Problems opening a user defined .txt file
Author |
Message |
AnubisProgrammer
|
Posted: Mon Mar 20, 2006 3:13 pm Post subject: Problems opening a user defined .txt file |
|
|
I'm guessing that it's obviously possible, I'm just not sure of the syntax to get ifstream to open a file that a user has created.
string filename;
cout<<"Please enter the name of your text file (including extension): ";
getline(cin, filename);
ifstream infile;
infile.open("filename");
so, it's asking for the user to type the file name including extension. (not sure if I need to do that or not) then, when it hits infile.open("filename") how do I type ("filename") so it will call the variable "filename" and not try to open a non-existant file? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dan
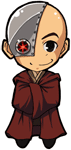
|
Posted: Mon Mar 20, 2006 3:17 pm Post subject: (No subject) |
|
|
By removing the "s in infile.open("filename");
P.S. it just could be that i am realy used to c rather then c++ but your code seems odd to me....are u using the standert librays? |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Tony
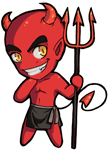
|
Posted: Mon Mar 20, 2006 3:24 pm Post subject: (No subject) |
|
|
as Dan points out, filename is your variable, while "filename" is a string that has a literal value of "filename". |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
md

|
Posted: Mon Mar 20, 2006 4:25 pm Post subject: (No subject) |
|
|
Sicne your using C++ and streams why are you using getline when you could do the much more correct std::cin >> filename; ? |
|
|
|
|
 |
AnubisProgrammer
|
Posted: Mon Mar 20, 2006 4:45 pm Post subject: (No subject) |
|
|
well, first of all, I changed
infile.open("filename")
to
infile.open(filename)
and it's still not working. I get this error:
error C2664: 'void __thiscall std::basic_ifstream<char,struct std::char_traits<char> >::open(const char *,int)' : cannot convert parameter 1 from 'class std::basic_string<ch
ar,struct std::char_traits<char>,class std::allocator<char> >' to 'const char *'
No user-defined-conversion operator available that can perform this conversion, or the operator cannot be called
"filename" is declared as a string at the moment...should it be?
and I don't use the proper std::cin style because my teacher's a moron and never taught us that. He actually didn't even teach us getline, I learned it myself. I'm a grade 12 in a high school programming class, they don't teach us too much. I honestly don't even understand std strings. Except that I include using namespace std; after my libraries. Will std::cin>>filename take an entire string, including spaces? |
|
|
|
|
 |
md

|
Posted: Mon Mar 20, 2006 4:49 pm Post subject: (No subject) |
|
|
No, getline will get the entire line with white spaces cin >> filename will stop at hte first space. Either way works fine though.
As for the string error it's because yes; the function is expecting a character array and you are passing it a class. use the c_str() function to get a character array representing the string. Normally I'd recoment using std::strng simply becuse it keeps you from having to worry about things like memory management when all you want is a short string. Then just use c_str() when you need a character array. |
|
|
|
|
 |
AnubisProgrammer
|
Posted: Mon Mar 20, 2006 4:52 pm Post subject: (No subject) |
|
|
yes....a character array. Like I said, I'm trying to learn a lot of this myself, so could you possibly show an example of how to start a character array or how to use the c_str() function...because I have no idea...sorry. |
|
|
|
|
 |
md

|
Posted: Mon Mar 20, 2006 4:59 pm Post subject: (No subject) |
|
|
Example fo converting to a character string:
c++: |
std::string test = "Blarg";
const char *character_string;
character_string = test.c_str();
|
If you don't know what a character string is, and even if you can't pass varaibles properly then really I dunno that you should be messing around with file IO. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
AnubisProgrammer
|
Posted: Mon Mar 20, 2006 5:19 pm Post subject: (No subject) |
|
|
I agree, without learning more I probably shouldn't be messing with file I/O. However, my class has gotten an assignment that deals with I/O, even though the teacher hasn't taught I/O at all...as you can tell, I don't like my teacher. He's the reason I ask so many questions on here, you guys actually answer them. Anywho, I tried that conversion and hit a little problem. Last question (I hope):
code: | string file;
cin>>file;
const char *filename;
filename=file.c_str();
infile.open(filename); |
with the proper ifstream infile and such, should that work? |
|
|
|
|
 |
md

|
Posted: Mon Mar 20, 2006 5:24 pm Post subject: (No subject) |
|
|
Whoops! I totally forgot about that constant declaration... if you took that out it would work. Teh code I gave was not to show how to use c_str() in your code; just a very general example of how to call it.
You could much more easily use
c++: |
string file;
cin>>file;
infile.open(file.c_str());
|
|
|
|
|
|
 |
|
|