Calling a Method with Multiple Parameters
Author |
Message |
huskiesgoaler34

|
Posted: Tue Apr 05, 2011 12:32 pm Post subject: Calling a Method with Multiple Parameters |
|
|
So I am creating a breakfast menu program and I am having trouble calling one of my methods. The method I am calling calculates the total cost of the food chosen. Now, I have created other methods in order to calculate the cost of the total amount of cereals, egg, toast,etc purchased. Now, I was successful in passing the variables to my total cost method but when I go to call it, I don't know the correct way to call a method with multiple parameters. Can you please help. Thanks!
code: |
public static void finalPayment (double cerealSubTotal, double eggSubTotal,
double meatSubTotal, double toastSubTotal,
double sideDishSubTotal, double drinkSubTotal )
{
Scanner input = new Scanner (System.in);
double totalCostBeforeTax;
double taxes;
double totalCost;
System.out.println ("");
System.out.println ("It is time to pay for your chosen dishes.");
System.out.println ("");
System.out.println ("Your totals come out to the following:");
System.out.println ("");
System.out.println ("Cereals: $" + cerealSubTotal);
System.out.println ("Egg Dishes: $" + eggSubTotal);
System.out.println ("Meat Dishes: $" + meatSubTotal);
System.out.println ("Toasts: $" + toastSubTotal);
System.out.println ("Side Dishes: $" + sideDishSubTotal);
System.out.println ("Drinks: $" + drinkSubTotal);
System.out.println ("");
System.out.println ("Your total comes out to");
totalCostBeforeTax = cerealSubTotal + eggSubTotal + meatSubTotal + toastSubTotal + sideDishSubTotal + drinkSubTotal;
taxes= totalCostBeforeTax * 0.13;
totalCost = totalCostBeforeTax + taxes;
}
switch (menuOption) {
case 1: cereals(); break;
case 2: eggDishes(); break;
case 3: meatDishes(); break;
case 4: toast(); break;
case 5: sideDishes(); break;
case 6: drinks(); break;
case 7: finalPayment(); break; //WHAT DO I PUT IN THE BRACKETS??????????????????????
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
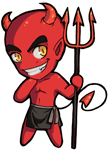
|
Posted: Tue Apr 05, 2011 12:51 pm Post subject: RE:Calling a Method with Multiple Parameters |
|
|
all 6 arguments described in
code: |
finalPayment (double cerealSubTotal, double eggSubTotal,
double meatSubTotal, double toastSubTotal,
double sideDishSubTotal, double drinkSubTotal )
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
huskiesgoaler34

|
Posted: Tue Apr 05, 2011 12:58 pm Post subject: Re: Calling a Method with Multiple Parameters |
|
|
I put all the variable names inside the bracket put it comes up as a syntax error:
code: |
case 7: finalPayment (cerealSubTotal,eggSubTotal, meatSubTotal, toastSubTotal, sideDishSubTotal, drinkSubTotal ); break;
|
|
|
|
|
|
 |
Tony
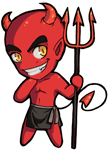
|
Posted: Tue Apr 05, 2011 1:04 pm Post subject: RE:Calling a Method with Multiple Parameters |
|
|
What is the syntax error? (And what is your interpretation of what that error means?) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DemonWasp
|
Posted: Tue Apr 05, 2011 1:05 pm Post subject: RE:Calling a Method with Multiple Parameters |
|
|
Well, did you declare the variables cerealSubTotal, eggSubTotal, ... , drinkSubTotal? Did you fill them with sensible values? |
|
|
|
|
 |
huskiesgoaler34

|
Posted: Tue Apr 05, 2011 1:07 pm Post subject: Re: Calling a Method with Multiple Parameters |
|
|
File: D:\CJsBreakfastMenu.java [line: 626]
Error: D:\CJsBreakfastMenu.java:626: cannot find symbol
symbol : variable cerealSubTotal
location: class CJsBreakfastMenu
Now, it appears to me that the complier can not find the variables. (I got 6 errors, all on the same line, one for each variable)
I am puzzled as to why this happens because I already passed the parameters to the finalPayment method. |
|
|
|
|
 |
huskiesgoaler34

|
Posted: Tue Apr 05, 2011 1:09 pm Post subject: Re: Calling a Method with Multiple Parameters |
|
|
All the values for each variable are delcared in previous methods. All of them have values. I passed these variables to my method and now when I go call the method, I can't seem to figure out why it doesn't work. |
|
|
|
|
 |
Tony
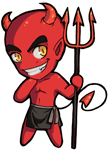
|
Posted: Tue Apr 05, 2011 1:25 pm Post subject: RE:Calling a Method with Multiple Parameters |
|
|
It's a matter of scope. A local variable declared inside of a method is visible only to that method. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
SmokeMonster
|
Posted: Wed Apr 06, 2011 2:58 am Post subject: Re: Calling a Method with Multiple Parameters |
|
|
Sounds like the methods that holds your switch statements does not have access to the variables. You need to pass the variables to the the method that has the switch block or make the scope of the variables global within your class. They should be private and global anyways within your BreakfastMenu class. I assume you have all the code in your main class since I see not calls to instances of a specific class? Just pull their declaration out of a specific function and make them global. A safer way would be to spilt the breakfastmenu and its operations into a separate class and use mutators to modify the data instead of just allowing main class unrestricted access. |
|
|
|
|
 |
|
|