A competition using goto
Author |
Message |
Dratino
|
Posted: Sun Apr 17, 2011 8:43 pm Post subject: A competition using goto |
|
|
Here's a little something I came up with just to be evil to structured programmers:
Devise a function a(m, n) to output a single integer, that follows the following restrictions:
1. The function must use a return variable, initialized at 0, and you may only have one statement that increments it.
2. The function may not define any other variables.
3. You may only use the functions and statements ++, --, ==, if, and goto. (If coding in a language other than C or C++, the equivalents may be used. ++ means "increase by 1", and -- means "decrease by 1".)
4. You may not have the same statement twice within the same block of code. (So, no using {++n; ++n;})
Make a function that will return the largest value for a(42, 42). My record is 904, and the source code for the solution is below:
code: | long long a(int m, int n){
long long output = 0;
stop_a:
++output;
stop_b:
--n;
if(n == 0){
--m;
goto stop_c;
}
goto stop_a;
stop_c:
if(m < 0) goto stop_d;
++n;
if(n < m) goto stop_c;
goto stop_a;
stop_d:
return output;
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Sun Apr 17, 2011 8:48 pm Post subject: RE:A competition using goto |
|
|
Um, what exactly does a(m, n) do? You seem to have forgotten to tell us that. |
|
|
|
|
 |
Dratino
|
Posted: Sun Apr 17, 2011 8:55 pm Post subject: RE:A competition using goto |
|
|
a(m, n) can do whatever you want it to, as long as it follows those rules.
It returns a single integer. |
|
|
|
|
 |
Tony
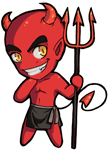
|
Posted: Sun Apr 17, 2011 9:10 pm Post subject: RE:A competition using goto |
|
|
Your rules don't mention anything regarding the use of integer literals; and your code does use them ( == 0), so... goto loops until 905? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
A.J

|
Posted: Sun Apr 17, 2011 9:11 pm Post subject: Re: A competition using goto |
|
|
Perhaps I am misunderstanding your rules:
c++: |
#include<iostream>
using namespace std;
#define arbitrary 0x7FFFFFFF
long long a(int m, int n)
{
unsigned long long ret = 0;
a:;
ret++;
if (ret==arbitrary) return ret;
goto a;
}
main()
{
cout << a(42, 42) << endl;
}
|
EDIT: I apologize Tony, as I posted my code right when you mentioned that. Coincidence. |
|
|
|
|
 |
Dan
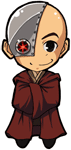
|
Posted: Sun Apr 17, 2011 9:41 pm Post subject: RE:A competition using goto |
|
|
The contest is rather silly. Can i just have LONG_MAX number of "++"s hardcoded into my code?
Also some overflow fun:
c: |
unsigned long long a(int m, int n){
unsigned long long output = 0;
return --output;
}
|
|
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
RandomLetters
|
|
|
|
 |
Dratino
|
Posted: Mon Apr 18, 2011 10:56 am Post subject: Re: RE:A competition using goto |
|
|
Tony @ Sun Apr 17, 2011 9:10 pm wrote: Your rules don't mention anything regarding the use of integer literals; and your code does use them ( == 0), so... goto loops until 905?
It actually doesn't.
Um. I'm going to have to modify that rule, then - another reason I put it on here is so that I could iron out the loopholes in the contest, because not just for having bugs in the program, but having bugs in the contest rules.
"No integer literals besides 0 may be used."
Dan @ Sun Apr 17, 2011 9:41 pm wrote: The contest is rather silly. Can i just have LONG_MAX number of "++"s hardcoded into my code?
Try not to. That would probably violate rule 4.
The point is to create a function that loops through a complicated system of goto's to simulate some sort of recursion. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dan
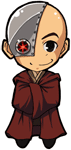
|
Posted: Mon Apr 18, 2011 3:43 pm Post subject: RE:A competition using goto |
|
|
Did you look at my underflow soultion? It seems to meet all the rules. Uses a single return varaible, only statment is a single --. No goto used but your rules don't say it has to be. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
A.J

|
Posted: Mon Apr 18, 2011 9:39 pm Post subject: RE:A competition using goto |
|
|
I don't believe my solution violates any rules either. |
|
|
|
|
 |
btiffin

|
Posted: Mon Apr 18, 2011 11:02 pm Post subject: Re: A competition using goto |
|
|
Dratino;
You want to google brainf#*k. It's a swear word name, fill in the #*, for a programming engine you may like.
It be funky in it's completeness.
Cheers |
|
|
|
|
 |
Dratino
|
Posted: Tue Apr 19, 2011 10:54 pm Post subject: Re: RE:A competition using goto |
|
|
Dan @ Mon Apr 18, 2011 3:43 pm wrote: Did you look at my underflow soultion? It seems to meet all the rules. Uses a single return varaible, only statment is a single --. No goto used but your rules don't say it has to be.
Yeah, I guess. Like I said, one point of this was to iron out the loopholes. Your underflow glitch would be one of those.
@btiffin: Yeah, I've heard of Brainfuck. |
|
|
|
|
 |
|
|