Random Ruby Ridiculousness
Author |
Message |
wtd
|
Posted: Sun Jan 24, 2010 2:47 am Post subject: Random Ruby Ridiculousness |
|
|
Ruby: | class Any
def initialize(enum)
@enum = enum
end
def ==(other)
@enum.any? { |x| x == other }
end
end
class All
def initialize(enum)
@enum = enum
end
def ==(other)
@enum.all? { |x| x == other }
end
end
module Enumerable
def any
Any.new self
end
def all
All.new self
end
end
if [1, 2, 3].any == 3
puts "Whee!"
end |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Sun Jan 24, 2010 2:53 am Post subject: RE:Random Ruby Ridiculousness |
|
|
Ruby: | class Fixnum
def even?
self % 2 == 0
end
end
class Any
def initialize(enum)
@enum = enum
end
def method_missing(symbol, *args)
@enum.any? { |x| x.send(symbol, *args) }
end
end
class All
def initialize(enum)
@enum = enum
end
def method_missing(symbol, *args)
@enum.all? { |x| x.send(symbol, *args) }
end
end
module Enumerable
def any
Any.new self
end
def all
All.new self
end
end
if [1, 2, 3].any.even?
puts "Whee!"
else
puts "Whoa!"
end
if [1, 2, 3].all.even?
puts "Gah!"
else
puts "Wah!"
end |
|
|
|
|
|
 |
Insectoid

|
Posted: Sun Jan 24, 2010 10:44 am Post subject: RE:Random Ruby Ridiculousness |
|
|
I dunno what modules do, but I gather that you've made classes initialized by methods in a module which return boolean values from an expression.
What I don't understand is what
code: |
def method_missing(symbol, *args) | does, or why you don't pass parameters into All and Any's constructors. |
|
|
|
|
 |
Tony
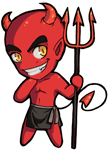
|
Posted: Sun Jan 24, 2010 11:01 pm Post subject: RE:Random Ruby Ridiculousness |
|
|
Ruby: |
class Foo
def method_missing(symbol, *args)
puts "Foo is missing method '#{symbol}', with arguments '#{args}'"
end
end
|
code: |
> Foo.new.bar "baz"
Foo is missing method 'bar', with arguments 'baz'
=> nil
|
Any and All constructors _do_ get parameters, in a way that "baz" is a parameter in an above example. That is, parenthesis are not strictly necessary.
Recall that + is a method on Fixnum class.
But we don't need to explicitly specify .method or (argument) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
wtd
|
Posted: Sun Jan 24, 2010 11:37 pm Post subject: RE:Random Ruby Ridiculousness |
|
|
Minor clean-up:
Ruby: | class Fixnum
def even?
self % 2 == 0
end
end
EnumerableWrapper = Struct.new :enum
class Any < EnumerableWrapper
def method_missing(symbol, *args)
@enum.any? { |x| x.send(symbol, *args) }
end
end
class All < EnumerableWrapper
def method_missing(symbol, *args)
@enum.all? { |x| x.send(symbol, *args) }
end
end
module Enumerable
def any
Any.new self
end
def all
All.new self
end
end
if [1, 2, 3].any.even?
puts "Whee!"
else
puts "Whoa!"
end
if [1, 2, 3].all.even?
puts "Gah!"
else
puts "Wah!"
end |
|
|
|
|
|
 |
|
|