creating enums in the function scope, possible in any language?
Author |
Message |
mccarre
|
Posted: Mon Mar 15, 2010 1:17 am Post subject: creating enums in the function scope, possible in any language? |
|
|
This comes to me as I would like to have significantly nicer code for a graph I'm coding in C#.
Everything is based off strings, and it is state driven, but checking states is a pain.
Currently this is what I have:
code: |
//create nodes
bnet.addNode("clouds", new[] { "clearSky", "partiallyCloudy", "cloudy" });
bnet.addNode("precipitation", new[] { "noPrecepitation", "raining", "snowing", "hailing" });
//create an edge, and add general probabilities. clouds effect on precipitation. {no-prec, rain, snow, hail}
bnet.addEdge("clouds", "precipitation")
.addGeneralProbability("clearSky", new[] { 0.85, 0.05, 0.05, 0.05 })
.addGeneralProbability("partiallyCloudy", new[] { 0.4, 0.2, 0.2, 0.2 })
.addGeneralProbability("cloudy", new[] { 0.1, 0.3, 0.3, 0.3 });
//checking if in state "raining" (ObservedValue is stored as a uint, like an enum would)
bool isRaining = bnet["precipitation"].ObservedValue == bnet["precipitation"].States.IndexOf("raining")
//if state was stored a a string instead of a uint [like a struct]
bool isRaining = bnet["precipitation"].ObservedValue == "raining"
|
I would like to be able to do this with something like enums. Now as nice as having a solution for C# would be, I'm curious if any language can do this nicely. When I say any language that basically means C++0x.
Here's an example of what i'd like to be able to do:
code: |
//create nodes
bnet.addNode("clouds", new enum { "clearSky", "partiallyCloudy", "cloudy" });
bnet.addNode("precipitation", new enum { "noPrecepitation", "raining", "snowing", "hailing" });
//create an edge, and add general probabilities.
bnet.addEdge("clouds", "precipitation")
.addGeneralProbability(bnet["clouds"].states.clearSky, new[] { 0.85, 0.05, 0.05, 0.05 });
//checking if in state "raining" (ObservedValue is stored as a uint, like an enum would)
bool isRaining = bnet["precipitation"].ObservedValue == bnet["precipitation"].raining;
|
This would potentially be checked at compile time, making it much easier to debug, and faster to run, plus in IDEs I could use intellisense stuff.
So my first problem/challenge is for a person to be able to create enums inside a function scope (the enum would still be static as enums are).
---
Further if there was a way to have the nodes added be part of an enum somehow, that'd be cool, but that seems much more difficult, because there is a node associated, not just a uint.
So my second challenge would be to have compile time bindings of objects to other objects. This one seems very difficult, like you would need to prepare an object at the beginning of runtime according to the compilation, with the bindings, then clone from that object any new objects you make. What i said was theoretical... I doubt a language could do it, but if anyone has any ideas, I'm curious to hear them. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
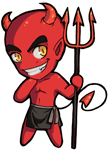
|
Posted: Mon Mar 15, 2010 3:05 am Post subject: Re: creating enums in the function scope, possible in any language? |
|
|
mccarre @ Mon Mar 15, 2010 1:17 am wrote: it is state driven, but checking states is a pain.
This is why one studies http://en.wikipedia.org/wiki/State_machine in CS University. There's very likely a library for such in "every language", but the subject is definitely worth studying. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
mccarre
|
Posted: Fri Jun 11, 2010 5:02 pm Post subject: Re: creating enums in the function scope, possible in any language? |
|
|
Necro post!!!
I've been busy with a lot of other things, and the project that this post related to has been on the back-burner for a while.
I used poor wording in my post. I said the first challenge would be to create an enum in the function scope. I meant create an enum dynamically, or something like it.
I know this is entirely possible in javascript, because objects are basically associative arrays, so I could create an object and then add each state as a member variable, and then set them to true and false as necessary. During development I would add defensive measures such as constantly checking to ensure only one value is true, however production I would ignore this for speed.
Still I think it would be possible to do this in C++ as well using some template tricks... if I had time to burn I'd try and figure it out... well maybe eventually, but the challenge is still on the table.
(Tony I know pretty well how state machines work... my code is not a state-machine, because a state-machine (at least finite) can not accomplish what I need. What I am using is just a labeled graph. Having states does not automatically make something a finite state machine. Well... according to the loose definition of a state machine it would be an NFA, but calling it that would give a person no information to how any of my code runs =P [every state can go to every state... useless def'n]) |
|
|
|
|
 |
2goto1

|
Posted: Sat Jun 19, 2010 9:22 pm Post subject: Re: creating enums in the function scope, possible in any language? |
|
|
Hi mccarre, this is an interesting subject to me. Btw, what does "Necro post" mean? Is that another term for "bump"?
Regarding your question, from another perspective is it effectively an anonymous type that you're trying to create, in that within your function/method body you would create the new enum type, and perhaps return that to your function/method's callers? Is that the intent you're aiming for?
If so, perhaps through the use of reflection it may be possible. I do know that in addition the C# 4.0 specification has new built-in support for dynamic types. It effectively allows you to create anonymous types and pass them around and use them outside of the scope in which they were created. See http://blog.codinglight.com/2009/05/c-40-dynamic-type-something-useful.html for an example. On the C++ side - not fully sure but that might be one direction worth looking towards!
2goto1 |
|
|
|
|
 |
mccarre
|
Posted: Sat Jun 19, 2010 9:33 pm Post subject: Re: creating enums in the function scope, possible in any language? |
|
|
I don't think I could ask for anything easier to write than that 2goto1, thanks.
I'll try this out and report back how will it works. I won't be trying it out till I'm done some other important tasks right now, but probably in a couple weeks.
I know one other possible way of doing it in C++ would be to use macro functions. The macro function could potentially build an enum using the preprocessor when I create a new node, in compile time.
My code is in C# right now, so I'll try the dynamic way first, but I may eventually port my code to C++ anyways, 'cause I'm becoming much more comfortable with C++ than C# now.
Btw, a "necro post" is one that revives an old or "dead" topic. I was poking fun at my self for doing such a thing. It's usually frowned upon, but I think it's justified considering I do not believe my question was still open.
Cheers. |
|
|
|
|
 |
copthesaint

|
Posted: Sat Jun 19, 2010 9:35 pm Post subject: RE:creating enums in the function scope, possible in any language? |
|
|
Necro post, quite obvious lol. What does a necromancer do? Brings what was dead, back to like, so Necro post? Bring the topic back to life, bumping it.
also, bnet = blizzard entertainment? lol |
|
|
|
|
 |
2goto1

|
Posted: Sat Jun 19, 2010 11:06 pm Post subject: RE:creating enums in the function scope, possible in any language? |
|
|
Hi mccarre, yep the compiled at runtime approach would be another interesting way to go about it. Let me know how either works out for you!
P.S. not sure but code access security may or may not be something to consider, if it matters at all.
2goto1 |
|
|
|
|
 |
|
|