Don't really understand passing function arguments
Author |
Message |
sheepo39
|
Posted: Fri Dec 11, 2009 5:46 pm Post subject: Don't really understand passing function arguments |
|
|
I'm working through a beginning C++ book, and I'm at passing function arguments, and I don't really understand it.
The code example is
#include <iostream>
using namespace std ;
float fToC ( float degreesF = 32.0 ) ;
// A C++ application demonstrating passing arguments in functions
int main()
{
float fahrenheit, celsius ;
cout << "Enter a Fahrenheit temperature:\t" ;
cin >> fahrenheit ;
celsius = fToC( fahrenheit ) ;
cout << fahrenheit << "F is " << celsius << "C" ;
cout << endl << "Freezing point: " << fToC() << "C" << endl ;
return 0 ;
}
float fToC ( float degreesF )
{
float degreesC = ( ( 5.0 / 9.0 ) * ( degreesF - 32.0 ) ) ;
return degreesC ;
}
I don't understand the part of
celsius = fToC( fahrenheit ) ;
and I was hoping if somebody could help explain it to me
I also don't understand the whole, passing by value concept.
Thanks, John |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
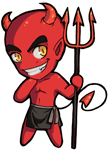
|
Posted: Fri Dec 11, 2009 5:55 pm Post subject: RE:Don\'t really understand passing function arguments |
|
|
May I suggest that you start introduction to programming with a different language? C++ is notoriously difficult to understand and use; it's a very poor choice for introducing oneself to the basic concepts (as anything basic is also wrapped around in a really complex language).
Also, shouldn't the book itself explain the concepts it's introducing?
And try to use [ code ] tags when posting code. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
sheepo39
|
Posted: Fri Dec 11, 2009 6:04 pm Post subject: Re: Don't really understand passing function arguments |
|
|
Oh sorry, I worded what I said wrong. I'm not a beginner programmer, although, I am a beginner programmer in C. I have past experience in lagnauges like Basic/VBasic, and Java, and Turing. Although a bit limited in some. |
|
|
|
|
 |
jbking
|
Posted: Fri Dec 11, 2009 6:21 pm Post subject: Re: Don't really understand passing function arguments |
|
|
sheepo39 @ Fri Dec 11, 2009 3:46 pm wrote: I don't understand the part of
celsius = fToC( fahrenheit ) ;
and I was hoping if somebody could help explain it to me
I also don't understand the whole, passing by value concept.
That line is where the function is called by passing in a value to convert. Rather than write the conversion directly in the main, the actual work of converting from one scale to another is done in a function so that you don't have to know exactly how that is done. Were you taught procedural or OO programming previously? Both paradigms use this concept of allowing reuse,e.g. imagine if that function along with many other imperial to metric functions were contained in a library that could be reused easily.
I think you may have to understand what a function is, and then you could move on to the whole pass by reference vs. pass by value idea. |
|
|
|
|
 |
Barbarrosa

|
Posted: Fri Dec 11, 2009 10:00 pm Post subject: Re: Don't really understand passing function arguments |
|
|
Passing by value:
code: |
Object 1 has value X
Object 2 has value Y
Pass to function taking value: {
Object 1 = Object 2
Object 1 has value Y
}
Object 1 has value X
|
As opposed to passing by reference:
code: |
Object 1 has value X
Object 2 has value Y
Pass to function taking reference: {
Object 1 = Object 2
Object 1 has value Y
}
Object 1 has value Y
|
You can pass by value or by reference between functions. You can also pass using pointers, but that can make things a lot more convoluted.
Java usually passes by reference, except for primitive types. C++ usually passes by value.
In C++, however, you can instantiate a reference variable, which behaves much like a Java reference variable, like so (note the ampersand):
code: |
int x = 1;
int& foo = x;
|
You may want to look up more on that.
If you call a function that takes a reference variable with a normal variable, whatever that function does to the variable will change the it. e.g.:
code: |
void chax(int& x){
x = 4;
}
int main(){
int x = 1;
chax(x);
std::cout << x; //should print out 4
}
|
|
|
|
|
|
 |
Tony
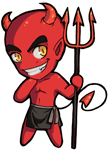
|
Posted: Fri Dec 11, 2009 11:07 pm Post subject: Re: Don't really understand passing function arguments |
|
|
Barbarrosa @ Fri Dec 11, 2009 10:00 pm wrote:
Java usually passes by reference, except for primitive types.
No. Java passes by value. Always. In Java, Object references are passed by value.
Java: |
class Test{
static void swap(String obj){
obj = new String("new");
}
public static void main(String[] args) {
String foo = new String("old");
swap(foo);
System.out.println(foo); // prints old
}
}
|
Seriously.
code: |
$ javac test.java
$ java Test
old
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Barbarrosa

|
Posted: Fri Dec 11, 2009 11:36 pm Post subject: Re: Don't really understand passing function arguments |
|
|
Sorry, I said that wrong. My bad.
Yes, Java passes object references by value. It also passes the primitive types by value. |
|
|
|
|
 |
Insectoid

|
Posted: Sat Dec 12, 2009 12:40 am Post subject: RE:Don\'t really understand passing function arguments |
|
|
Unlike Actionscript, in which everything is passed by reference and you have to cheat a bit to duplicate variables (ie array2 = array1.concat() ) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|