formating / traversing hash
Author |
Message |
Tony
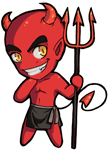
|
Posted: Fri Oct 13, 2006 11:38 am Post subject: formating / traversing hash |
|
|
given a hash of keys => values, I want to construct a conditional statement that the hash describes, in the form "a = b AND c = d", etc. So far I've came up with
code: |
irb(main):001:0> foo = {"a"=>"b","c"=>"d"}
=> {"a"=>"b", "c"=>"d"}
irb(main):002:0> bar = ""
=> ""
irb(main):003:0> foo.each{|key, value|
irb(main):004:1* bar = "#{bar} #{key} = #{value} AND" }
=> {"a"=>"b", "c"=>"d"}
irb(main):005:0> bar.slice!(0..-4)
=> " a = b AND c = d "
|
which seems like a pretty ugly solution. I especially don't like that last hack on a leftover "AND". Is there a more eligant approach?
While #collect gives me a nice array
code: |
irb(main):007:0> foo.collect
=> [["a", "b"], ["c", "d"]]
|
the #join acts as if the array was flattened
code: |
irb(main):006:0> foo.collect.join("*")
=> "a*b*c*d"
|
 |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Fri Oct 13, 2006 11:51 am Post subject: (No subject) |
|
|
What happens if you pass a block to collect?  |
|
|
|
|
 |
Tony
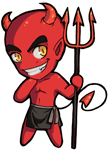
|
|
|
|
 |
wtd
|
Posted: Fri Oct 13, 2006 12:10 pm Post subject: (No subject) |
|
|
Tony wrote: code: |
irb(main):025:0> bar = foo.collect{|a| a.join("=")}.join(" AND ")
=> "a=b AND c=d"
|
excellent question, thank you 
You're welcome, though I would have said:
code: | foo.collect { |k, v| "#{k} = #{v}" }.join(" AND ") |
|
|
|
|
|
 |
|
|