Using a Stack
Author |
Message |
Bashar
|
Posted: Wed Dec 10, 2008 3:34 pm Post subject: Using a Stack |
|
|
Hello,
I am doing an assignment on stacks. Here's part b of the question, which I am stuck on:
Quote: b) [5 marks] Complete the following method which modifies a stack of CarInfo objects by removing all cars of a specified colour, leaving the remainder of the stack unchanged. Note that you may use one additional stack variable in this question.
public static void removeByColour(StackInterface s, String colour)
/* pre: not null, all entries on s are of type CarInfo
* post: removes from s all CarInfo objects with specified colour
* Note: the values remaining on s have the same relative order as when the
* method was called.
*/
This is the class CarInfo
code: | public class CarInfo
{ // instance variables for unique Car identification number, and for the colour of the car
private int id;
private String colour;
/* CarInfo constructor
* pre: idNum different from all other CarInfo ids, c is a String corresponding to a colour
* post: CarInfo object created
*/
public CarInfo(int idNum, String c)
{ this.id = idNum;
this.colour = c;
}
/* getID
* post: returns the unique car ID number
*/
public int getID() { return this.id; }
/* getColour
* post: returns the colour of the car
*/
public String getColour() { return this.colour; }
/* paint
* pre: newColour != null
* post: this car's colour is set to newColour
*/
public void paint(String newColour)
{ this.colour = newColour;
}
/* equals
* post: return true iff the id number and colours of the cars match
*/
public boolean equals(CarInfo other)
{ if (other==null) return false;
return this.id==other.id && this.colour.equals(other.colour);
}
/* toString
* post: returns a String of the format "id: colour" for this car
*/
public String toString()
{ return "" + id + ": " + colour;
}
} |
This is what I have for the solution with the problematic line highlighted:
code: | public static void removeByColour( StackInterface stk, String clr ) {
Stack temp = new Stack ();
Object tempO = new Object();
int index = 0;
while (!stk.isEmpty()){
tempO = stk.pop();[color=red]
if (tempO.getColour() != clr) temp.push (tempO);[/color]
}
while (!temp.isEmpty()){
tempO = temp.pop();
stk.push (tempO);
}
} |
I created tempO as an object, which means I don't have access to the methods of CarInfo. However, I need CarInfo methods to check for the color. The Pre-condition of the method says that I can assume everything in the original stack (stk) is a CarInfo instance, but I am not sure how that helps!
What am I missing? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
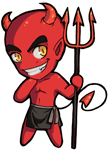
|
Posted: Wed Dec 10, 2008 3:51 pm Post subject: Re: Using a Stack |
|
|
Bashar @ Wed Dec 10, 2008 3:34 pm wrote: I created tempO as an object, ... However, ... The Pre-condition .. says that ... everything in the original stack (stk) is a CarInfo instance
So, what class should "tempO" be? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Bashar
|
Posted: Wed Dec 10, 2008 5:34 pm Post subject: Re: Using a Stack |
|
|
It should be CarInfo. However, the problem is that I need to pass two parameters to the constructor. I am unsure of the values to pass to initialize tempO.
What I tried to do is to downcast tempO later on, and that seems to work.
Except it gives me this:
File: /Users/basharjabbour/Documents/University/CS134/Ass. 5/StackQ.java [line: 41]
Warning: [unchecked] unchecked call to push(E) as a member of the raw type java.util.Stack |
|
|
|
|
 |
HeavenAgain

|
Posted: Wed Dec 10, 2008 5:56 pm Post subject: RE:Using a Stack |
|
|
To avoid the warning, the element type for the Stack object must be the same as the element you are trying to push in.
By default the Stack element is Object. But you can simply change it into CarInfo.
ie.
code: | Stack<CarInfo> temp = new Stack<CarInfo> (); |
The same can be applied to any object. eg. String, Integer, etc. |
|
|
|
|
 |
|
|