Spaces in strings - how?
Author |
Message |
Srlancelot39

|
Posted: Wed Feb 09, 2011 12:49 am Post subject: Spaces in strings - how? |
|
|
I am making a program that may result in the user entering a string containing spaces, and I need the program to accept the entire input (including spaces and characters after a space) and store it in the variable. Currently the program is carrying over words after a space into the next get.
Example (pseudocode and output):
get var1
(input: word1 word2)
get var2 - this ends up taking word2 as its value
print var1,var2
output: word1,word2
I know this example looks like crap, but basically, the program is carrying over the input. How do I get it so that var1 is holding "word1 word2"?
Thank you! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
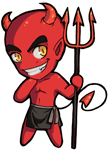
|
|
|
|
 |
Srlancelot39

|
Posted: Wed Feb 09, 2011 1:19 am Post subject: RE:Spaces in strings - how? |
|
|
do i have to use 'str' or is that where my variable name goes? |
|
|
|
|
 |
Srlancelot39

|
Posted: Wed Feb 09, 2011 1:23 am Post subject: Re: Spaces in strings - how? |
|
|
// Assignment1 2.13.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include <string>
#include <string.h>
#include "iostream"
#include "conio.h"
#include <fstream>
using namespace std;
int main()
{
string driveletter;
char pathname[256];
string filename;
string extension;
cout << "Welcome to FileMaster!" << endl;
cout << "Please enter the drive letter (i.e. 'C'): ";
cin >> driveletter;
cout << "Please enter the path name (i.e. \\Windows\\System): ";
cin.get (pathname,256);
cout << "Please enter the file name (i.e. Readme): ";
cin >> filename;
cout << "Please enter the file extension (i.e. txt): ";
cin >> extension;
cout << driveletter << ":" << pathname << "\\" << filename << "." << extension << endl;
getch();
return 0;
}
now its ignoring the other cin statements after i enter a value for the first one....lol |
|
|
|
|
 |
Xupicor
|
Posted: Tue Feb 22, 2011 5:58 pm Post subject: RE:Spaces in strings - how? |
|
|
Why not make use of std::string:
c++: | #include <iostream>
#include <string>
int main() {
using namespace std;
string s;
getline(cin, s); // reads up to (including) \n, and discards \n char.
cout << s << '\n';
return 0;
} |
|
|
|
|
|
 |
|
|