Help with conversion to lower case
Author |
Message |
Colliflower
|
Posted: Wed Nov 02, 2011 8:57 pm Post subject: Help with conversion to lower case |
|
|
I am trying to convert the letters of a string to lower case using a for loop and the tolower command, but i am getting an error...
#include <iostream>
#include <ctype.h>
#include <string>
using namespace std;
int main () {
std::string sDecision, sWord;
cout << "Hello! Would you like to move your letter up, or down?";
cin >> sDecision;
sWord= "";
for (int num = 0; num < sDecision.size(); num++) {
//Error occurs saying cannot convert from char to const char
sWord.append(char(tolower(sDecision[num])));
}
cout << sWord << "\n";
system("PAUSE");
return 0;
}
I have tried multiple things, but i cannot get this to work please help |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
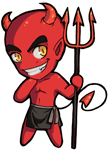
|
Posted: Wed Nov 02, 2011 10:06 pm Post subject: Re: Help with conversion to lower case |
|
|
Colliflower @ Wed Nov 02, 2011 8:57 pm wrote: I have tried multiple things
what kind of things have you tried? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Colliflower
|
Posted: Wed Nov 02, 2011 10:16 pm Post subject: Re: Help with conversion to lower case |
|
|
at first i was appending the letters by calling them strings but my teacher said i should try using characters instead, I was mainly just trying whatever came to mind but the period ran out before i could figure out the problem and continue the question (I am kinda new to c++ so i am not entirely sure what I can and cannot do...) |
|
|
|
|
 |
Tony
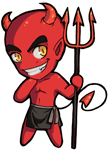
|
Posted: Wed Nov 02, 2011 10:48 pm Post subject: Re: Help with conversion to lower case |
|
|
Colliflower @ Wed Nov 02, 2011 8:57 pm wrote: //Error occurs saying cannot convert from char to const char
well char and const char are different types... |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
S_Grimm

|
Posted: Thu Nov 03, 2011 9:08 am Post subject: Re: Help with conversion to lower case |
|
|
http://www.cplusplus.com/reference/clibrary/cctype/tolower/
give that function a try.
Remember that strings are just arrays of characters and can be accessed just like an array.
edit: try going through each character in the string and calling to lower, then passing it into an output string. |
|
|
|
|
 |
Colliflower
|
Posted: Thu Nov 03, 2011 4:14 pm Post subject: Re: Help with conversion to lower case |
|
|
S_Grimm @ Thu Nov 03, 2011 9:08 am wrote: http://www.cplusplus.com/reference/clibrary/cctype/tolower/
give that function a try.
Remember that strings are just arrays of characters and can be accessed just like an array.
edit: try going through each character in the string and calling to lower, then passing it into an output string.
That is what i am trying to do, I am going through each character of the string sDecision and use the tolower command on each letter and then append it to the sWord string. I don't know what I am doing wrong though...
Tony @ Wed Nov 02, 2011 10:48 pm wrote:
well char and const char are different types...
I am not sure where i am using the const char though, I know what a const char is, I just don't see where I am using one... |
|
|
|
|
 |
Tony
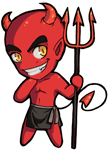
|
Posted: Thu Nov 03, 2011 5:25 pm Post subject: RE:Help with conversion to lower case |
|
|
check your function signatures, one is probably expecting a different type than you are providing.
...
Also, the actual error is different from what you posted:
Quote:
test.cc:12: error: invalid conversion from ?char? to ?const char*?
test.cc:12: error: initializing argument 1 of ?std::basic_string<_CharT, _Traits, _Alloc>& std::basic_string<_CharT, _Traits, _Alloc>::append(const _CharT*) [with _CharT = char, _Traits = std::char_traits<char>, _Alloc = std::allocator<char>]?
Emphasis mine. The problem is that string.append is expecting const char* (not const char as you've said), while you are giving it just char. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Colliflower
|
Posted: Thu Nov 03, 2011 6:03 pm Post subject: Re: Help with conversion to lower case |
|
|
Thanks Tony i had to change a lot of things because I got new errors every time I tried fixing the old ones
I ended up with this as a fix
Quote: #include <iostream>
#include <ctype.h>
#include <string>
using namespace std;
int main () {
string sDecision;
int iSize;
cout << "Hello! Would you like to move your letter up, or down?";
cin >> sDecision;
iSize = sDecision.size();
for (int num = 0; num < iSize; num++) {
sDecision[num] = ((tolower(sDecision[num])));
}
cout << sDecision << "\n";
system("PAUSE");
return 0;
}
Thanks again for helping out the noobie! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|