Alternating turns
Author |
Message |
Roman
|
Posted: Thu Aug 07, 2008 4:58 pm Post subject: Alternating turns |
|
|
In my Tic-Tac-Toe game I want to make alternating turns, so that the first time you click it's an X, then when you click on a different field it's an O and so on.
I'm thinking of creating a turnControl function that would keep track of turns basically by itself. Is that possible, or do I need to assist it by sending it information?
I'm thinking of something like:
code: |
def testTurnControl():
for i in range(9):
print turnControl()
def turnControl():
?????
|
I want to make the turn control return alternate values. 1, 2, 1, 2, 1, etc... Any help would be greatly appreciated =) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
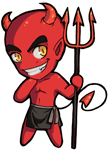
|
Posted: Thu Aug 07, 2008 5:35 pm Post subject: RE:Alternating turns |
|
|
There are a number of ways to implement this, though all of them involve keeping track of who has control over the current tern, and then flip to the other one.
You can do if - else
You can do (turn++) mod 2
My favourite approach though is that when you multiply a number by -1, it just flips the sign of the result back and forth.
code: |
current_turn = current_turn * -1
|
will give you a sequence of 1, -1, 1, -1, etc. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DemonWasp
|
Posted: Thu Aug 07, 2008 11:36 pm Post subject: RE:Alternating turns |
|
|
Tony's method will definitely work for two players, and is pretty cool. If you're wanting to work with arrays, however, it may require some extra work.
My preferred method would be something like:
code: |
// Lame pseudocode
currentPlayer = ( currentPlayer + 1 ) modulus (number of players)
|
(The modulus is just the remainder after division, so 5 modulus 2 is 1, and 37 modulus 5 is 2, etc)
With this, you'd have currentPlayer take values 0, 1, 0, 1, 0, 1, ... assuming two players. With more players, it would take on a cycle like 0, 1, 2, 3, 4, 0, 1, 2, 3, 4, ...
Edit: looking closer, I note that Tony already covered this. Whoops. |
|
|
|
|
 |
Roman
|
Posted: Fri Aug 08, 2008 2:22 am Post subject: Re: Alternating turns |
|
|
Thanks guys! I was trying different ways but got what I guess is the programmer's version of writer's block...
I ended up getting even more help (god I need it x_x) and have the following:
code: |
def turnControl(self):
self.x += 1
return (self.x%2 and 1) or 2
|
self.x is defined as 0 at the beginning. The reason for this was so I could sub in the 1 and 2 right away into a different function (which accepts 1 or 2, so I didn't change the design much). The *(-1) way worked well too. So many ways to do it x_x
Thanks a lot for the help! One step closer to a fully playable, bugless version of Tic Tac Toe haha  |
|
|
|
|
 |
|
|