Sorting Integers in a comma delimited text file
Author |
Message |
revangrey

|
Posted: Mon Apr 11, 2011 1:47 pm Post subject: Sorting Integers in a comma delimited text file |
|
|
1. "Write a Class called IntegerSorter which contains a list of integers and a method for sorting
this list."
When writing this class, I am expected to implement an interface called Sorter. I pasted it below in the code tags.
2. "In order to test your code, you will need to write a simple program to generate random
integers and store them in a comma delimited text file. Additionally, you will need to write
some code to read the text file that you create." (going to try this later today)
My goal in this assignment is to sort a list in increasing order, by comparing the first two numbers in a list and swapping them if the first number is bigger than the second number.
Every time the sorter goes through the list of numbers, it will start after the previous starting point, until the numbers are sorted in order increasing from top to bottom.
My question is: how do I swap these numbers and make sure that the sorter starts right after the previous starting point?
Should I use one of the sort methods...? (it is at the bottom under "fill")
If you were wondering, I am very lost... and any help is appreciated!
code: | public interface Sorter {
/**
* This method sets the current list that is used in this class. The original list should never be changed.
* @param list - a reference to the list of elements to be sorted.
*/
public void setList(int[] list);
/**
* This methods sorts the list in increasing order.
* @param type - parameter to be used to choose an internal sorting method.
* If type = 1 use sorting method 1
* If type = 2 use sorting method 2
* etc....
*/
public void sort(int type);
/**
* This method prints out the current state of the list
* @return a String representing the list, either sorted or not depending on whether sort() was previously called.
* The format of the String is: num1, num2, num3, ...
*/
public String toString();
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
apython1992

|
Posted: Mon Apr 11, 2011 2:21 pm Post subject: RE:Sorting Integers in a comma delimited text file |
|
|
I'm not sure about using a built-in sort method - if this is for a school assignment (which it looks like), then chances are your prof/teacher is expecting you to come up with your own algorithm. Or maybe not.
With your method (bubble sort), you want to keep going through the list and swapping until you don't need to swap any more (that is, you pass through the entire list without making a single swap). I don't know if Java has a swap method or not. But if not, you can use a temporary variable.
code: |
int a = 3;
int b = 7;
int temp;
// Perform swap
temp = a;
a = b;
b = temp;
|
As for your question regarding the previous starting point - I'm not sure I understand what you mean by that. I'm gonna guess that you're trying to evaluate your list after every pass on a larger scale to determine where to start your next pass. With a bubble sort though, this wouldn't be worth anything, because checking where to start next requires the same amount of operations as passing through the list from the beginning again. It would just be redundant and really only add overhead. |
|
|
|
|
 |
revangrey

|
Posted: Mon Apr 11, 2011 6:37 pm Post subject: Re: RE:Sorting Integers in a comma delimited text file |
|
|
apython1992 @ Mon Apr 11, 2011 2:21 pm wrote: I'm not sure about using a built-in sort method - if this is for a school assignment (which it looks like), then chances are your prof/teacher is expecting you to come up with your own algorithm. Or maybe not.
Good point...
apython1992 @ Mon Apr 11, 2011 2:21 pm wrote:
As for your question regarding the previous starting point - I'm not sure I understand what you mean by that. I'm gonna guess that you're trying to evaluate your list after every pass on a larger scale to determine where to start your next pass. With a bubble sort though, this wouldn't be worth anything, because checking where to start next requires the same amount of operations as passing through the list from the beginning again. It would just be redundant and really only add overhead.
I think I was mistaken when I was explaining the method with which I am required to sort. I am supposed to continue swapping groups of two numbers if the top number is greater than the bottom number, but the program is not supposed to attempt a swap of the final number from the previous loop.
4| 2.............. |2| 2
2| 4.............. |4| 4
14| 14............ |9| 9
9| 9.............. |5| 5
5| 5.............. |7| 7
7| 7.............. |1| 1
1| 1.............. |14| 10
10| 10 ............ |10| 14
Above are examples of the first and last swap of the numbers, with the "..." indicating the steps I omitted, and the "|" being placed to separate the numbers for the sake of neatness. Not to imply absolute value or something...
The first column contains the numbers before sorting, the second column the numbers after the first swap, the second last column the numbers before the last swap, and the last column contains the numbers after one loop of swapping.
Please excuse the layman's terms... |
|
|
|
|
 |
apython1992

|
Posted: Mon Apr 11, 2011 7:11 pm Post subject: RE:Sorting Integers in a comma delimited text file |
|
|
This is effectively a bubble sort. Though I'm still not quite sure I understand where you're having your difficulty...are you trying to prevent your algorithm from swapping the last element from the previous loop with the first element in the next loop? |
|
|
|
|
 |
revangrey

|
Posted: Wed Apr 13, 2011 1:49 pm Post subject: Re: RE:Sorting Integers in a comma delimited text file |
|
|
Two days later, and I can read and write to the text file... Thanks for the reply
The program has to be able to read and write to the file, as well as sort the integers from the file. (a .txt file)
apython1992 @ Mon Apr 11, 2011 7:11 pm wrote: ...are you trying to prevent your algorithm from swapping the last element from the previous loop with the first element in the next loop?
Right now I am just trying to understand how the IntegerSorter itself would work. For example, the program would have to read the randomly generated integers from the text file (I can do this), put them in an array(?)/take them one at a time from the text file(?), sort them until they are all sorted (somehow the program has to know when to stop), and output the sorted integers. Once the integers have been generated, each integer takes up one line in the text file. So if the program takes the numbers from the text file line by line, it will still be taking them one at a time.
My main problem would be how to make the program stop as soon as the Integers are sorted, because I honestly have no ideas of how to accomplish that as of yet...
Does the following idea for swapping the integers make sense? I think it is basically the same thing as what you mentioned before...
if int A > int B then swap int A and B
next line rinse and repeat
int a;
int b;
int temp;
if temp > b
then b = a;
and a = b;
Apologies for any spelling and grammar mistakes, I'm pretty tired...
Any and all help appreciated! |
|
|
|
|
 |
apython1992

|
Posted: Wed Apr 13, 2011 1:57 pm Post subject: RE:Sorting Integers in a comma delimited text file |
|
|
As far as knowing when to stop goes...you would have to pass through the entire array without making a single swap. This could be as easy as having a boolean variable, like swap_occurred that is always set to false at the beginning of each pass through the list. If you have to swap, that value should be set to true. Keep making passes through the list as long as swap_occurred = true by the end of the pass through the array. |
|
|
|
|
 |
Tony
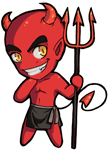
|
|
|
|
 |
revangrey

|
Posted: Wed Apr 13, 2011 3:02 pm Post subject: Re: RE:Sorting Integers in a comma delimited text file |
|
|
Tony @ Wed Apr 13, 2011 2:12 pm wrote: An important distinction here is where the numbers are stored -- in memory or on disk.
code: |
if temp > b
then b = a;
and a = b;
|
in a = b step, what is the value of b?
Once you figure this out, maybe you can also help SapphireBlue? http://compsci.ca/v3/viewtopic.php?p=234425
if a>b then
temp = b;
b = a;
a = temp;
That should make more sense...
apython1992 @ Wed Apr 13, 2011 1:57 pm wrote: As far as knowing when to stop goes...you would have to pass through the entire array without making a single swap. This could be as easy as having a boolean variable, like swap_occurred that is always set to false at the beginning of each pass through the list. If you have to swap, that value should be set to true. Keep making passes through the list as long as swap_occurred = true by the end of the pass through the array.
Thanks! I'll get right to it... |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|