[Tutorial] Strings and String Manipulation
Author |
Message |
rizzix
|
Posted: Fri May 30, 2003 6:00 pm Post subject: [Tutorial] Strings and String Manipulation |
|
|
This tutorial will help you understand the String objects in Java.
In most languages strings are an array of characters. This includes Turing.
But in OOP languages like Java/C++ the strings you get too fool around with are objects. Thus string manipulation is made really easy!
In Java you declare a refrences to a string object like this.
now you may assign a new String object to the my_string reference
like this:
Java: |
my_string = new String("This is a string literal");
|
Nothing new here, that was expected.
But you may also assign a string like this (this is the more common way of doing it):
Java: |
my_string = "This is a string literal";
|
The compiler automatically creates a string object with the contents "This is a string literal".
This means that if you can do this:
Java: |
String my_string;
int length;
my_string = new String("Hello World");
length = my_string. length();
|
you can also do this:
Java: |
int length;
length = "Hello World".length();
|
the concatenation operator for strings is: +
Java: |
my_string = my_string + " Again!";
|
String in Java are immutable objects. So you can't change it's contents.
If you need to you might as well creat an object of type StringBuffer
take a look at this code:
Java: |
String my_string;
my_string = "Hello World";
my_string. replace('H', 'C');
|
This code will work as expected! But didn't we just change the contents of the string?
No. We didn't actually. What really happened is that the replace method created a new string object with the changes and returned the object to the my_string handle.
All classes in Java automaticlly inherit the public String toString() method. This method is called when concatinating other objects with string objects.
Lets extend this method to see it in action!
First create a new class
Java: |
class TestObject {
String the_string;
public void setString (String str ) {
the_string = str;
}
public String getString () {
return the_string;
}
public String toString () {
return "This is an object of type: TestObject";
}
public static void main (String[] args ) {
TestObject obj;
obj = new TestObject ();
obj. setString("Hello World");
System. out. println(obj. getString() + " Again! " + obj );
}
}
|
compile it and run! It should work.
Here we have used all concepts we talked about. Concatenation, literals, and the use of the toString() method in objects.
Note: since all objects inherit the toString method you can try out concatenating an object with a null string i.e: "" and get the default string returned by that object.
You need to concatenate it since it is the concatenation operator that calls the method of that object.
take a look at this code:
Java: |
String my_string1, my_string2;
my_string1 = "abc";
my_string2 = "abc";
if (my_string1 == my_string2 ) {
System. out. println("Match");
} else {
System. out. println("Do not match");
}
|
The result will be Do not match. Why?
Remember that my_string1 and my_string2 are only refrences to objects of type String. Since the refrences do not point to the same object the result is false.
To get the expected result you need to use the equals method of the String object.
Java: |
if (my_string1.equals(my_string2)) {
|
To learn more on what you can do with Strings in Java take a look at the Java API documentation. You will find it under the java.lang package, which is the package that is automatically imported by all class files. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
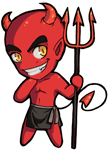
|
Posted: Fri May 30, 2003 8:04 pm Post subject: (No subject) |
|
|
string is an object?! Awesome 8) I'm looking farward to learning Java...
+25Bits
One question though... You mentioned that you can't change the content of the string... Could you write an example of how the user would enter their name and store that in a variable? How would I go about having buffers (variables to store temporary information before some process uses them)? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
rizzix
|
Posted: Sat May 31, 2003 8:02 am Post subject: (No subject) |
|
|
You can do this in Java:
Java: |
String my_string;
my_string = "abc";
System. out. println(my_string );
my_string = "def";
System. out. println(my_string );
|
It works as expected. But note each time you are assigning a new string object to the my_string handle!
Usually you may never need to use StringBuffers. Unless you want to do some intensive manipulation on a series of characters. |
|
|
|
|
 |
|
|