Author |
Message |
JSBN

|
Posted: Fri Jun 13, 2003 9:34 pm Post subject: Delaying |
|
|
Uh... how do you do a delay in java?
ie: in turing
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
octopi

|
Posted: Fri Jun 13, 2003 11:54 pm Post subject: (No subject) |
|
|
I have no idea about java, or if this will even make sense to you, but a simple search on google spit out this
Thread.sleep(DELAY);
Where DELAY is a number, any number in milliseconds (I think)
http://www.google.com |
|
|
|
|
 |
JSBN

|
Posted: Sat Jun 14, 2003 10:24 am Post subject: (No subject) |
|
|
i tried it but it just screws up my program :/
Thx for trying tho |
|
|
|
|
 |
Tony
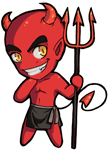
|
Posted: Sat Jun 14, 2003 11:45 am Post subject: (No subject) |
|
|
You could just run a huge forloop calculating garbage values to waste time  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
rizzix
|
Posted: Wed Jun 18, 2003 11:20 pm Post subject: (No subject) |
|
|
JSBN wrote: i tried it but it just screws up my program :/
Thx for trying tho
hey dude!
That is the way to do it.
You see the only problem it does not work is cuz u haven't caught the exception that is thrown by the static method sleep of the class Thread.
all you have to do is modify ur code to something like this..
code: |
try {
bla;
bla;
Thread.sleep(100);
} catch (InterruptedException e) {
System.out.println(e);
}
|
basically make sure the Thread.sleep(int msec); is in the try block and that there is a following catch block to catch the InterruptedException exception. ^^
I'll have a tutorial on why we have this funny syntax in Java soon after my exams. You'll see this try/catch/final exception handling used extensively in Java. It's something u need to know.
I'm planning on comming out with more tutorials to explain these concepts and more.. |
|
|
|
|
 |
poseidon
|
Posted: Wed Jul 23, 2003 10:46 pm Post subject: random numbers |
|
|
Is there possibly another way to delay without using try/catch and all that exception stuff?
dam .... doing java makes u appreciate the simplicity of turing .... one liners in turing are replaced w/ these long and complicated lines of bull in java. |
|
|
|
|
 |
rizzix
|
Posted: Thu Jul 24, 2003 3:05 pm Post subject: (No subject) |
|
|
Turing isn't multi threaded.. Java on the other hand is.. Your application runs in the main thread.. form this thread u can create new threads..
since your application is a thread, it has to trap this interrupt exception.
if ur sure ur not going spawn new threads ur self then u can try this:
code: |
public static void sleep(int msec) {
try {
  Thread.sleep(msec);
} catch (InterruptedException e) {
  System.out.println(e);
}
}
|
now u can call this method without encapsulating it in a try/catch block. since the method it self handles the exception. |
|
|
|
|
 |
poseidon
|
Posted: Thu Jul 24, 2003 8:52 pm Post subject: delaying |
|
|
hmmm ... alrite ill try ...
btw ..... this is probably a stupid q ... but wat does
code: | catch (InterruptedException e) |
do?
and wat's the purpose of the try/catch commands?
is it used to "catch" or prevent errors or something
btw thx man |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
SilverSprite
|
Posted: Thu Jul 24, 2003 10:05 pm Post subject: (No subject) |
|
|
Look in the tutorial section.. there is a tutorial on exception handling. |
|
|
|
|
 |
|