[Quick-Tutorial] Cheating
Author |
Message |
Saad

|
Posted: Tue Apr 29, 2008 6:37 pm Post subject: [Quick-Tutorial] Cheating |
|
|
Cheat
No, I'm not talking about cheating as in copying and etc. This post is about the cheat function in Turing.
Introduction
Lets read the definition of cheat first (From the Turing help files)
Quote: A type cheat interprets the representation (bits) of one type as another type. Type cheats are dirty (machine-dependent) and sometimes dangerous (arbitrary corruption) and should be used only by programmers who know the underlying computer representation of values.
So you might be lost and utterly confused so I'll define it in simpler terms. cheat allows us to convert the internal representation of a type into another type.
Cheat Function Definition
code: | cheat (targetType, expn [ : sizeSpec ] ) |
Defining each:
- targetType would be the type to convert to
- expn [ : sizeSpec ] would be the expression/variable to convert to the targetType
Code
Lets look at our first code
Turing: | put cheat (int, 'A')
|
This would output 65. Why?
Lets look at the binary representation of 'A'. 'A' is represented by its Ascii value which happens to be 65 (In Binary 01000001). Turing takes the 01000001 and then converts that into an integer which is a 65.
Requisite for second example
Pointers
Now lets look at some pointer manipulation. A pointer just points to the an address in memory it stores an addressint. Now Turing doesn't allow us to assign pointers the address of another variable (I dont know why), however we can use cheat to make it point to a variable.
Turing: |
var foo := 10
var fooPointer : unchecked ^ int
cheat (addressint, fooPointer ) := addr (foo )
put ^fooPointer
foo := 1
put ^fooPointer
|
The output will be 10 and 1 respectively
Lets go through the code more deeply
Turing: | var fooPointer : unchecked ^int
|
This creates an unchecked pointer to an integer. We chose unchecked because we don't want to create a new integer with the current pointer and thus checking will fail.
Turing: | cheat (addressint, fooPointer ) := addr (foo ) |
This is the core part of our code.
Turing: | cheat (addressint, fooPointer) |
This means that we want to sets fooPointer's representation to an addressint
This returns the address of the variable (The type is addressint) which is coverted by the cheat to an addressint.
Turing: | put ^fooPointer
foo := 1
put ^fooPointer
|
We then use the derefencing operators to get what the value pointer by fooPointer is (which we set to be Foo)
That's all for now and thanks for reading! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Nick

|
Posted: Tue Apr 29, 2008 6:44 pm Post subject: RE:[Quick-Tutorial] Cheating |
|
|
wow... it's really that simple?
I when first saw the cheat function I thought it was really confusing, but the way you explained it makes it sound simple
I hope there's more to come
+ karma (as bits are kinda worthless) |
|
|
|
|
 |
CodeMonkey2000
|
Posted: Tue Apr 29, 2008 6:44 pm Post subject: RE:[Quick-Tutorial] Cheating |
|
|
Hey, this is just type casting! But it feels kind of weird in turing. |
|
|
|
|
 |
Mackie

|
Posted: Tue Apr 29, 2008 9:58 pm Post subject: RE:[Quick-Tutorial] Cheating |
|
|
Excellent Tutorial! I Wasn't even aware of this things existence. But, I suppose it could come in handy.
+Bits
+Karma |
|
|
|
|
 |
Tony
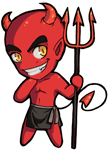
|
Posted: Tue Apr 29, 2008 11:55 pm Post subject: Re: [Quick-Tutorial] Cheating |
|
|
I think that the first example might be a bit misleading. Or at least skips over some important parts.
Saad @ Tue Apr 29, 2008 6:37 pm wrote:
Lets look at our first code
Turing: | put cheat (int, 'A')
|
This would output 65. Why?
Lets look at the binary representation of 'A'. 'A' is represented by its Ascii value which happens to be 65 (In Binary 01000001). Turing takes the 01000001 and then converts that into an integer which is a 65.
It's not that 'A' is represented by ASCII value of 65. Actually string literals could be kind of confusing, so lets treat it as var _a : char(1) := 'A' instead (this is what the example implies anyway). A single character takes up a block of memory, 1 byte in size. We treat _a as a pointer, read for the length taken up by the type, and interpret what kind of an integer that data could represent. That's cheat. Things get more complicated if we are dealing with less compatible types. Try to interpret cheat(int, "Tony") as an exercise.
Now I don't think it's very useful to take literal interpretations of binary data as different variables types, but this does provide a way to get some fancy work done on raw memory data. Nice. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Tyr_God_Of_War
|
Posted: Wed Feb 10, 2010 8:41 pm Post subject: Re: [Quick-Tutorial] Cheating |
|
|
Another area where cheat is very useful is when trying to do anything with natural numbers.
Whenever a nat is used with an int, it becomes an int.
In Turing's help page for natural numbers ("nat"), it suggests doing this
Turing: |
const natOne : nat := 1 %This is silly and un-natural
var myNat:nat:=16#80000000 %Larger than maxint
%elsewhere in the code
myNat := myNat + natOne
%BECAUSE...
myNat := myNat +1
% ...fails because Turing gets an integer overflow error because 1 is an int, not a nat.
% However,...
myNat := myNat + cheat(nat, 1)
% works fine.
|
|
|
|
|
|
 |
|
|