2012 12:16 pm Post subject: RE:for loop add 1 to 10000 but skip all the values that have 5 in it. |
|
|
or that... lol thats easier to get and comprehend. |
|
|
|
|
 |
|