Author |
Message |
Epoch.
|
Posted: Tue Mar 28, 2006 8:56 am Post subject: Fun with arrays! |
|
|
Hi, I was wondering if there was a way to seperate the digits in an integer into an array. I noticed it can be done with Strings, but I haven't seen anything hinting towards integers.
The reason I am asking is I need to write a program to take in a nine digit number and then do different calculations using the seperate digits. For example, step one requires me to double the second, fourth, sixth and eighth digits and then add them.
Any help is appreciated greatly. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Andy
|
Posted: Tue Mar 28, 2006 12:19 pm Post subject: (No subject) |
|
|
why not convert the integer into a string then break it down? or you can write your own method to extract the last digit add it to your array, then delete the integer by 10 |
|
|
|
|
 |
Dan
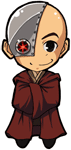
|
Posted: Tue Mar 28, 2006 1:32 pm Post subject: (No subject) |
|
|
Why converter it to an int to begin with? You are inputing it as a string so leave it as a string, use toCharArray and then boom you have an array of chars repersting the ascii of the number. Then you could either leave them as chars and uses an offset or convert them to ints as needed. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
wtd
|
Posted: Tue Mar 28, 2006 1:41 pm Post subject: (No subject) |
|
|
It's easy enough to do with integers if you understand modulus and recursion. |
|
|
|
|
 |
Epoch.
|
Posted: Wed Mar 29, 2006 8:16 am Post subject: (No subject) |
|
|
Andy wrote: why not convert the integer into a string then break it down? or you can write your own method to extract the last digit add it to your array, then delete the integer by 10
It won't let me convert an int value to a String value, it gives me an error reading "inconvertible types".
Hacker Dan wrote: Why converter it to an int to begin with? You are inputing it as a string so leave it as a string, use toCharArray and then boom you have an array of chars repersting the ascii of the number. Then you could either leave them as chars and uses an offset or convert them to ints as needed.
I'm taking it in as an int, not a String - but I see what you're getting at. I'll give it a shot.
wtd wrote: It's easy enough to do with integers if you understand modulus and recursion.
I know how to use modulus, but I have never seen recursion. I've only been programming for about two months in a stupid grade 12 independant course since the normal teacher in on maternity leave. So, I'm not given the material as fast since I go at my own pace without set deadlines. The whole thing is dumb but I need the cred to get into my courses in college.
Again, your help is appreciated. |
|
|
|
|
 |
Andy
|
Posted: Wed Mar 29, 2006 9:15 am Post subject: (No subject) |
|
|
then you're doing something wrong.. post us the code you used to convert from integer to string |
|
|
|
|
 |
HellblazerX

|
Posted: Wed Mar 29, 2006 8:51 pm Post subject: (No subject) |
|
|
Epoch. wrote: It won't let me convert an int value to a String value, it gives me an error reading "inconvertible types".
it sounds like you tried to typecast the int into String, which you cant, because you can't typecast from a primitive to an object. You'll need to use the toString () method.
Epoch. wrote: I'm taking it in as an int, not a String - but I see what you're getting at. I'll give it a shot.
I'm pretty sure all input comes in as String to begin with. How are you taking it in as an int? |
|
|
|
|
 |
Epoch.
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
md

|
Posted: Thu Mar 30, 2006 9:21 am Post subject: (No subject) |
|
|
I think it should be Int(number).toString()
You should also take a look at your code for making sure the number is 9 digits... what happens if I enter 1000000001 so it goes past the first loop, and then 3 inside the second? |
|
|
|
|
 |
HellblazerX

|
Posted: Thu Mar 30, 2006 5:56 pm Post subject: (No subject) |
|
|
really, I thought it was Integer.toString (number). But then again, I'm using a really outdated Java compiler, so what do I know? |
|
|
|
|
 |
md

|
Posted: Thu Mar 30, 2006 6:41 pm Post subject: (No subject) |
|
|
It very well could be Integer(number).toString()... I was guessing from a mediocre memory of Java. The logic error on the other hand is pretty glairing. |
|
|
|
|
 |
Andy
|
Posted: Thu Mar 30, 2006 8:09 pm Post subject: (No subject) |
|
|
both works. hellblazer is simply using an static method of the Integer class, and cornflake is actually creating an Integer object and then converting it to String |
|
|
|
|
 |
wtd
|
Posted: Thu Mar 30, 2006 10:09 pm Post subject: (No subject) |
|
|
Actually Cornflakes is not. He would have to use "new" to do that. |
|
|
|
|
 |
Epoch.
|
Posted: Fri Mar 31, 2006 8:14 am Post subject: (No subject) |
|
|
*sigh* For some reason neither of those two methods work for me... here's the code and the *new* given errors.
code: | strNumber = Integer.toString(number); |
U:\Progs\Chapter 4\SIN.java:37: toString() in java.lang.Object cannot be applied to (int)
strNumber = Integer.toString(number);
code: | strNumber = Integer(number).toString(); |
U:\Progs\Chapter 4\SIN.java:37: cannot find symbol
symbol : method Integer(int)
location: class SIN
strNumber = Integer(number).toString();
I'm going to press on to the next chapter of work and come back to this one, but any further assistance will be greatly appreciated as I cannot find anything about this anywhere
Thanks again for you help.  |
|
|
|
|
 |
wtd
|
Posted: Fri Mar 31, 2006 2:54 pm Post subject: (No subject) |
|
|
Works fine for me.
code: | scala> java.lang.Integer.toString(42)
line5: java.lang.String = 42 |
|
|
|
|
|
 |
|