Author |
Message |
Junaid2pac
|
Posted: Sun Dec 30, 2007 8:15 pm Post subject: Accessing a variable from one class to another |
|
|
//1st class:
class GameFrame extends JFrame
public JTextField getVelocity;
//2nd class:
GameFrame vx;
int getDx = Integer.parseInt(vx.getVelocity.getText().trim());
Question: Would this be the correct way of accessing one variable from one class to another? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Mon Dec 31, 2007 12:32 am Post subject: RE:Accessing a variable from one class to another |
|
|
Ideally, no. The variable should be encapsulated, so that accessing it requires a getVelocity method, and setting a new value requires a setVelocity method.
I would also suggest a naming along the lines of:
code: | class GameFrame extends JFrame {
private JTextField velocityTextField;
public JTextField getVelocityTextField() {
return velocityTextField;
}
} |
code: | int getDx = Integer.parseInt(vx.getVelocityTextField().getText().trim()); |
Or better:
code: | class GameFrame extends JFrame {
private JTextField velocityTextField;
public int getVelocity() {
return Integer.parseInt(velocityTextField.getText().trim());
}
} |
code: | int getDx = vx.getVelocity(); |
|
|
|
|
|
 |
Tony
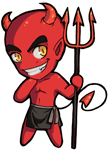
|
Posted: Mon Dec 31, 2007 12:57 am Post subject: RE:Accessing a variable from one class to another |
|
|
indeed it is was wtd has said.
code: |
public JTextField getVelocity;
|
is way too misleading. One would expect getVelocity to be a method and return the Velocity. It is certainly not obvious that it actually holds a textfield that could hold an arbitrary value. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Junaid2pac
|
Posted: Mon Dec 31, 2007 4:20 pm Post subject: Re: Accessing a variable from one class to another |
|
|
thanks |
|
|
|
|
 |
Junaid2pac
|
Posted: Mon Dec 31, 2007 4:30 pm Post subject: Re: Accessing a variable from one class to another |
|
|
but it still gives me the following error:
Exception in thread "AWT-EventQueue-0" java.lang.NullPointerException
at Ball.<init>(Junaid_Java_ISU.java:124)
----------------------------------------------------------------------------------------
which is at:
int getDx = vx.getVelocity(); |
|
|
|
|
 |
Junaid2pac
|
Posted: Mon Dec 31, 2007 5:22 pm Post subject: Re: Accessing a variable from one class to another |
|
|
also:
[/code]
//First Class
class GameFrame extends JFrame
{
public GameFrame()
{
velocityText = new JTextField(2);
velocityText.setText("2");
public int getVelocity()
{
return Integer.parseInt(velocityText.getText().trim());
}
addButton(p, "Start", new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
Ball b = new Ball(canvas);
b.start();
}
});
}
public void addButton(Container c, String title, ActionListener a)
{
JButton b = new JButton(title);
c.add(b);
b.addActionListener(a);
}
}
//2nd Class
class Ball extends Thread
{
GameFrame vx;
int getDx = vx.getVelocity(); //This is where the error points to
}
code: |
it compiles and shows the number 2 in velocityText field, but when i press start it gives me that error..........but when i get rid of the line: int getDx = vx.getVelocity();
It works |
|
|
|
|
|
 |
Junaid2pac
|
Posted: Mon Dec 31, 2007 5:22 pm Post subject: Re: Accessing a variable from one class to another |
|
|
sorry i didnt know how to use the code thing |
|
|
|
|
 |
HeavenAgain

|
Posted: Mon Dec 31, 2007 5:27 pm Post subject: RE:Accessing a variable from one class to another |
|
|
that is because in your 2nd class the vx object of GameFrame does not point to anything to fix this try this
code: | //2nd Class
class Ball extends Thread
{
GameFrame vx = new GameFrame();
int getDx = vx.getVelocity(); //This is where the error points to
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Junaid2pac
|
Posted: Mon Dec 31, 2007 5:31 pm Post subject: Re: Accessing a variable from one class to another |
|
|
Thanx....finally its working |
|
|
|
|
 |
Junaid2pac
|
Posted: Mon Dec 31, 2007 5:43 pm Post subject: Re: Accessing a variable from one class to another |
|
|
it works but only once
When i change the value in the textfield it keeps the velocity that was set in the text field at in the beginning.
How can i change my code so it gets the value each time i click the start button |
|
|
|
|
 |
HeavenAgain

|
Posted: Mon Dec 31, 2007 5:48 pm Post subject: RE:Accessing a variable from one class to another |
|
|
you have a very mess code there, and its really hard to read off code which is not organized, but from the look of the code, your value comes from the constructor of your GameFrame class (you are using it to prompt user for a value useing JTextField (which is wrong) constructor should only "over write" the default ones, by giving the new default value.
Now to fix your problem, you can try something like this in your 2nd class
code: | class Ball extends Thread
{
GameFrame vx;
while (true)
{
vx = new GameFrame();
int getDx = vx.getVelocity(); //This is where the error points to
}
} | but this is not how it should be done |
|
|
|
|
 |
Junaid2pac
|
Posted: Wed Jan 02, 2008 7:50 pm Post subject: Re: Accessing a variable from one class to another |
|
|
i am still sort of confused as to why the velcoity of the ball only works for the initial value assigned to the text field.
i used this:
code: |
velocityText.setText("5");
|
in the first class called GameFrame
After that changing the value inside the text field box dosnt acutally change the velocity, it stays the same
Pls help |
|
|
|
|
 |
Junaid2pac
|
Posted: Wed Jan 02, 2008 7:51 pm Post subject: Re: Accessing a variable from one class to another |
|
|
I also have this in the first class to constantly checking the text field
code: |
public int getVelocity()
{
return Integer.parseInt(velocityText.getText().trim());
} |
|
|
|
|
|
 |
Junaid2pac
|
Posted: Wed Jan 02, 2008 10:33 pm Post subject: Re: Accessing a variable from one class to another |
|
|
I just realized i don't have an addActionListener
but when i add this to the GameFrame Class:
code: |
velocityText.setText("5");
velocityText.addActionListener(this); |
it gives me the following error:
addActionListener(java.awt.event.ActionListener) in javax.swing.JTextField cannot be applied to (GameFrame) |
|
|
|
|
 |
Junaid2pac
|
Posted: Wed Jan 02, 2008 10:54 pm Post subject: Re: Accessing a variable from one class to another |
|
|
i believe i have figured out my problem, but in the action performed method...how do you show which button u r talking about when there is more than 1? |
|
|
|
|
 |
|