Having trouble with the ++ operators
Author |
Message |
slider203
|
Posted: Sat Feb 13, 2010 4:42 pm Post subject: Having trouble with the ++ operators |
|
|
Hello for my Java class I need to calculate and out put the values from 1 to 5 using the ++ operator.
Ok im not sure if this is how to do it but here is my code when I run it it outputs 6, I am not sure if this is the right answer but my teacher said the values would be from 1 to 5 inclusive anyways please check it out and let me know what you think
Quote:
// OnetoFive
//
// This program calculates and outputs the values 1 to 5 inclusive.
class OnetoFive
{
public static void main (String args [])
{
int num1 = 1;
int num5 = 5;
System.out.println (num1 ++ + num5);
}
}
thanks anyhelp you can offer is appreciated. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
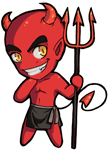
|
Posted: Sat Feb 13, 2010 5:30 pm Post subject: RE:Having trouble with the ++ operators |
|
|
Means the output should be
Quote:
1
2
3
4
5
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Euphoracle

|
Posted: Sat Feb 13, 2010 5:32 pm Post subject: RE:Having trouble with the ++ operators |
|
|
++ is a postfix operator. here's a code snippit in c
c: |
int c = 0;
// this outputs 0
printf("%d", c++ );
// but now the value of c is 1
// this outputs 1
printf("%d", c++ );
// but now the value of c is 2
// this outputs 2
printf("%d", c );
// the value of c is 2
|
basically, it returns the current value and increments the value by 1. This can be compared to the prefix operator which does it in a different order. ++c will return the new value of c. eg.
c: |
int c = 0;
// prints 1
printf("%d", ++c );
// value of c is now 1
// prints 1
printf("%d", c++ );
// value of c is now 2
|
|
|
|
|
|
 |
syntax_error

|
Posted: Sat Feb 13, 2010 5:46 pm Post subject: Re: Having trouble with the ++ operators |
|
|
Think of the problem you have at hand your output needs to be the following:
to use the variable++ you need to think what it does to the orginal value of the variable. For example the following code
Java: |
int count = 34;
system.out.println ("the original value of count is", count, "count with ++ is", count++);
/*in the memory of the var count it is now changed to 35 it is no longer stored as 34
so when you print out count once more you should get 35*/
system.out.println ("value of count", count);
|
SO you must think of the starting value of the variable if you ad doing var++ each time to out put it. |
|
|
|
|
 |
DtY

|
Posted: Sat Feb 13, 2010 7:52 pm Post subject: RE:Having trouble with the ++ operators |
|
|
I don't know why no one has said this yet, but you'll need to use a loop (most likely, a for loop). |
|
|
|
|
 |
slider203
|
Posted: Mon Feb 15, 2010 7:43 pm Post subject: Re: Having trouble with the ++ operators |
|
|
Hey cool thanks I think I got it now I have the output 1,2,3,4,5 and my code runs fine your answer really helped wish I could give Karma but dont have enough posts sorry..but you rock :p
syntax_error @ Sat Feb 13, 2010 5:46 pm wrote: Think of the problem you have at hand your output needs to be the following:
to use the variable++ you need to think what it does to the orginal value of the variable. For example the following code
Java: |
int count = 34;
system.out.println ("the original value of count is", count, "count with ++ is", count++);
/*in the memory of the var count it is now changed to 35 it is no longer stored as 34
so when you print out count once more you should get 35*/
system.out.println ("value of count", count);
|
SO you must think of the starting value of the variable if you ad doing var++ each time to out put it. |
|
|
|
|
 |
|
|