Translating an english sentence to spanish using a given database
Author |
Message |
G-nerd
|
Posted: Wed Nov 24, 2010 6:46 am Post subject: Translating an english sentence to spanish using a given database |
|
|
Hi I need help trying to translate an English sentence to Spanish using a database given and also making the user continue to ask an English sentence until asked to be terminated using a given character.
Here is my code
code: |
import string
db = [['dog', 'perro'], ['man', 'hombre'], ['woman', 'mujer'], ['house', 'casa'], ['eat',
'comer'], ['speak', 'hablar'], ['walk', 'caminar'], ['black', 'negro'], ['white', 'blanco'],
['large', 'grande'], ['fat', 'gordo'], ['skinny', 'flaca'], ['street', 'calle'], ['from', 'de'],
['to', 'a'], ['in', 'en'], ['the', 'el']]
def toSpanish2(x):
for i in range(len(string.split(x)): # for all the elements in x after converting the string to lists
for j in range(len(db)): # for all the elements in db
if string.split(x)[i]==db[j]: # if any element of x is equivalent to any element in the database
if db[j][0]==string.split(x)[i]: # if the 0th element = and element of i
return db[j][1] # return the 1st element in the list of db
if x == "null": # if x equals null
Break #then terminate the program
return string.join(x) #if one of the conditions are met, return the string that was converted into list form back into regular string form
x=raw_input("Please type an English Sentence:") # Make user ask for english sentence to be converted
|
When I run the code on python it first asks me to type in a sentence, which I do, but using strings so that in can be converted to a list. However, once the english sentence has been implemented, nothing comes out. Can someone show me what the error is.
My friend has a different code but I can't understand it. He says it works but not properly. I don't understand how he came up with the x.split().
code: |
import string
x=raw_input(" Please type an English sentence: ")
db = [['dog', 'perro'], ['man', 'hombre'], ['woman', 'mujer'], ['house', 'casa'], ['eat',
'comer'], ['speak', 'hablar'], ['walk', 'caminar'], ['black', 'negro'], ['white', 'blanco'],
['large', 'grande'], ['fat', 'gordo'], ['skinny', 'flaca'], ['street', 'calle'], ['from', 'de'],
['to', 'a'], ['in', 'en'], ['the', 'el']]
def toSpanish2(x):
sum=[]
for i in range (len(x.split())):
for j in range(len(db)):
if (x.split())[i] in db[j][0]:
db[j][0]=db[j][1]
sum.append(db[j][0])
return " ".join(sum)
|
when he types : " the fat man fat"
it just produces el gordo hombre
it should say el gordo hombre gordo
Please any help would be gladly appreciated. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
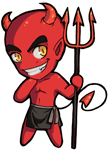
|
Posted: Thu Nov 25, 2010 12:59 am Post subject: RE:Translating an english sentence to spanish using a given database |
|
|
> Can someone show me what the error is.
If the Python interpreter does not give you warnings/errors, then there isn't one and the code is doing exactly what you told it to do. That might be different from what you thought about though Try to figure out what your code is doing and how it's different from what you thought it was doing. A common technique is to use print statements to output program's state as it runs, and comparing that against your expectations.
> I don't understand how he came up with the x.split().
x is an argument which receives a string object. String objects have a number of methods defined, including #split()
http://docs.python.org/library/stdtypes.html#str.split |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
kctan
|
Posted: Tue Nov 30, 2010 12:39 am Post subject: Re: Translating an english sentence to spanish using a given database |
|
|
Make some small change to your friend's code. If you need more explanation, let me know.
code: | import string
x=raw_input(" Please type an English sentence: ")
db = [['dog', 'perro'], ['man', 'hombre'], ['woman', 'mujer'], ['house', 'casa'], ['eat',
'comer'], ['speak', 'hablar'], ['walk', 'caminar'], ['black', 'negro'], ['white', 'blanco'],
['large', 'grande'], ['fat', 'gordo'], ['skinny', 'flaca'], ['street', 'calle'], ['from', 'de'],
['to', 'a'], ['in', 'en'], ['the', 'el']]
def toSpanish2(x):
sum=[]
for i in range (len(x.split())):
for j in range(len(db)):
if (x.split())[i] in db[j][0]:
sum.append(db[j][1])
break
return " ".join(sum)
print toSpanish2(x) |
|
|
|
|
|
 |
wtd
|
Posted: Tue Nov 30, 2010 1:44 am Post subject: Re: Translating an english sentence to spanish using a given database |
|
|
First off, let's convert your db to a dictionary.
code: | db = [['dog', 'perro'], ['man', 'hombre'], ['woman', 'mujer'], ['house', 'casa'], ['eat',
'comer'], ['speak', 'hablar'], ['walk', 'caminar'], ['black', 'negro'], ['white', 'blanco'],
['large', 'grande'], ['fat', 'gordo'], ['skinny', 'flaca'], ['street', 'calle'], ['from', 'de'],
['to', 'a'], ['in', 'en'], ['the', 'el']]
words = dict(db) |
Now, let's get a list of the words in an input sentence:
code: | sentence_words = sentence.split(" ") |
Now, let's get a new list of translated words.
code: | sentence_words_translated = [words[w] for w in sentence_words] |
Now, let's use a generator expression passed to join to get the new sentence.
code: | new_sentence = " ".join(words[w] for w in sentence_words) |
Isn't that much nicer than this explicit looping and list of lists malarky? |
|
|
|
|
 |
kctan
|
Posted: Tue Nov 30, 2010 3:32 am Post subject: Re: Translating an english sentence to spanish using a given database |
|
|
wtd @ Tue Nov 30, 2010 2:44 pm wrote:
Isn't that much nicer than this explicit looping and list of lists malarky?
I think it depends. For someone who is not familiar with list comprehension, your solution may not be easy to understand. |
|
|
|
|
 |
wtd
|
Posted: Tue Nov 30, 2010 11:00 am Post subject: RE:Translating an english sentence to spanish using a given database |
|
|
Then that person would be well-advised to learn about list comprehensions and generators.
Python is not Tring with different syntax, after all. |
|
|
|
|
 |
|
|