My First GUI Program
Author |
Message |
rar

|
Posted: Mon May 31, 2010 11:11 am Post subject: My First GUI Program |
|
|
Hello
I'm working with Dev-C++ and I'm trying to create a program in C with a GUI. Being as I've never done this before, I start with their pre-made Windows application so that I can start with an idea of what code needs to be present for a program to have a GUI. Here is the code they give:
code: |
#include <windows.h>
/* Declare Windows procedure */
LRESULT CALLBACK WindowProcedure (HWND, UINT, WPARAM, LPARAM);
/* Make the class name into a global variable */
char szClassName[ ] = "WindowsApp";
int WINAPI WinMain (HINSTANCE hThisInstance,
HINSTANCE hPrevInstance,
LPSTR lpszArgument,
int nFunsterStil)
{
HWND hwnd; /* This is the handle for our window */
MSG messages; /* Here messages to the application are saved */
WNDCLASSEX wincl; /* Data structure for the windowclass */
/* The Window structure */
wincl.hInstance = hThisInstance;
wincl.lpszClassName = szClassName;
wincl.lpfnWndProc = WindowProcedure; /* This function is called by windows */
wincl.style = CS_DBLCLKS; /* Catch double-clicks */
wincl.cbSize = sizeof (WNDCLASSEX);
/* Use default icon and mouse-pointer */
wincl.hIcon = LoadIcon (NULL, IDI_APPLICATION);
wincl.hIconSm = LoadIcon (NULL, IDI_APPLICATION);
wincl.hCursor = LoadCursor (NULL, IDC_ARROW);
wincl.lpszMenuName = NULL; /* No menu */
wincl.cbClsExtra = 0; /* No extra bytes after the window class */
wincl.cbWndExtra = 0; /* structure or the window instance */
/* Use Windows's default color as the background of the window */
wincl.hbrBackground = (HBRUSH) COLOR_BACKGROUND;
/* Register the window class, and if it fails quit the program */
if (!RegisterClassEx (&wincl))
return 0;
/* The class is registered, let's create the program*/
hwnd = CreateWindowEx (
0, /* Extended possibilites for variation */
szClassName, /* Classname */
"Windows App title bar message/title", /* Title Text */
WS_OVERLAPPEDWINDOW, /* default window */
CW_USEDEFAULT, /* Windows decides the position */
CW_USEDEFAULT, /* where the window ends up on the screen */
500, /* The programs width */
500, /* and height in pixels */
HWND_DESKTOP, /* The window is a child-window to desktop */
NULL, /* No menu */
hThisInstance, /* Program Instance handler */
NULL /* No Window Creation data */
);
/* Make the window visible on the screen */
ShowWindow (hwnd, nFunsterStil);
/* Run the message loop. It will run until GetMessage() returns 0 */
while (GetMessage (&messages, NULL, 0, 0))
{
/* Translate virtual-key messages into character messages */
TranslateMessage(&messages);
/* Send message to WindowProcedure */
DispatchMessage(&messages);
}
/* The program return-value is 0 - The value that PostQuitMessage() gave */
return messages.wParam;
}
/* This function is called by the Windows function DispatchMessage() */
LRESULT CALLBACK WindowProcedure (HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
switch (message) /* handle the messages */
{
case WM_DESTROY:
PostQuitMessage (0); /* send a WM_QUIT to the message queue */
break;
default: /* for messages that we don't deal with */
return DefWindowProc (hwnd, message, wParam, lParam);
}
return 0;
}
|
My first question, although probably not at all important at this stage, is
How do you enable a File menu (meaning the menu at the top that has File, Edit, Help, etc.)? I see code that says:
code: |
NULL, /* No menu */
|
Based on the comment, I'm assuming that NULL can be replaced in order to enable a menu, somehow. I'm just not sure what to replace it with.
This will be the best way for me to learn in the time that I have (being as I will soon be required to make a program with a GUI by a side job I'm working at) and I would like to learn from the code already given from Dev. So if anyone could help me out, it'd be much appreciated!! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
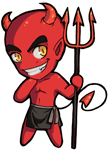
|
Posted: Mon May 31, 2010 11:44 am Post subject: RE:My First GUI Program |
|
|
Look up the .h file that has CreateWindowEx defined. Those have documentation... sometimes. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
rar

|
Posted: Mon May 31, 2010 11:51 am Post subject: RE:My First GUI Program |
|
|
This is what I found for windows.h in the files that came with Dev.
code: |
/*
windows.h - main header file for the Win32 API
Written by Anders Norlander <anorland@hem2.passagen.se>
This file is part of a free library for the Win32 API.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
*/
#ifndef _WINDOWS_H
#define _WINDOWS_H
#if __GNUC__ >=3
#pragma GCC system_header
#endif
/* translate GCC target defines to MS equivalents. Keep this synchronized
with winnt.h. */
#if defined(__i686__) && !defined(_M_IX86)
#define _M_IX86 600
#elif defined(__i586__) && !defined(_M_IX86)
#define _M_IX86 500
#elif defined(__i486__) && !defined(_M_IX86)
#define _M_IX86 400
#elif defined(__i386__) && !defined(_M_IX86)
#define _M_IX86 300
#endif
#if defined(_M_IX86) && !defined(_X86_)
#define _X86_
#elif defined(_M_ALPHA) && !defined(_ALPHA_)
#define _ALPHA_
#elif defined(_M_PPC) && !defined(_PPC_)
#define _PPC_
#elif defined(_M_MRX000) && !defined(_MIPS_)
#define _MIPS_
#elif defined(_M_M68K) && !defined(_68K_)
#define _68K_
#endif
#ifdef RC_INVOKED
/* winresrc.h includes the necessary headers */
#include <winresrc.h>
#else
#include <stdarg.h>
#include <windef.h>
#include <wincon.h>
#include <winbase.h>
#if !(defined NOGDI || defined _WINGDI_H)
#include <wingdi.h>
#endif
#ifndef _WINUSER_H
#include <winuser.h>
#endif
#ifndef _WINNLS_H
#include <winnls.h>
#endif
#ifndef _WINVER_H
#include <winver.h>
#endif
#ifndef _WINNETWK_H
#include <winnetwk.h>
#endif
#ifndef _WINREG_H
#include <winreg.h>
#endif
#ifndef _WINSVC_H
#include <winsvc.h>
#endif
#ifndef WIN32_LEAN_AND_MEAN
#include <cderr.h>
#include <dde.h>
#include <ddeml.h>
#include <dlgs.h>
#include <imm.h>
#include <lzexpand.h>
#include <mmsystem.h>
#include <nb30.h>
#include <rpc.h>
#include <shellapi.h>
#include <winperf.h>
#ifndef NOGDI
#include <commdlg.h>
#include <winspool.h>
#endif
#if defined(Win32_Winsock)
#warning "The Win32_Winsock macro name is deprecated.\
Please use __USE_W32_SOCKETS instead"
#ifndef __USE_W32_SOCKETS
#define __USE_W32_SOCKETS
#endif
#endif
#if defined(__USE_W32_SOCKETS) || !(defined(__CYGWIN__) || defined(__MSYS__) || defined(_UWIN))
#if (_WIN32_WINNT >= 0x0400)
#include <winsock2.h>
/*
* MS likes to include mswsock.h here as well,
* but that can cause undefined symbols if
* winsock2.h is included before windows.h
*/
#else
#include <winsock.h>
#endif /* (_WIN32_WINNT >= 0x0400) */
#endif
#ifndef NOGDI
#if !defined (__OBJC__)
#if (__GNUC__ >= 3) || defined (__WATCOMC__)
#include <ole2.h>
#endif
#endif /* __OBJC__ */
#endif
#endif /* WIN32_LEAN_AND_MEAN */
#endif /* RC_INVOKED */
#ifdef __OBJC__
/* FIXME: Not undefining BOOL here causes all BOOLs to be WINBOOL (int),
but undefining it causes trouble as well if a file is included after
windows.h
*/
#undef BOOL
#endif
#endif
|
|
|
|
|
|
 |
rar

|
|
|
|
 |
BigBear
|
Posted: Mon May 31, 2010 1:46 pm Post subject: RE:My First GUI Program |
|
|
Since your using dev C++ and want to start learning graphics I would suggest going to
Tools -> Check for Updates/Packages...
Then select devpacks.org and Check for Updates
then select Allegro and install it.
Once it is installed go to new -> Project then select the multimedia tab and allegro.
and you can start using the allegro graphics library
documentation found here
http://www.allegro.cc/manual/ |
|
|
|
|
 |
TerranceN
|
Posted: Mon May 31, 2010 2:44 pm Post subject: RE:My First GUI Program |
|
|
I agree with BigBear, use Allegro or SDL or SOMETHING other than the Win32 API if you can. Win32 is pointlessly confusing, but if you insist here is a tutorial on adding menus. |
|
|
|
|
 |
|
|