Polygon Class
Author |
Message |
batman
|
Posted: Fri Nov 03, 2006 6:05 pm Post subject: Polygon Class |
|
|
Alright, so im trying to work on a polygon class but im having troubles with it. I know how to do a rectangle class, a circle class but im kinda confused about polygons. I have no clue how to do a polygon class. I ve done all ta i could. Can someone help me draw a polygon?????????
I think have to set the coordinates so the center is at 0,0 so some of te coordinates are negative. I need help fixing my code.
code: |
import java.awt.*;
public class Polygon
{
int x, y; // centre of object
public int width;
public int height;
Color c;
public Polygon (int initialX, int initialY, int initialHeight)
{
x = initialX;
y = initialY;
height = initialHeight;
width = height / 2;
c = Color.BLUE;
}
public Rect (int initialX, int initialY, int initialHeight, Color initialColor)
{
x = initialX;
y = initialY;
height = initialHeight;
width = height / 2;
c = initialColor;
}
public void setPosition (int newX, int newY)
{
x = newX;
y = newY;
}
public void setHeight (int newHeight)
{
height = newHeight;
width = height / 2;
}
public void draw (Graphics g)
{
int drawX = x - width / 2;
int drawY = y - height / 2;
g.setColor (c);
g.fillPolygon (drawX, drawY, width, height);
}
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Booya
|
Posted: Fri Nov 03, 2006 6:10 pm Post subject: (No subject) |
|
|
I think there is somethin wrong with ur code when you are trying to draw the polygon at the end. A polygon has six coordinates not 4. How u fix this im not sure because im newbe. |
|
|
|
|
 |
ericfourfour
|
Posted: Sat Nov 04, 2006 1:10 am Post subject: (No subject) |
|
|
Actually, a polygon as more than 2 sides a hexagon has 6. Your polygon should be made up of triangles though. That way it will be easier to deal with collisions. |
|
|
|
|
 |
Dan
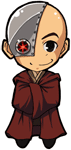
|
Posted: Sun Nov 05, 2006 2:41 pm Post subject: (No subject) |
|
|
A polygon is any shape made up of at least 3 lines that conect to each other. Java has a bulit in polygon class that can show you how it is done.
The easyest way to make a polygon is to take a 2d array of x and y quardents and then draw line segnements between the points. Then join the last x and y to the first x and y to complet the shape. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Clayton

|
Posted: Mon Nov 06, 2006 8:49 am Post subject: (No subject) |
|
|
ie, in Turing the code would look something like this:
Turing: |
procedure Polygon (x,y : array 1 .. * of int, clr : int)
for i : 1 .. upper (x ) - 1
Draw.Line (x (i ),y (i ), x (i + 1), y (i + 1), clr )
end for
Draw.Line (x (upper(x )),y (upper(y )),x (1),y (1),clr )
end Polygon
|
|
|
|
|
|
 |
ericfourfour
|
Posted: Mon Nov 06, 2006 4:54 pm Post subject: (No subject) |
|
|
Well, if you wanna save yourself a Draw.Line use this.
code: | type coord :
record
x, y : real
end record
proc polygon (pos : array 0 .. * of coord, clr : int)
for id1 : 0 .. upper (pos)
var id2 := (id1 + 1) mod (upper (pos) + 1)
Draw.Line (round (pos (id1).x), round (pos (id1).y), round (pos (id2).x), round (pos (id2).y), clr)
end for
end polygon |
To get the second id just add one to the first id and then get the remainder between that and the amount of positions.
code: | second id = (first id + 1) mod (amount of positions) |
I think (I'm not completely sure) in Java it would be:
code: | id2 = (id1 + 1) % pos.length |
|
|
|
|
|
 |
gsquare567
|
Posted: Tue Nov 07, 2006 11:19 pm Post subject: (No subject) |
|
|
what you have to do is make an array of the x's and y's and then they'll pair up when you drawPolygon.. eg.
code: |
// should give you a diamond =D
int[] x = {-2, 0, 2, 0};
int[] y = {0, 2, 0, -2};
g2d.setColor(new Color(Color.Blue));
g2d.drawPoly(x,y);// something like this... probs ton of errors
|
|
|
|
|
|
 |
gsquare567
|
Posted: Tue Nov 07, 2006 11:20 pm Post subject: (No subject) |
|
|
sorry instead of drawPoly i meant drawFill()... or you could just use draw() if you dont want it filled in =) check out the graphics and graphics2d classes in da api |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
batman
|
Posted: Wed Nov 08, 2006 2:36 pm Post subject: Polygon |
|
|
Quote:
// should give you a diamond =D
int[] x = {-2, 0, 2, 0};
int[] y = {0, 2, 0, -2};
g2d.setColor(new Color(Color.Blue));
g2d.drawPoly(x,y);// something like this... probs ton of errors
I already know how to draw a polygon thanks for the help though but i was talking about making it in a class then extending using an applet to draw the polyogn. I figured it out but thanks for the help. By the way grd.fillPolygon wont work unless u have x.length,x and y. Not just x,y. |
|
|
|
|
 |
gsquare567
|
Posted: Fri Nov 10, 2006 8:36 pm Post subject: (No subject) |
|
|
no we were both wrong it wud be g2d.drawFill(x[], y[]);  |
|
|
|
|
 |
|
|