Tip: Writing Cleaner Code
Author |
Message |
Hackmaster
|
Posted: Thu Nov 02, 2006 9:40 am Post subject: Tip: Writing Cleaner Code |
|
|
I have lately encountered some problems when looking at some of my friends' code...largely that they program like they hate humans. there are a few, very simple, yet crucial things you can do for people who are reading your code to make it MUCH easier to read. The reason that people comment, and make better code in general is simply so one programmer can read the others code.
1. COMMENT!!!
how do people know what you are doing in a complicated program?
code: |
var ans:string
var mat:real
var lotsofcomplexstufflikethis:string
loop
loop
loop
put"stuff would go here in this triple nested loop"
put"blah blah complicated"
end loop
end loop
end loop
|
wow. who would write this? I don't know. but, you can improve this with comments. observe:
code: |
%This is what this program does, and this is it's title
var ans:string %this variable does this
var mat:real %this variable does this
var lotsofcomplexstufflikethis:string %this variable does this
%this bunch of loops is used to do this
loop
loop
loop
%this acomplishes this
put"stuff would go here in this triple nested loop"
put"blah blah complicated"
end loop
end loop
end loop
%end program.
|
better... but not great. we need more than comments. Also note that you don't really need comments on your variable names, but if they have if they have some complicated and specific purpose, it might be a good idea. because this program is long and boring, we need some separation. look at this, it's hard to tell what does what because everything is one chunk of text. try this:
2.WHITESPACE
code: |
var ans:string %this variable does this
var mat:real %this variable does this
var lotsofcomplexstufflikethis:string %this variable does this
%this bunch of loops is used to do this
loop
loop
loop
%this acomplishes this
put"stuff would go here in this triple nested loop"
put"blah blah complicated"
end loop
end loop
end loop
%end program.
|
better... how about indenting? if white space is good, indenting and white space is better. Turing has an indent button for a reason.
3.INDENTING
code: |
var ans : string %this variable does this
var mat : real %this variable does this
var lotsofcomplexstufflikethis : string %this variable does this
%this bunch of loops is used to do this
loop
loop
loop
%this acomplishes this
put"stuff would go here in this triple nested loop"
put"blah blah complicated"
end loop
end loop
end loop
%end program.
|
one more thing will help... good variable names. no one likes a,b,x,and ans as a name for a variable. this is the final code update:
4.GOOD VARIABLE NAMES!!
code: |
var answer : string %this variable does this
var mathHolder : real %this variable does this
var lots_Of_Complex_Stuff_Like_This : string %this variable does this
%this bunch of loops is used to do this
loop
loop
loop
%this acomplishes this
put"stuff would go here in this triple nested loop"
put"blah blah complicated"
end loop
end loop
end loop
%end program.
|
Make more complete names. extra typing won't kill you. depending on the length of the variable, as said above, it would be better to either add '_' between words, or just Capitalize them.
that's all folks, i hope you find these tips useful! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Clayton

|
Posted: Thu Nov 02, 2006 10:42 am Post subject: (No subject) |
|
|
errr.... Once again, someone has done this already. However it is nice that you are trying. If you really feel the urge to write a tutorial, take a good long look through
- The Turing Walkthrough
- Improving Tutorials Thread
- The actual current tutorial you want to revamp/re-write, if you think you can surpass its quality quite a bit, message Cervantes or Tony to get approval.
After you have done all of those steps, write the tutorial (preferrably in some text editor like TextPad. Then, message the entire thing (BBCode and all) to either Cervantes or Tony for suggestions and final approval. Then post it.
Hackmaster wrote:
put caps at the beginning of new words in variables, and make more complete names. extra typing won't kill you.
What about this_kind_of_name? It's really about what suits you, just pick a style and stay with it. Also, on commenting, you don't have to comment on every single variable that you declare. If you have something like this:
Turing: |
var name_of_gandalf : string := "Gandalf"
|
It would be very redundant to comment that it is a string containing the name of Gandalf. There is such a thing as over commenting. Too much and your code just gets harder to read instead of easier. |
|
|
|
|
 |
Tony
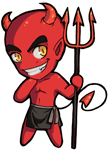
|
Posted: Thu Nov 02, 2006 11:22 am Post subject: (No subject) |
|
|
I second Freakman's observations.
It was an alright start, but you should realize something is up when your example code is longer than explanation of it.
Commenting is used to describe why you are doing something, not how you're doing it. How is in the code itself.
Good variables names? blah : string is not a good example of that.
Quote:
put caps at the beginning of new words in variables
Why? It's good practice to explain why it's one way or another. Especially if you don't have much authority yet (new member).
I'll explain why you're wrong here - capitalization of variable names is reserved for classes. So:
code: |
var num_of_sheep : int % variable
var my_sheep : pointer to Sheep
new Sheep, my_sheep % class instance
const FIELD : grass_field_type % constant
|
notice how I don't need to explain what any of those variables are for. I might need to write a comment to explain why I'm herding sheep though.  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
gugiey
|
Posted: Wed Nov 15, 2006 9:38 am Post subject: (No subject) |
|
|
jorden ur a champ.
i guess ill have to start useing them more offten |
|
|
|
|
 |
wtd
|
Posted: Wed Nov 15, 2006 5:20 pm Post subject: (No subject) |
|
|
Let me add something to this, just for fun:
Properly scope your variables. Very concise variable names become moderately more tolerable when they exist within a very small, very specific scope. |
|
|
|
|
 |
|
|