Author |
Message |
unoho

|
Posted: Wed Jan 19, 2011 5:55 pm Post subject: structure and pointers help |
|
|
okay ..so im new to C++. i do have 4 months of experience with C..
so the problem is everytime i try to run it, it outputs error saying the program has stopped working..windows is looking for solution.
any idea what i could be doing wrong?
code: |
#include <iostream>
#include <stdlib.h>
#include <assert.h>
#include <string>
using namespace std;
struct myStruct{
int val;
myStruct *next;
};
void print(myStruct *p1, myStruct *p2){
assert (p1 && p2);
if(p1->val < p2->val){
cout << p1->val << endl;
cout << p2->val << endl;
}else{
cout << p1->val << endl;
cout << p2->val << endl;
}
return;
}
int main(int argc, char * argv[]){
myStruct *p1;
myStruct *p2;
p1->val = 45;
p2->val = 60;
print(p1, p2);
return 0;
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
chrisbrown

|
Posted: Wed Jan 19, 2011 6:24 pm Post subject: RE:structure and pointers help |
|
|
Neither p1 or p2 point to anything meaningful. Declaring a pointer does not automatically create the object to be pointed at.
Declare p1 and p2 as objects instead of pointers (i.e. without *), and pass their addresses to print. |
|
|
|
|
 |
TerranceN
|
Posted: Wed Jan 19, 2011 6:32 pm Post subject: RE:structure and pointers help |
|
|
I believe this is relevant. |
|
|
|
|
 |
unoho

|
Posted: Wed Jan 19, 2011 7:24 pm Post subject: RE:structure and pointers help |
|
|
although i already saw the binky video, thanks anyways..
and @ chrisbrown, it worked..thnk u as well |
|
|
|
|
 |
Tony
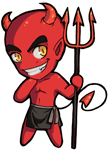
|
Posted: Wed Jan 19, 2011 7:32 pm Post subject: Re: RE:structure and pointers help |
|
|
chrisbrown @ Wed Jan 19, 2011 6:24 pm wrote: Declare p1 and p2 as objects instead of pointers (i.e. without *), and pass their addresses to print.
Ah, but that depends on where in memory you want those objects to be. As above, they'll be on the stack.. which works in this particular case of them being at the very bottom. Should he allocate objects on the stack that goes out of scope (such as inside a function that returns), it will probably result in errors. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
unoho

|
Posted: Fri Jan 21, 2011 10:20 pm Post subject: RE:structure and pointers help |
|
|
quick question...on my void print function im supposed to check too see if the parameters are NULL or not.. i was just wondering is that right way to check?
void print(myStruct *p1, myStruct *p2){
assert (p1 && p2);
} |
|
|
|
|
 |
chrisbrown

|
Posted: Fri Jan 21, 2011 11:15 pm Post subject: RE:structure and pointers help |
|
|
Ask yourself: what happens to the execution of the program if either argument is NULL?
Now implement the check using an if/else statement and ask the same question.
Which one seems safer?
Which is more expandable? |
|
|
|
|
 |
Xupicor
|
Posted: Tue Feb 22, 2011 6:59 pm Post subject: Re: structure and pointers help |
|
|
Do you even need pointers there? If not, use references:
c++: | struct Foo {
int a;
}
int bar(const Foo& foo) { // you can be sure that foo is a valid object
return foo.a
}
int main() {
Foo foo;
int a = bar(foo);
return 0;
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
unoho

|
Posted: Wed Feb 23, 2011 12:00 am Post subject: RE:structure and pointers help |
|
|
oh at tht moment, we didn't learn reference.. but ya i guess i could use reference now for that if i had to do it again. |
|
|
|
|
 |
|