Number List, having trouble!!
Author |
Message |
pileggi
|
Posted: Thu Oct 12, 2006 7:25 am Post subject: Number List, having trouble!! |
|
|
Hi
I'm trying to make a number list, going from
000000000
to
999999999
we're learning encryption in school, so we need to make a number list from 0 to a billion, but keeping the rest of the places filled with "0"
ex
000000001
000000002
etc.
(yes I realize this will make an incredibly large file, and my system will be dead for a while, I'm working on that)
i ran it this morning using Ready to Program Dr Java, run with args, print to file.. made a 160MB text file in like 15 mins.
So I need to figure out a way to code it in that it will write to a file, and change the file it writes to every hundred thousand numbers or something.. (I don't know how to write to files.. :-s we don't learn that)
Also, if you run my code, you'll notice the formatting goes off between 100, to 110.
And then later on from 10000 to 11000
there is an extra zero.. and probably every subsequent multiple of ten or something.. I don't know
Do you guys think it would be easier to create an array of 10 across, down by 1 billion. Initialize everything to zero, then just store numbers starting from the right side, into the array (using mod + div to break the numbers down into individual digits)
0 0 0 0 0 0 0 0 1
0 0 0 0 0 0 0 0 2
0 0 0 0 0 0 0 0 3
etc.
And then print the array to a file?
Is it even possible to make an array of one billion? How can I get around this :-s
code: |
class testpass
{
public static void main (String [] args)
{
int x=0;
for(int i2=0;i2<10;i2++)
{
for(int i3=0;i3<(1* Math.pow(10,i2));i3++)
{
for(int i=9;i>i2;i--)
{
System.out.print("0");
}
System.out.print(x);
x=x+1;
System.out.println();
}
}
}
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
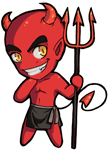
|
Posted: Thu Oct 12, 2006 11:39 am Post subject: (No subject) |
|
|
just use java.util.Formatter and format your integers to output leading zeros. I would additionally suggest turning the formatting off after the first 10%, when you no longer need it. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
CroAnte
|
Posted: Fri Oct 13, 2006 9:26 am Post subject: (No subject) |
|
|
If you want to break up the file in to separate ones every, say, 10 million, you could use nested loops:
code: |
for (int x=0; x<1000000000; x+=10000000)
{
//open file here
for (int y=0; y<10000000; y+=1)
{
z = x + y;
//generate numbers and write to file
}
// close file here
}
|
This will create 100 files. You may want to use your 'x' as your filename. |
|
|
|
|
 |
|
|