Author |
Message |
Martin

|
Posted: Mon Jul 03, 2006 7:44 am Post subject: Rope Physics |
|
|
Using the techniques in the Advanced Character Physics essay I linked to in [General Programming], I give you... 2d rope physics!
Just type make.
Left and right to rotate the cube, x to reset the rope.
Thanks to Nehe too.
EDIT: To compile this, you need to install libSDL's development libraries.
Cool things to do.
In main.cpp line 151, change frameskip's threshold higher or lower (this is my shitty way of making the app not run too fast.) Lower values will simulate higher gravity, and vice versa.
In particlesystem.cpp, on line 17 edit restlength to make the rope longer or shorter (restlength is the length between two adjacent nodes on the rope.) Also, on line 86, the tip of the rope is constrained to a point, which is why the rope hangs in space. You can add different constraints (constrain the other end somewhere and you can get a jump rope effect.)
Description: |
|
 Download |
Filename: |
particle.zip |
Filesize: |
40.34 KB |
Downloaded: |
629 Time(s) |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
apomb

|
Posted: Mon Jul 03, 2006 10:25 am Post subject: (No subject) |
|
|
i typed make, it said code: | /bin/sh: sdl-config: command not found
main.o: file not recognized: File format not recognized
collect2: ld returned 1 exit status
make: *** [main] Error 1
|
need a bit more instruction
|
|
|
|
|
 |
Martin

|
Posted: Mon Jul 03, 2006 10:54 am Post subject: (No subject) |
|
|
Oh, I forgot - you'll need to install libSDL to build the source!
|
|
|
|
|
 |
Tony
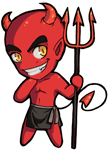
|
Posted: Mon Jul 03, 2006 1:36 pm Post subject: (No subject) |
|
|
Quote:
/bin/sh: line 1: sdl-config: command not found
I've got my SDL.Framework though.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Mazer

|
Posted: Mon Jul 03, 2006 2:20 pm Post subject: (No subject) |
|
|
Don't forget to make sure you have your opengl headers installed. And I had to add -lGL and -lGLU to the makefile before I could compile it (Does `sdl-config --libs` handle the OpenGL libs for you, Martin?).
It's pretty awesome though. Are you planning to add friction too?
|
|
|
|
|
 |
Martin

|
Posted: Mon Jul 03, 2006 6:40 pm Post subject: (No subject) |
|
|
Tony: The SDL Framework is apparently not good enough. Compile and install it from source for everything that you need.
Mazer: The makefile works in Cygwin and OSX as it is. sdl-config --libs gives me this on OS X: -L/sw/lib -lSDLmain -lSDL -framework Cocoa -framework OpenGL. Friction is coming soon, first though I want to make it work with boxes instead of just rope and sticks.
|
|
|
|
|
 |
Mazer

|
Posted: Mon Jul 03, 2006 6:54 pm Post subject: (No subject) |
|
|
Ah, ok. I just get -L/usr/lib -lSDL -lpthread, though I suspect it has to do with having libSDL precompiled. (Ubuntu Dapper repositories)
|
|
|
|
|
 |
wtd
|
Posted: Mon Jul 03, 2006 7:11 pm Post subject: (No subject) |
|
|
You should know that math.h is a C header. For fewer potential worries, use the "cmath" header.
Also, is there some reason the functions (aside from main) which currently reside in main.cpp are not separated out into a separate header/implementation file combo?
Oh, and if you're not doing the above, why forward declare the functions? Especially when you put main at the bottom of the file anyway.
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Mon Jul 03, 2006 7:25 pm Post subject: (No subject) |
|
|
You may find it useful to manage your rotating counter thingy:
code: | if (frameskip > 10 )
{
frameskip = 0;
ps.TimeStep();
} |
Via an object.
code: | class rotating_counter : private std::vector<void (*)(void)>
{
private:
const int _start;
const int _end;
int _current;
public:
rotating_counter(const int start, const int end)
: _start(start), _end(end), _current(start)
, std::vector<void (*)(void)>()
{ }
const int start() const { return _start; }
const int end() const { return _end; }
const int current() const { return _current; }
void increment()
{
_current += 1;
if (_current > _end) _current = _start;
// for each func in the vector, call that function.
}
void add_on_rotate_function(void (*f)(void))
{
push_back(f);
}
}; |
|
|
|
|
|
 |
Martin

|
Posted: Mon Jul 03, 2006 8:22 pm Post subject: (No subject) |
|
|
Because my version is 3 lines of actual code. But in any case, it's a hack until I get around to putting some actual frame limiting in. As for the everything in main, the code is hacked together. The functions are forwarded because the code is messy and I didn't want to have to worry about it
When it gets unmanagable, I'll fix it, I swear.
|
|
|
|
|
 |
wtd
|
Posted: Mon Jul 03, 2006 10:03 pm Post subject: (No subject) |
|
|
You know, if you write the code properly from the start then it doesn't get unmanageable.
|
|
|
|
|
 |
Martin

|
Posted: Mon Jul 03, 2006 11:07 pm Post subject: (No subject) |
|
|
But as soon as I separate the graphics code, I end up having to write a graphics engine, which takes longer (because I have to figure out how to do it first). So until then, this'll suffice.
That said, here's an updated version. The red side has friction! Also, the makefile should work properly with Cygwin now.
Description: |
|
 Download |
Filename: |
particle.rar |
Filesize: |
5.02 KB |
Downloaded: |
236 Time(s) |
|
|
|
|
|
 |
rizzix
|
Posted: Sat Jul 08, 2006 2:06 pm Post subject: (No subject) |
|
|
Here use this (all you osx folks who can't compile his code):
code: | cd particle/particle-osx
xcodebuild |
Description: |
|
 Download |
Filename: |
particle.zip |
Filesize: |
1.87 MB |
Downloaded: |
355 Time(s) |
|
|
|
|
|
 |
Martin

|
Posted: Sun Jul 09, 2006 7:26 pm Post subject: (No subject) |
|
|
And here we have a box that bounces around.
EDIT( Now with textures )
EDIT 2 - Now there are five boxes. Up next ... collision detection between the boxes! Note - sometimes the box is folded in on itself - this is because initially the four points are set to random coordinates. A fix would be to set the points less randomly.
Description: |
|
 Download |
Filename: |
particle.zip |
Filesize: |
179.12 KB |
Downloaded: |
282 Time(s) |
Description: |
|
 Download |
Filename: |
particle.zip |
Filesize: |
179.03 KB |
Downloaded: |
282 Time(s) |
Description: |
|
 Download |
Filename: |
particle.zip |
Filesize: |
193.21 KB |
Downloaded: |
267 Time(s) |
|
|
|
|
|
 |
md

|
Posted: Mon Jul 10, 2006 12:34 pm Post subject: (No subject) |
|
|
Awesome stuff martin... now if only it were slightly less CPU hogging
|
|
|
|
|
 |
|